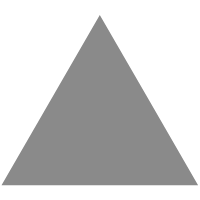
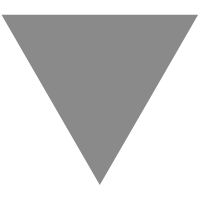
Framework for making games in JavaScript
source link: https://www.tuicool.com/articles/RB3mi2M
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
PtrJS
A framework for JavaScript canvas
Documentation
Variables
-
mobile
- variable that is
true
if the code is opened from a mobile device orfalse
if it is not; useful if you have special control buttons for mobile devices - width and height - contain the width and height of the canvas element
- innerWidth and innerHeight - contain the inner width and inner height of the browser window
- mouseX and mouseY - contain the current coordinates of the mouse on the screen
- PI - Pi constant
- TWO_PI - two times Pi
- HALF_PI - half Pi
- E - Euler's constant
- SQRT2 - square root of 2
- SQRT1_2 - square root of 1/2
- LN2 - natural logarithm of 2
- LN10 - natural logarithm of 10
- LOG2E - base 2 logarithm of e
- LOG10E - base 10 logarithm of e
- frameCount - contains the number of frames that have passed since the loop started running
- frameRate - contains the current number of frames per second
-
mousePressed
- equals to
true
if the mouse is currently being pressed orfalse
if it isn't
Functions
setup() update() preload() noCanvas() framerate(newFramerate) map(num, a, b, c, d) random(num1, num2) randInt(num1, num2) randomizeColor(r, g, b) floor(num) ceil(num) round(num) pow(num, pow) sqrt(num) sqr(num) abs(num) sin(angle) cos(angle) asin(num) acos(num) tan(angle) atan(num) exp(num) logh(num) min(nums) max(nums) write(text) log(text) table(array) error(text) warn(text) setText(element, text) keyDown() keyUp() touchStart() touchMove() touchEnd() mouseDown() mouseMove() mouseUp() swipe(dir) createVector(x, y) randomVector(magnitude) createPoint(x, y) randomPoint() createCanvas(widht, height) Vector.fromAngle(angle) color(red, green, blue, alpha) isInArray(array, element) ramoveFromArray(array, element) createMatrix(columns, rows) distance(x1, y1, x2, y2) swap(array, index1, index2) sort(array) toRadians(degrees) toDegrees(radians) constrain(num, min, max) lerp(value1, value2, step) joinArray(array, spacing) removeChars(text, characters) removeCharAt(text, index) replaceCharAt(text, index, replacement)
Classes
Canvas
Class Canvas is the main class of the framework. When an instance is created, it creates a canvas element and appends it to the document body. An instance can be created by calling the createCanvas()
function. Example: let canvas = createCanvas(400, 400);
Attributes
- width - contains the width of the canvas; it can also be accessed through the width global variable
- height - contains the height of the canvas; it can also be accessed through the height global variable
- maxWidth - maximum canvas width; by default set to 99.000, from -33.000 to + 66.000
- maxHeight - maximum canvas height; by default set to 99.000, from -33.000 to + 66.000
- canvas - points to the actual canvas element that is displayed in the document
- ctx - contains the context of the canvas element
Methods
setMaxSize(newMaxWidth, newMaxHeight) setSize(newWidth, newHeight) fullScreen() clear() background(r, g, b) fill(r, g, b, a) noFill() stroke(r, g, b, a) noStroke() lineWidth(width) line(x1, y1, x2, y2) lineFromVector(vector) lineFromAngle(x, y, angle, length) rect(x, y, width, height) rectMode(mode) point(x, y) circle(x, y, radius) ellipse(x, y, width, height, rotation) arc(x, y, radius, startAngle, endAngle) beginShape(x, y) vertex(x, y) closeShape() text(text, x, y, fontSize, fontName) textAlign(alignment) translate(x, y) rotate(angle) scale(widthScale, heightScale) save() restore() screenshot() playPause() pause() play() drawImage(image, sx, sy, swidth, sheight, x, y, width, height)
- image - an image object
- sx (optional) - x value from which the cropping of the image starts
- sy (optional) - y value from which the cropping of the image starts
- swidth (optional) - width of the crop
- sheight (optional) - height of the crop
- x - x coordinate of the canvas from which the image starts drawing
- y - y coordinate of the canvas from which the image starts drawing
- width (optional) - width of the image on the canvas; if left blank, it will be displayed in its full size
- height (optional) - height of the image on the canvas; if left blank, it will be displayed in its full size
Image
Takes a path to the image as an argument.
Attributes
- path - contains the path to the image
- filename - contains the name of the image file
- image - contains the image element
Point
A point on the canvas
Attribures
- x - the x coordinate of the point
- y - the y coordinate of the point
Methods
distance(point2) isOffScreen()
Vector
A 2D Vector object
Attributes
- x - the x coordinate
- y - the y coordinate
- previousX - contains the previous x coordinate of the vector in case it has changed
- previousY - contains the previous y coordinate of the vector in case it has changed
Methods
set(newX, newY) add(vector2) subtract(vector2) multiply(vector2) divide(vector2) angle() rotate(angle) magintude() magnitudeSqr() setMagnitude(newMagnitude) copy() normalize() distance(vector2) isOffScreen() lerp(vector2, step) constrain(minX, maxX, minY, maxY)
Color
A color class
Attributes
- red - red value of the color
- green - green value of the color
- blue - blue value of the color
- alpha - alpha value of the color
Methods
randomize(randomizeAlpha) color()
You can check out the example folder for some examples of code usage
Creating a new PtrJS project
To create a new PtrJS project you can clone one of the templates from the examples folder. The offline template is self-contained and all the script files come with it, whereas the other template uses the online version of the file and uses the latest version by default. You can change this by changing the url in the script tag in the index.html file.
You can also implement the files into your existing project by dowloading the script file or adding it through the CDN. The URL for the CDN is as follows:
https://cdn.petarmijailovic.com/ptrjs/version/ptr.js or https://cdn.petarmijailovic.com/ptrjs/version/ptr.min.js
To use the latest version, add the following line of code into your head tag
<script src="https://cdn.petarmijailovic.com/ptrjs/latest/ptr.min.js"></script>
The lowest available version is 2.0
The latest version is 2.0.5
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK