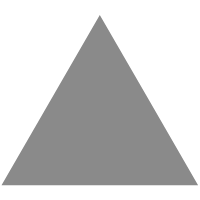
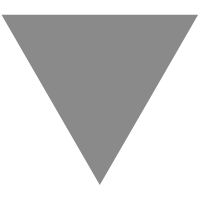
GitHub - system-ui/theme-ui: Build consistent, themeable React UIs based on desi...
source link: https://github.com/system-ui/theme-ui
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
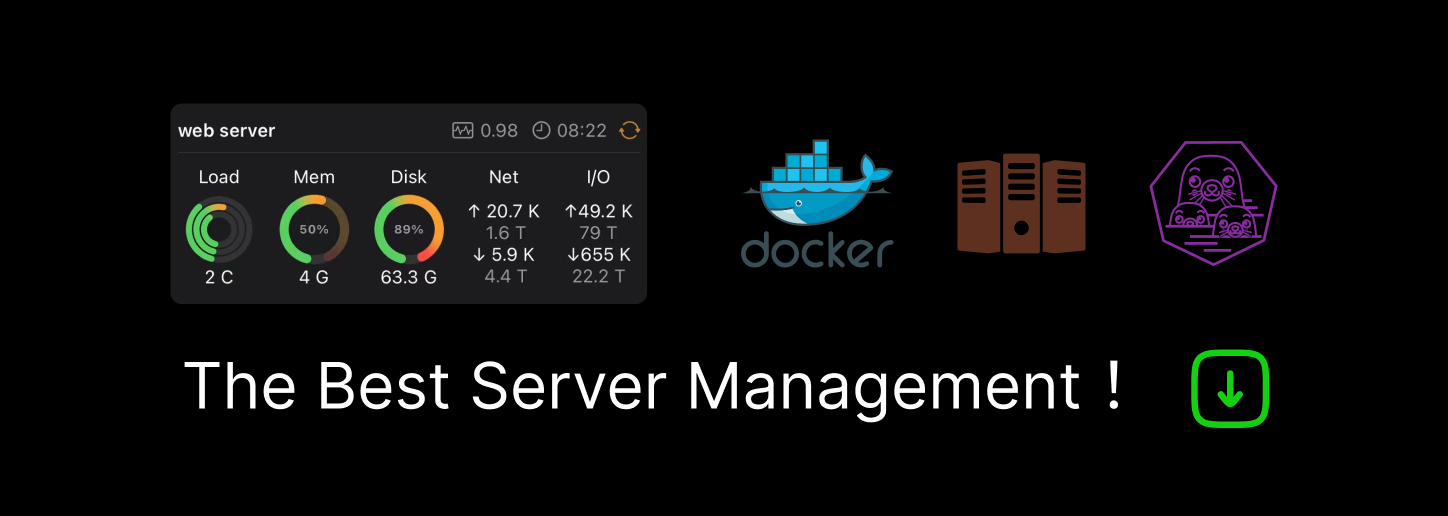
README.md
Theme UI
Build consistent, themeable React UIs based on design system constraints and design tokens
- Style your application consistently with a global theme object and customizable design tokens
- Style MDX content with themes
- Follows the Theme Specification for interoperability with other libraries
- Style elements directly with the
css
prop and @styled-system/css - Works with or without custom styled components and Styled System
- First-class support for Typography.js themes
- First-class support for dark mode and other color modes
- Use contextual theming for nested stylistic changes
- Primitive page layout components
- Keep styles isolated with Emotion
Getting Started
npm i theme-ui
Theme UI also requires the following dependencies
npm i @emotion/core @emotion/styled @mdx-js/react
Wrap your application with the ThemeProvider
component and pass in a custom theme
object.
// basic usage import React from 'react' import { ThemeProvider } from 'theme-ui' import theme from './theme' export default props => <ThemeProvider theme={theme}> {props.children} </ThemeProvider>
The theme
object should follow the System UI Theme Specification,
which lets you define custom color palettes, typographic scales, fonts, and more.
// example theme.js export default { fonts: { body: 'system-ui, sans-serif', heading: '"Avenir Next", sans-serif', monospace: 'Menlo, monospace', }, colors: { text: '#000', background: '#fff', primary: '#33e', }, }
css
prop
Use the css
prop throughout your application, along with the css
utility to pick up values from the theme.
Using the css
utility means that
color and other values can reference values defined in theme
object.
import React from 'react' import { css } from 'theme-ui' export default () => <div css={css({ fontWeight: 'bold', fontSize: 4, // picks up value from `theme.fontSizes[4]` color: 'primary', // picks up value from `theme.colors.primary` })}> Hello </div>
Read more about the css
utility in the @styled-system/css docs.
Responsive styles
The css
utility also supports using arrays as values to change properties responsively with a mobile-first approach.
import React from 'react' import { css } from 'theme-ui' export default props => <div css={css({ // applies width 100% to all viewport widths, // width 50% above the first breakpoint, // and 25% above the next breakpoint width: [ '100%', '50%', '25%' ], })} />
jsx
pragma
To use values from the theme
object without importing the css
utility, use the custom jsx
pragma, which will automatically convert Theme UI css
prop values.
/** @jsx jsx */ import { jsx } from 'theme-ui' export default props => <div css={{ fontSize: 4, color: 'primary', bg: 'lightgray', }} />
Read more in the custom pragma docs.
MDX Components
Use the components
prop to add components to MDX scope.
This can be used to introduce new behavior to markdown elements in MDX, such as adding linked headings or live-editable code examples.
Internally, the ThemeProvider
is a combination of MDXProvider
and Emotion's ThemeProvider
.
// with mdx components import React from 'react' import { ThemeProvider } from 'theme-ui' import mdxComponents from './mdx-components' import theme from './theme' export default props => <ThemeProvider components={mdxComponents} theme={theme}> {props.children} </ThemeProvider>
theme.styles
The MDX components can also be styled via the theme.styles
object.
This can be used as a mechanism to pass in fully-baked themes and typographic styles to MDX content.
// example theme export default { colors: { primary: '#33e', }, styles: { // this styles child MDX `<h1>` components h1: { fontSize: 32, // this value comes from the `color` object color: 'primary', }, } }
Styled components
These components' styles can also be consumed outside of an MDX doc with the Styled
component.
Note that these are only styled using the same theme.styles
object and not the same components passed to the ThemeProvider
context.
import React from 'react' import { Styled } from 'theme-ui' export default props => <Styled.div> <Styled.h1> Hello </Styled.h1> </Styled.div>
To change the underlying component in Styled
, use the as
prop.
<Styled.a as={Link} to='/'>Home</Styled.a>
Layout Components
Theme UI includes several components for creating page layouts.
Layout
: sets a flex column with 100vh min-heightHeader
: flexbox rowFooter
: flexbox rowMain
: flex auto container for pushing the Footer to the bottom of the viewportContainer
: max-width, centered container
import React from 'react' import { Layout, Header, Main, Container, Footer } from 'theme-ui' export default props => <Layout> <Header> Hello </Header> <Main> <Container> {props.children} </Container> </Main> <Footer> © 2019 </Footer> </Layout>
Box & Flex
The Box
& Flex
layout components are similar to the ones found in Rebass, but are built with Emotion and @styled-system/css
.
import React from 'react' import { Flex, Box } from 'theme-ui' export default props => <Flex flexWrap='wrap'> <Box width={[ 1, 1/2 ]} /> <Box width={[ 1, 1/2 ]} /> </Flex>
More Documentation
MIT License
Recommend
-
62
README.md Pine
-
43
README.md
-
30
README.md Conference App in a Box This repo goes along with my Dev.to post titled
-
26
This post is part of a 3 part series. Consider readingpart 1 andpart 2 first, although this post should still make sense on its own. Some people like to see the finished product first. If this is you, you can se...
-
14
Darklaf - A themeable swing Look and Feel This project is based on the darcula look and feel for Swing. Screenshots
-
8
Keeping a React Design System consistent: using visual regression testing to save time and headachesEnsuring visual consistency is one of the biggest challenges of working on a UI component...
-
10
theme-csx A utility React Native theming framework for rapidly building themeable components. Features Similar to standard react native styling, but with additional props that can be added to make it themeable.
-
4
Case Study: How TeamPassword Builds Consistent Design System and Product with UXPin Merge
-
7
The Many Faces of Themeable Design Systems Very rarely is exactly one design system created to serve exactly one product that expresses exactly one design language. Nearly all the d...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK