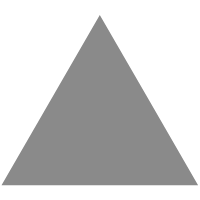
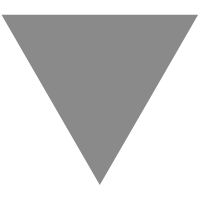
使用vue-i18n实现多语言切换效果
source link: https://www.tuicool.com/articles/Uv2y2yi
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
安装vue-i18n
我们使用npm安装vue-i18n。
npm install vue vue-i18n --save
引入vue-i18n
首先在 main.js 中引入 vue-i18n。
import Vue from 'vue' import App from './App' import VueI18n from 'vue-i18n' Vue.use(VueI18n) // 通过插件的形式挂载
接着创建带有选项的 VueI18n 实例。
const i18n = new VueI18n({ locale: localStorage.getItem('locale') || 'zh-CN', //this.$i18n.locale // 通过切换locale的值来实现语言切换 messages: { 'zh-CN': require('./lang/zh'), // 中文语言包 'en-US': require('./lang/en') // 英文语言包 } })
注意实例中加载了中英文两个语言包。分别准备两个语言包, 使用require引入到main.js中:
中文语言包:zh.js
export const m = { welcome: '欢迎来到北京', today: '今天是', week: { sun: '星期日', mon: '星期一', tues: '星期二', wed: '星期三', thur: '星期四', fri: '星期五', sat: '星期六' } }
英文语言包: en.js
export const m = { welcome: 'Welcome to Beijing.', today: 'Today is ', week: { sun: 'Sunday', mon: 'Monday', tues: 'Tuesday', wed: 'Wednesday', thur: 'Thursday', fri: 'Friday', sat: 'Saturday' } }
然后把 i18n 挂载到 vue 根实例上:
new Vue({ el: '#app', i18n, components: { App }, template: '<App/>' })
使用vue-i18n
我们先建立模板:
<button @click="changeLang">切换语言</button> <h1>{{$t('m.welcome')}}</h1> <h3>{{$t('m.today')}}{{weekname}}</h3>
注意用法,在组件的模板template中,调用 $t()
方法, {{$t('m.welcome')}}
表示使用welcome的语言。如果是在组件的script中,调用 this.$i18n.t()
方法获取语言,下文会讲到。
我们想通过点击“切换语言”按钮,来对模板中的文字内容进行相应的语言切换。
那我们就在方法 changeLang()
中这样写:
changeLang() { this.lang = localStorage.getItem('locale') || 'zh-CN'; if ( this.lang === 'zh-CN' ) { this.lang = 'en-US'; this.$i18n.locale = this.lang; } else { this.lang = 'zh-CN'; this.$i18n.locale = this.lang; } localStorage.setItem('locale', this.lang); let week = this.getWeek(); this.weekname = week; },
我们先在本地存储中获取 locale
的值,如果不存在则默认为 zh-CN
。然后在判断当前语言是中文还是英文,如果是中文则切换成英文,反之亦然。通过 this.$i18n.locale
实现语言的切换。
我们希望浏览器保存每次切换后的语言,用户刷新页面的时候会自动识别语言。因此我们使用 localStorage
本地存储来保存每次设置后的语言,当然你也可以使用 coockie
实现。
有时候我们要在js部分处理多语言,例如以下 getWeek()
部分实现了当前是星期几的代码,仅供参考。
getWeek() { let week = new Date().getDay(); let day = 'm.week.sun'; switch (week) { case 0: day = 'm.week.sun'; break; case 1: day = 'm.week.mon'; break; case 2: day = 'm.week.tues'; break; case 3: day = 'm.week.wed'; break; case 4: day = 'm.week.thur'; break; case 5: day = 'm.week.fri'; break; case 6: day = 'm.week.sat'; break; } return this.$i18n.t(day); }
以上就是使用vue-i18n实现中英文语言切换效果的详细内容。有关vue-i18n的更多用法可以参考项目地址: http://kazupon.github.io/vue-i18n/
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK