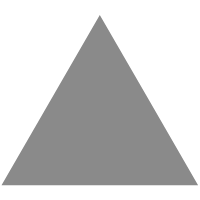
37
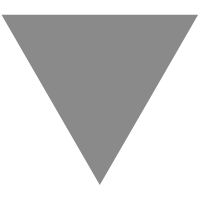
React hook usePosition() for fetching and following a browser geolocation
source link: https://www.tuicool.com/articles/nYzeuua
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React hook for following a browser geolocation
React hook usePosition()
allows you to fetch client's browser geolocation and/or subscribe to all further geolocation changes.
▶︎ Storybook demo of usePosition()
hook.
Installation
Using yarn
:
yarn add use-position
Using npm
:
npm i use-position --save
Usage
Import the hook:
import { usePosition } from 'use-position';
Fetching client location
const { latitude, longitude, timestamp, accuracy, error } = usePosition();
Following client location
In this case if browser detects geolocation change the latitude
, longitude
and timestamp
values will be updated.
const { latitude, longitude, timestamp, accuracy, error } = usePosition(true);
Following client location with highest accuracy
The second parameter of usePosition()
hook is position options .
const { latitude, longitude, timestamp, accuracy, error } = usePosition(true, {enableHighAccuracy: true});
Full example
import React from 'react'; import { usePosition } from '../src/usePosition'; export const Demo = () => { const { latitude, longitude, timestamp, accuracy, error } = usePosition(true); return ( <code> latitude: {latitude}<br/> longitude: {longitude}<br/> timestamp: {timestamp}<br/> accuracy: {accuracy && `${accuracy}m`}<br/> error: {error} </code> ); };
Specification
usePosition()
input
-
watch: boolean
- set it totrue
to follow the location. -
settings: object
- position options-
settings.enableHighAccuracy
- indicates the application would like to receive the most accurate results (defaultfalse
), -
settings.timeout
- maximum length of time (in milliseconds) the device is allowed to take in order to return a position (defaultInfinity
), -
settings.maximumAge
- the maximum age in milliseconds of a possible cached position that is acceptable to return (default0
).
-
usePosition()
output
-
latitude: number
- latitude (i.e.52.3172414
), -
longitude: number
- longitude (i.e.4.8717809
), -
timestamp: number
- timestamp when location was detected (i.e.1561815013194
), -
accuracy: number
- location accuracy in meters (i.e.24
), -
error: string
- error message ornull
(i.e.User denied Geolocation
)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK