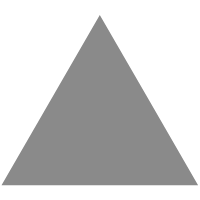
63
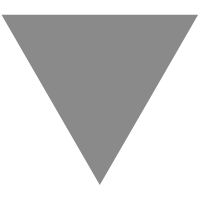
GitHub - Taymindis/wfqueue: c/c++ wait-free queue, easy built cross platform.
source link: https://github.com/Taymindis/wfqueue
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
wfqueue.h 
c/c++ FIFO wait-free queue, easy built cross platform(no extra dependencies needed)
Guarantee thread safety memory management, and it's all in one header only, as fast as never wait.
All Platform tests
support MPMC, MPSC, MCSP
API
// Fixed size of queue, it should at least 2 times more than the number of concurrency level. wfqueue_t *wfq_create(size_t fixed_size); int wfq_enq(wfqueue_t *q, void* val); void* wfq_deq(wfqueue_t *q); void wfq_destroy(wfqueue_t *q); size_t wfq_size(wfqueue_t *q); size_t wfq_capacity(wfqueue_t *q);
Example
if c++
#include "wfqueue.h" // Fixed size of queue, it should at least 2 times more than the number of concurrency level. wfqueue_t *q = wfq_create(fixed_sz); // wrap in to thread wfq_enq(q, new ClassVal); // or malloc if c programming, return 1 if success enqueue // wrap in to thread ClassVal *s = (intV*)wfq_deq(q); // return NULL/nullptr if no val consuming if(s) { s->do_op(); delete s; } wfq_destroy(q);
if c
#include "wfqueue.h" // Fixed size of queue, it should at least 2 times more than the number of concurrency level. wfqueue_t *q = wfq_create(fixed_sz); // wrap in to thread wfq_enq(q, malloc(sizeof(ClassVal)); // or malloc if c programming, return 1 if success enqueue // wrap in to thread ClassVal *s = (intV*)wfq_deq(q); // return NULL/nullptr if no val consuming if(s) { s->do_op(); free(s); } wfq_destroy(q);
Build
include header file in your project
Wrap this in your class
example
class MyQueue { wfqueue_t *q; public: MyQueue ( size_t sz ) { q = wfq_create(sz); } inline int enq(Xclass *val) { return wfq_enq(q, val); } inline Xclass *deq() { return (Xclass*)wfq_deq(q); } inline size_t getSize() { return wfq_size(q); } ~MyQueue() { wfq_destroy(q);; } }
You may also like lock free queue FIFO
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK