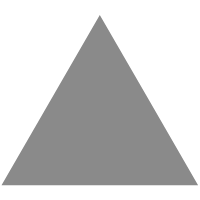
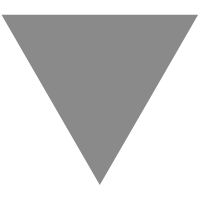
如何在 JS 循环中正确使用 async 与 await
source link: https://juejin.im/post/5cf7042df265da1ba647d9d1
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
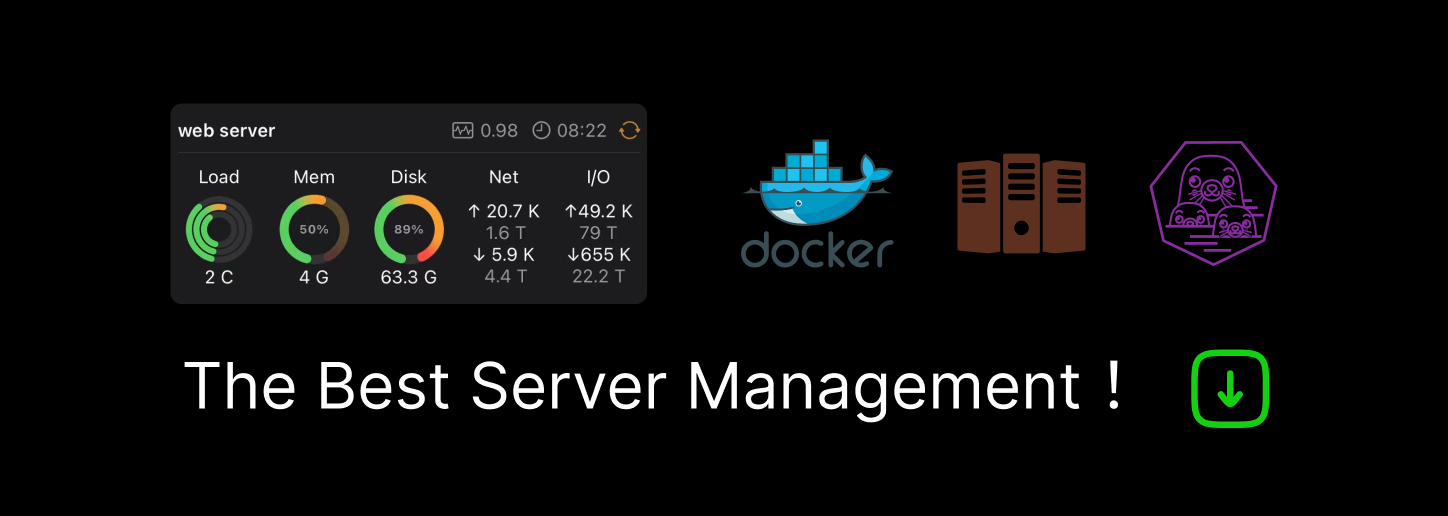
如何在 JS 循环中正确使用 async 与 await
译者:前端小智
点赞再看,微信搜索 【大迁世界】 关注这个没有大厂背景,但有着一股向上积极心态人。本文
GitHub
github.com/qq449245884… 上已经收录,文章的已分类,也整理了很多我的文档,和教程资料。
大家都说简历没项目写,我就帮大家找了一个项目,还附赠【搭建教程】。
在本文中,分享一些在如果循环中使用await
值得注意的问题。
准备一个例子
对于这篇文章,假设你想从水果篮中获取水果的数量。
const fruitBasket = {
apple: 27,
grape: 0,
pear: 14
};
复制代码
你想从fruitBasket
获得每个水果的数量。 要获取水果的数量,可以使用getNumFruit
函数。
const getNumFruit = fruit => {
return fruitBasket[fruit];
};
const numApples = getNumFruit('apple');
console.log(numApples); //27
复制代码
现在,假设fruitBasket
是从服务器上获取,这里我们使用 setTimeout
来模拟。
const sleep = ms => {
return new Promise(resolve => setTimeout(resolve, ms))
};
const getNumFruit = fruit => {
return sleep(1000).then(v => fruitBasket[fruit]);
};
getNumFruit("apple").then(num => console.log(num)); // 27
复制代码
最后,假设你想使用await
和getNumFruit
来获取异步函数中每个水果的数量。
const control = async _ => {
console.log('Start')
const numApples = await getNumFruit('apple');
console.log(numApples);
const numGrapes = await getNumFruit('grape');
console.log(numGrapes);
const numPears = await getNumFruit('pear');
console.log(numPears);
console.log('End')
}
复制代码
在 for 循环中使用 await
首先定义一个存放水果的数组:
const fruitsToGet = [“apple”, “grape”, “pear”];
复制代码
循环遍历这个数组:
const forLoop = async _ => {
console.log('Start');
for (let index = 0; index < fruitsToGet.length; index++) {
// 得到每个水果的数量
}
console.log('End')
}
复制代码
在for
循环中,过上使用getNumFruit
来获取每个水果的数量,并将数量打印到控制台。
由于getNumFruit
返回一个promise
,我们使用 await
来等待结果的返回并打印它。
const forLoop = async _ => {
console.log('start');
for (let index = 0; index < fruitsToGet.length; index ++) {
const fruit = fruitsToGet[index];
const numFruit = await getNumFruit(fruit);
console.log(numFruit);
}
console.log('End')
}
复制代码
当使用await
时,希望JavaScript暂停执行,直到等待 promise 返回处理结果。这意味着for
循环中的await
应该按顺序执行。
结果正如你所预料的那样。
“Start”;
“Apple: 27”;
“Grape: 0”;
“Pear: 14”;
“End”;
复制代码
这种行为适用于大多数循环(比如while
和for-of
循环)…
但是它不能处理需要回调的循环,如forEach
、map
、filter
和reduce
。在接下来的几节中,我们将研究await
如何影响forEach
、map和filter
。
在 forEach 循环中使用 await
首先,使用 forEach
对数组进行遍历。
const forEach = _ => {
console.log('start');
fruitsToGet.forEach(fruit => {
//...
})
console.log('End')
}
复制代码
接下来,我们将尝试使用getNumFruit
获取水果数量。 (注意回调函数中的async
关键字。我们需要这个async
关键字,因为await
在回调函数中)。
const forEachLoop = _ => {
console.log('Start');
fruitsToGet.forEach(async fruit => {
const numFruit = await getNumFruit(fruit);
console.log(numFruit)
});
console.log('End')
}
复制代码
我期望控制台打印以下内容:
“Start”;
“27”;
“0”;
“14”;
“End”;
复制代码
但实际结果是不同的。在forEach
循环中等待返回结果之前,JavaScrip先执行了 console.log('End')。
实际控制台打印如下:
‘Start’
‘End’
‘27’
‘0’
‘14’
复制代码
JavaScript 中的 forEach
不支持 promise 感知,也不支持 async
和await
,所以不能在 forEach
使用 await
。
在 map 中使用 await
如果在map
中使用await
, map
始终返回promise
数组,这是因为异步函数总是返回promise
。
const mapLoop = async _ => {
console.log('Start')
const numFruits = await fruitsToGet.map(async fruit => {
const numFruit = await getNumFruit(fruit);
return numFruit;
})
console.log(numFruits);
console.log('End')
}
“Start”;
“[Promise, Promise, Promise]”;
“End”;
复制代码
如果你在 map
中使用 await
,map
总是返回promises
,你必须等待promises
数组得到处理。 或者通过await Promise.all(arrayOfPromises)
来完成此操作。
const mapLoop = async _ => {
console.log('Start');
const promises = fruitsToGet.map(async fruit => {
const numFruit = await getNumFruit(fruit);
return numFruit;
});
const numFruits = await Promise.all(promises);
console.log(numFruits);
console.log('End')
}
复制代码
运行结果如下:
如果你愿意,可以在promise
中处理返回值,解析后的将是返回的值。
const mapLoop = _ => {
// ...
const promises = fruitsToGet.map(async fruit => {
const numFruit = await getNumFruit(fruit);
return numFruit + 100
})
// ...
}
“Start”;
“[127, 100, 114]”;
“End”;
复制代码
在 filter 循环中使用 await
当你使用filter
时,希望筛选具有特定结果的数组。假设过滤数量大于20的数组。
如果你正常使用filter
(没有 await),如下:
const filterLoop = _ => {
console.log('Start')
const moreThan20 = fruitsToGet.filter(async fruit => {
const numFruit = await fruitBasket[fruit]
return numFruit > 20
})
console.log(moreThan20)
console.log('END')
}
复制代码
Start
["apple"]
END
复制代码
filter
中的await
不会以相同的方式工作。 事实上,它根本不起作用。
const filterLoop = async _ => {
console.log('Start')
const moreThan20 = await fruitsToGet.filter(async fruit => {
const numFruit = fruitBasket[fruit]
return numFruit > 20
})
console.log(moreThan20)
console.log('END')
}
// 打印结果
Start
["apple", "grape", "pear"]
END
复制代码
为什么会发生这种情况?
当在filter
回调中使用await
时,回调总是一个promise
。由于promise
总是真的,数组中的所有项都通过filter
。在filter
使用 await
类以下这段代码
const filtered = array.filter(true);
复制代码
在filter
使用 await
正确的三个步骤
-
使用
map
返回一个promise 数组 -
使用
await
等待处理结果 -
使用
filter
对返回的结果进行处理const filterLoop = async _ => { console.log('Start');
const promises = await fruitsToGet.map(fruit => getNumFruit(fruit));
const numFruits = await Promise.all(promises);
const moreThan20 = fruitsToGet.filter((fruit, index) => { const numFruit = numFruits[index]; return numFruit > 20; })
console.log(moreThan20); console.log('End') }
在 reduce 循环中使用 await
如果想要计算 fruitBastet
中的水果总数。 通常,你可以使用reduce
循环遍历数组并将数字相加。
const reduceLoop = _ => {
console.log('Start');
const sum = fruitsToGet.reduce((sum, fruit) => {
const numFruit = fruitBasket[fruit];
return sum + numFruit;
}, 0)
console.log(sum)
console.log('End')
}
复制代码
运行结果:
当你在 reduce
中使用await
时,结果会变得非常混乱。
const reduceLoop = async _ => {
console.log('Start');
const sum = await fruitsToGet.reduce(async (sum, fruit) => {
const numFruit = await fruitBasket[fruit];
return sum + numFruit;
}, 0)
console.log(sum)
console.log('End')
}
复制代码
[object Promise]14
是什么 鬼??
剖析这一点很有趣。
-
在第一次遍历中,
sum
为0
。numFruit
是27
(通过getNumFruit(apple)
的得到的值),0 + 27 = 27
。 -
在第二次遍历中,
sum
是一个promise
。 (为什么?因为异步函数总是返回promises
!)numFruit
是0
.promise 无法正常添加到对象,因此JavaScript将其转换为[object Promise]
字符串。[object Promise] + 0
是object Promise] 0
。 -
在第三次遍历中,
sum
也是一个promise
。numFruit
是14
.[object Promise] + 14
是[object Promise] 14
。
解开谜团!
这意味着,你可以在reduce
回调中使用await
,但是你必须记住先等待累加器!
const reduceLoop = async _ => {
console.log('Start');
const sum = await fruitsToGet.reduce(async (promisedSum, fruit) => {
const sum = await promisedSum;
const numFruit = await fruitBasket[fruit];
return sum + numFruit;
}, 0)
console.log(sum)
console.log('End')
}
复制代码
但是从上图中看到的那样,await
操作都需要很长时间。 发生这种情况是因为reduceLoop
需要等待每次遍历完成promisedSum
。
有一种方法可以加速reduce
循环,如果你在等待promisedSum之前先等待getNumFruits()
,那么reduceLoop
只需要一秒钟即可完成:
const reduceLoop = async _ => {
console.log('Start');
const sum = await fruitsToGet.reduce(async (promisedSum, fruit) => {
const numFruit = await fruitBasket[fruit];
const sum = await promisedSum;
return sum + numFruit;
}, 0)
console.log(sum)
console.log('End')
}
复制代码
这是因为reduce
可以在等待循环的下一个迭代之前触发所有三个getNumFruit
promise。然而,这个方法有点令人困惑,因为你必须注意等待的顺序。
在reduce中使用wait最简单(也是最有效)的方法是
-
使用
map
返回一个promise 数组 -
使用
await
等待处理结果 -
使用
reduce
对返回的结果进行处理const reduceLoop = async _ => { console.log('Start');
const promises = fruitsToGet.map(getNumFruit); const numFruits = await Promise.all(promises); const sum = numFruits.reduce((sum, fruit) => sum + fruit);
console.log(sum) console.log('End') }
这个版本易于阅读和理解,需要一秒钟来计算水果总数。
从上面看出来什么
-
如果你想连续执行
await
调用,请使用for
循环(或任何没有回调的循环)。 -
永远不要和
forEach
一起使用await
,而是使用for
循环(或任何没有回调的循环)。 -
不要在
filter
和reduce
中使用await
,如果需要,先用map
进一步骤处理,然后在使用filter
和reduce
进行处理。
文章每周持续更新,可以微信搜索「 大迁世界 」第一时间阅读和催更(比博客早一到两篇哟),本文 GitHub github.com/qq449245884… 已经收录,整理了很多我的文档,欢迎Star和完善,大家面试可以参照考点复习,另外关注公众号,后台回复福利,即可看到福利,你懂的。
Recommend
-
126
在很长一段时间里面,FE们不得不依靠回调来处理异步代码。使用回调的结果是,代码变得很纠结,不便于理解与维护,值得庆幸的是Promise带来了.then(),让代码变得井然有序,便于管理。于是我们大量使用,代替了原来的回调方式。但是不存在一种方法可以让当前的执行流...
-
76
ASP.NET 谨用 async/await
-
75
C# 5.0 引入 async/await 关键字,旨在简化异步编程模型,抛去语法糖就是 Net4.0 的 Task + 状态机。其实在处理异步编程使用 Task 还是挺简单的,不过既然推出了新的语法糖,难免会尝试一下,然而在使用中却没想象中那么单纯。以下针对ASP.NET 应用程序实际使用过...
-
82
So I finally hopped on the boat with usa...
-
12
Golang 是一种并发编程语言。它具有强大的特性,如 Goroutines 和 Channels,可以很好地处理异步任务。另外,goroutines 不是 OS 线程,这就是为什么您可以在不增加开销的情况下根据需要启动任意数量的 goroutine...
-
9
.NET Web应用中为什么要使用async/await异步编程?前言1.什么是async/aw...
-
5
async/await 使用方式先说一下async的用法,它作为一个关键字放到函数前面,用于表示函数是一个异步函数,因为async就是异步的意思, 异步函数也就意味着该函数的执行不...
-
6
async && await概念async使用async关键字声明的函数,是AsyncFunction构造函数的实例,在async函数体内,可以使用await接收promise实例async和await关...
-
11
async...await在tcp通讯中的正确用法 编...
-
5
器→工具, 编程语言 PEP 492:使用 async 和 awa...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK