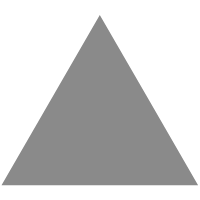
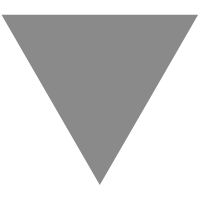
Home | React Hook Form - Simple React form validation
source link: https://react-hook-form.com/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React Hook Form
Performant, flexible and extensible forms with easy-to-use validation.
Intuitive, feature-complete API providing a seamless experience to developers when building forms.
HTML standard
Leverage existing HTML markup and validate your forms with our constraint-based validation API.
Super Light
Package size matters. React Hook Form is a tiny library without any dependencies.
Performance
Minimizes the number of re-renders and faster mounting, striving to provide the best user experience.
Adoptable
Since form state is inherently local, it can be easily adopted without other dependencies.
Award
Winner of 2020 GitNation React OS Award for Productivity Booster.
Less code. More performant
Reducing the amount of code you need to write, and removing unnecessary re-renders are some of the primary goals of React Hook Form. Now dive in and explore with the following example:
React Hook Form
import React from "react";
import { useForm } from "react-hook-form";
const Example = () => {
const { handleSubmit, register, errors } = useForm();
const onSubmit = values => console.log(values);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input
name="email"
ref={register({
required: "Required",
pattern: {
value: /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i,
message: "invalid email address"
}
})}
/>
{errors.email && errors.email.message}
<input
name="username"
ref={register({
validate: value => value !== "admin" || "Nice try!"
})}
/>
{errors.username && errors.username.message}
<button type="submit">Submit</button>
</form>
);
};
Isolate Re-renders
You have the ability to isolate components re-renders which leads to better performance on your page or app. The following example demonstrates this behaviour.
Note: Type in the input box to see the render behaviour.
React Hook Form
Controlled Form
Change Subscriptions
Performance is an important aspect of user experience in terms of building forms. You will have the ability to subscribe to individual input changes without re-rendering the entire form.
Reduce Rendering
Do you ever wonder how many component re-renders have been triggered by the user? React Hook Form embraces uncontrolled form validation to reduce any unnecessary performance penalty.
Total re-renders: 30+
Total re-renders: 3
Total re-renders: 30+
Faster Mounting
The following screenshots demonstrate how much faster component mounting is with React Hook Form. Mounting and rendering the Library Code Comparison is ~13% faster than Formik and ~25% faster than Redux Form.⚠ Note: a 6x CPU slowdown was simulated with Chrome Dev Tools for the sake of the benchmark.
React Hook Form
- No. of mount(s): 1
- No. of committing change(s): 1
- Total time: 1800ms

Formik
- No. of mount(s): 6
- No. of committing change(s): 1
- Total time: 2070ms

Redux Form
- No. of mount(s): 17
- No. of committing change(s): 2
- Total time: 2380ms

⚠ Want to see more intense performance tests? Check out the result of 1000 fields within a form here.
Live Demo
The following form demonstrates form validation in action. Each column represents what has been captured in the custom hook. You can also change fields in the form by clicking the EDIT button.
Example
Watch
ⓘ Change inputs value to update watched values
{ "First name": "", "Last name": "", "Email": "", "Mobile number": "", "Title": "", "Developer": "" }
Errors
ⓘ Validation errors will appear here
Touched
ⓘ Touched fields will display here
[]
Ready to get started?
Form handling doesn't have to be painful. React Hook Form will help you write less code while achieving better performance.
Design and Build by @Bill Luo ✚ React Simple Animate ✚ React Simple Img ✚ Little State Machine
Please support us by leaving a ★ @github | Feedback
Hosted on ▲ Vercel Now
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK