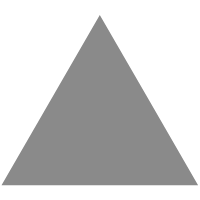
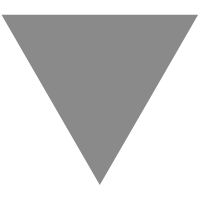
SQLAlchemy的Mapping与Declarative
source link: https://www.tuicool.com/articles/6NVFvez
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
前面介绍过vSQLAlchemy中的 Engine 和 Connection,这两个对象用在row SQL (原生的sql语句)上操作,而 ORM(Object Relational Mapper)则是一种用面向对象的思维来操作表数据的技术。所谓ORM 就是Python 对象到数据表的一种映射关系。
以前 SQLAlchemy 是怎么把Python对象和数据库中表里面的每条记录进行映射的呢?通过一个 mapping
函数
先来看个例子:
from sqlalchemy import Table, MetaData, Column, Integer, String, from sqlalchemy.orm import mapper # 数据库的元数据,你可以认为它是一个容器,装载了所有的表结构 metadata = MetaData() # 数据库中的news_article表 article = Table("news_article", metadata, Column("id", Integer, primary_key=True), Column("title", String) ) # 这是一个普通的Article类 class Article: def __init__(self, title): self.title = title # 通过mapper函数进行映射关联 mapper(Article, article)
关联后怎么使用呢?看例子:
from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=engine) session = Session() # 通过Artcile类来查询id==4554的记录,这完全是用面向对象的方式执行sql了 # 返回结果就是Article的实例对象 result = session.query(Article).filter(Article.id==4554).first() print(result.id) # 4554 print(result.title) # xxxxxxxxx
mapper 函数进行映射后,通过query查询返回的结果,会自动将返回结果构造成一个Article对象,并拥有了id 属性,这就是ORM的魔力所在。
而新的ORM映射不需要手动通过mapping函数来关联table与类之间的关系,可以直接通过声明(Declarative )系统(我不知道这样翻译对不对)来定义一个类,这个类会直接映射到数据库的表, declarative
把 Table、mapper、还有类这三者放在一块进行声明,从而实现了ORM的映射。来看例子:
from sqlalchemy.ext.declarative import declarative_base Base = declarative_base() class Article(Base): __tablename__ = 'news_article' id = Column(Integer, primary_key=True) title = Column(String(50))
是不是简单很多了,没有了Table的定义,没有mapper函数,只有一个类的定义,这个类必须继承基类 Base,Base 就是我们的声明系统,这样就完成了Table与类之间的映射关系,而背后的操作都是通过一个 declarative_base
工厂方法构造的声明系统完成的。
我们把 Article
又称之为映射类,这个类持有 Table 和 mapper 函数的引用。
>>> print(Article.__table__) news_article >>>print(Article.__mapper__) Mapper|Article|news_article # 前面将的metadata 可以通过 Base 获取 >>>print(Base.metadata) MetaData(bind=None)
MetaData 有什么用的?可以通过它来创建表或者删除表。
https://docs.sqlalchemy.org/en/13/orm/mapping_styles.html#classical-mapping
有问题可以扫描二维码和我交流
关注公众号「Python之禅」,回复「1024」免费获取Python资源

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK