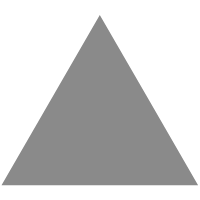
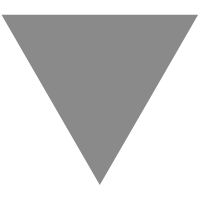
Usb.Net 3.0 Beta
source link: https://www.tuicool.com/articles/ueQZZ3V
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Usb.Net is a cross platform USB library for .NET, UWP, and Android. Adapters can be added for platforms such as Linux, or OSX. It puts a wrapper the OS specific APIs and allows you to write USB code that runs on any of the platforms. Device.Net is a framework for communicating with connected devices such as USB, and Hid devices. Version 3.0 Beta of these libraries have been released, and I’d like to hear about feedback.
Major Changes
This is the Github release notes page that lists the issues the were fixed. The main problems with previous versions of Usb.Net were that it was trying to use interrupt pipes for transfer, and the buffer sizes could not be specified. The library has been overhauled so that any if the existing USB interfaces or pipes can be selected, and the buffer sizes can be specified in constructors. This should cover any scenario for connecting to a device.
Using the Library
The best place to get started is with the GitHub wiki . This page is a little out of date and is missing some information about how to set the USB interface, and Endpoint. This documentation is being worked on, and will be added to the wiki soon. Meantime, if you have trouble modifying the sample for your device, please get in contact through the issues page, and I will be happy to help.
using Device.Net; using System; using System.Threading.Tasks; namespace Usb.Net.Windows { public class UsbExample : IDisposable { private WindowsUsbDevice _WindowsUsbDevice; public UsbExample(string deviceId) { _WindowsUsbDevice = new WindowsUsbDevice(deviceId, new DebugLogger(), new DebugTracer(), 64, 64); } public async Task InitializeAsync() { await _WindowsUsbDevice.InitializeAsync(); //This is not necessary for most cases, but allows you to select an interface and endpoint _WindowsUsbDevice.UsbDeviceHandler.ReadUsbInterface = _WindowsUsbDevice.UsbDeviceHandler.UsbInterfaces[1]; _WindowsUsbDevice.UsbDeviceHandler.ReadUsbInterface.ReadEndpoint = _WindowsUsbDevice.UsbDeviceHandler.ReadUsbInterface.UsbInterfaceEndpoints[1]; _WindowsUsbDevice.UsbDeviceHandler.WriteUsbInterface = _WindowsUsbDevice.UsbDeviceHandler.UsbInterfaces[2]; _WindowsUsbDevice.UsbDeviceHandler.WriteUsbInterface.ReadEndpoint = _WindowsUsbDevice.UsbDeviceHandler.WriteUsbInterface.UsbInterfaceEndpoints[1]; } public void Dispose() { _WindowsUsbDevice.Dispose(); } } }
The above is a windows sample but the same code will work on any of the platforms.
Grab the NuGet. You will need to add Device.Net manually.
Usb.Net
Hid.Net
Device.Net
Make sure you include pre-release.
Why use Device.Net and Usb.Net?
There are plenty of C# libraries around for USB. The problem is that they are all different across platforms. Most of the code cannot be reused. Usb.Net maintains uniform code across platforms, but also coupled with Hid.Net allows you to communicate with Hid devices in exactly the same way. Bluetooth support is also on its way. The Device.Net framework is much more than a simple library. So, please check it out and help me iron out the kinks.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK