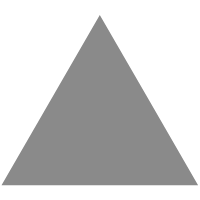
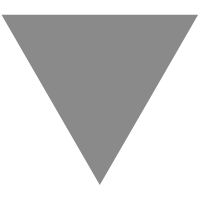
JDK源码分析-ReentrantLock
source link: https://www.tuicool.com/articles/vyeqqyy
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
概述
在 JDK 1.5 以前,锁的实现只能用 synchronized 关键字;1.5 开始提供了 ReentrantLock,它是 API 层面的锁 。先看下 ReentrantLock 的类签名以及如何使用:
public class ReentrantLock implements Lock, java.io.Serializable {}
典型用法:
public void m() {
lock.lock(); // block until condition holds
try {
// ... method body
} finally {
lock.unlock()
}
}
该用法和使用 synchronized 关键字效果是一样的。 既然有了 synchronized,为什么又会有 Lock 呢?相比于 synchronized,其实 ReentrantLock 的出现并不重复,它增加了不少功能,下面先简单介绍几个概念。
公平锁&非公平锁 : 所谓锁是否公平,简单理解就是一系列线程获取到锁的顺序是否遵循「先来后到」。即,如果先申请锁的线程先获取到锁,就是公平锁;否则就是非公平锁。ReentrantLock 的默认实现和 synchronized 都是非公平锁。
可重入锁 :锁是否可重入, 就是一个线程是否可以多次获取同一个锁,若是,就是可重入锁。ReentrantLock 和 synchronized 都是可重入锁。
代码分析
构造器
ReentrantLock 有两个构造器,分别如下:
private final Sync sync;
// 构造一个 ReentrantLock 实例(非公平锁)
public ReentrantLock() {
sync = new NonfairSync();
}
// 构造一个 ReentrantLock 实例(指定是否公平)
public ReentrantLock(boolean fair) {
sync = fair ? new FairSync() : new NonfairSync();
}
可以看到,两个构造器都是初始化一个 Sync 类型的成员变量。而且,当 boolean 值 fair 为 true 时,初始化的 sync 为 FairSync,为 false 时初始化为 NonFairSync,二者分别表示「公平锁」和「非公平锁」。可以看到无参构造默认是非公平锁。
常用方法
ReentrantLock 常用的方法就是 Lock 接口定义的几个方法,如下:
// 获取锁(阻塞式)
public void lock() {
sync.lock();
}
// 获取锁(响应中断)
public void lockInterruptibly() throws InterruptedException {
sync.acquireInterruptibly(1);
}
// 尝试获取锁
public boolean tryLock() {
return sync.nonfairTryAcquire(1);
}
// 尝试获取锁(有超时等待)
public boolean tryLock(long timeout, TimeUnit unit)
throws InterruptedException {
return sync.tryAcquireNanos(1, unit.toNanos(timeout));
}
// 释放锁
public void unlock() {
sync.release(1);
}
可以看到,这几个方法内部都是通过调用 Sync 类(或其子类)的方法来实现, 因此先从 Sync 类入手分析,代码如下(部分省略) :
// 抽象类,继承了 AQS
abstract static class Sync extends AbstractQueuedSynchronizer {
// 获取锁的方法,由子类实现
abstract void lock();
// 非公平锁的 tryLock 方法实现
final boolean nonfairTryAcquire(int acquires) {
final Thread current = Thread.currentThread();
// 获取 AQS 的 state 变量
int c = getState();
// 若为 0,表示当前没有被其他线程占用
if (c == 0) {
// CAS 修改 state,若修改成功,表示成功获取资源
if (compareAndSetState(0, acquires)) {
// 将当前线程设置为 owner,到这里表示当前线程成功获取资源
setExclusiveOwnerThread(current);
return true;
}
}
// state 不为 0,且 owner 为当前线程
// 表示当前线程已经获取到了资源,这里表示“重入”
else if (current == getExclusiveOwnerThread()) {
int nextc = c + acquires;
if (nextc < 0) // overflow
throw new Error("Maximum lock count exceeded");
// 修改 state 值(因为当前线程已经获取资源,不存在竞争,因此无需 CAS 操作)
setState(nextc);
return true;
}
return false;
}
// 释放锁操作(对 state 做减法)
protected final boolean tryRelease(int releases) {
int c = getState() - releases;
if (Thread.currentThread() != getExclusiveOwnerThread())
throw new IllegalMonitorStateException();
boolean free = false;
if (c == 0) {
free = true;
// 成功释放后将 owner 设为空
setExclusiveOwnerThread(null);
}
// 修改 state 的值
// PS: 因为可能存在“重入”,因此一次释放操作后当前线程仍有可能占用资源,
// 所以不会直接把 state 设为 0
setState(c);
return free;
}
// 其他方法...
final boolean isLocked() {
return getState() != 0;
}
}
Sync 类继承自 AQS,其中 nonfairTryAcquire 方法是非公平锁 tryAcquire 方法的实现。
从上面代码可以看出,锁的获取和释放是通过修改 AQS 的 state 变量来实现的。lock 方法可以看做对 state 执行“加法”操作,而 unlock 可以看做对 state 执行“减法”操作,当 state 为 0 时,表示当前没有线程占用资源。
公平锁&非公平锁
(1)非公平锁 NonFairSync :
static final class NonfairSync extends Sync {
final void lock() {
// CAS 尝试将 state 值修改为 1
if (compareAndSetState(0, 1))
// 若修改成功,则将当前线程设为 owner,表示成功获取锁
setExclusiveOwnerThread(Thread.currentThread());
// 若获取失败,则执行 AQS 的 acquire 方法(独占模式获取资源)
else
acquire(1);
}
protected final boolean tryAcquire(int acquires) {
return nonfairTryAcquire(acquires);
}
}
可以看到,非公平锁的 lock 操作为:先尝试以 CAS 方式修改 state 的值,若修改成功,则表示成功获取到锁,将 owner 设为当前线程;否则就执行 AQS 中的 acquire 方法,具体可参考前文「 JDK源码分析-AbstractQueuedSynchronizer(2) 」,这里不再赘述。
(2)公平锁 FairSync:
static final class FairSync extends Sync {
final void lock() {
acquire(1);
}
// 公平锁的 tryAcquire 实现
protected final boolean tryAcquire(int acquires) {
final Thread current = Thread.currentThread();
int c = getState();
// state 为 0,表示资源未被占用
if (c == 0) {
// 若队列中有其他线程在排队等待,则返回 false,表示获取失败;
// 否则,再尝试去修改 state 的值
// PS: 这里是公平锁与非公平锁的区别所在
if (!hasQueuedPredecessors() &&
compareAndSetState(0, acquires)) {
setExclusiveOwnerThread(current);
return true;
}
}
// 若当前线程已占用了锁,则“重入”
else if (current == getExclusiveOwnerThread()) {
int nextc = c + acquires;
if (nextc < 0)
throw new Error("Maximum lock count exceeded");
setState(nextc);
return true;
}
return false;
}
}
可以看到,与非公平锁相比,公平锁的不同之处在于增加了判断条件 hasQueuedPredecessors,即首先判断主队列 中是否有其他线程在等待,当没有其他线程在排队时再去获取,否则获取失败。
hasQueuedPredecessors 在 AQS 中实现如下:
/**
* Queries whether any threads have been waiting to acquire longer
* than the current thread.
*/
public final boolean hasQueuedPredecessors() {
// The correctness of this depends on head being initialized
// before tail and on head.next being accurate if the current
// thread is first in queue.
Node t = tail; // Read fields in reverse initialization order
Node h = head;
Node s;
return h != t &&
((s = h.next) == null || s.thread != Thread.currentThread());
}
小结
synchronized 与 ReentrantLock 比较:
相同点: 二者都是互斥锁,可重入,默认都是非公平锁。
不同点: synchronized 是语法层面实现,自动获取锁和释放锁;ReentrantLock 是 API 层面实现,手动获取锁和释放锁。
ReentrantLock 相比 synchronized 的优势:
1. 可响应中断;
2. 获取锁可设置超时;
3. 可实现公平锁;
4. 可绑定多个条件(Condition)。
JDK 1.6 以后,synchronized 与 ReentrantLock 性能基本持平,JVM 未来的性能优化也会更偏向于原生的 synchronized。因此,如何选择还要根据实际需求, 性能不再是不选择 synchronized 的原因了。
相关阅读:
JDK源码分析-AbstractQueuedSynchronizer(2)
Stay hungry, stay foolish.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK