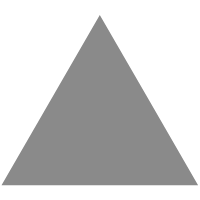
28
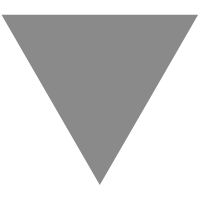
controller如何拿到自定义view的点击事件?
source link: https://juejin.im/post/5cebeb816fb9a07ed136b4ad
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
controller如何拿到自定义view的点击事件?
2019年05月27日
阅读 1572
controller如何拿到自定义view的点击事件?
如下图所示:自定义PersonalCenterView,如何在controller拿到按钮(小箭头)的点击方法?
一、UI布局代码如下所示
- (void)initUI
{
//子视图
[self addSubview:self.headImageView];
[self addSubview:self.nameLabel];
[self addSubview:self.autographLabel];
[self addSubview:self.pushButton];
}
- (void)layoutSubviews
{
[super layoutSubviews];
[self.headImageView mas_makeConstraints:^(MASConstraintMaker *make) {
make.left.mas_equalTo(20);
make.top.mas_equalTo(20);
make.width.mas_equalTo(60);
make.height.mas_equalTo(60);
}];
[self.nameLabel mas_makeConstraints:^(MASConstraintMaker *make) {
make.left.mas_equalTo(self.headImageView.mas_right).mas_offset(15);
make.top.mas_equalTo(25);
make.height.mas_equalTo(20);
}];
[self.autographLabel mas_makeConstraints:^(MASConstraintMaker *make) {
make.left.mas_equalTo(self.headImageView.mas_right).mas_offset(15);
make.top.mas_equalTo(self.nameLabel.mas_bottom).mas_offset(10);
make.height.mas_equalTo(20);
}];
[self.pushButton mas_makeConstraints:^(MASConstraintMaker *make) {
make.centerY.mas_equalTo(self.mas_centerY);
make.right.mas_equalTo(-20);
make.width.mas_equalTo(10);
make.height.mas_equalTo(17);
}];
}
- (UIImageView*)headImageView
{
if (!_headImageView) {
_headImageView = [[UIImageView alloc]init];
_headImageView.backgroundColor = [UIColor redColor];
_headImageView.layer.masksToBounds = YES;
_headImageView.layer.cornerRadius = 30;
_headImageView.image = [UIImage imageNamed:@"head.jpg"];
}
return _headImageView;
}
- (UILabel*)nameLabel
{
if (!_nameLabel) {
_nameLabel = [[UILabel alloc]init];
_nameLabel.font = [UIFont systemFontOfSize:15];
_nameLabel.text = @"陈小丸🍡";
}
return _nameLabel;
}
- (UILabel*)autographLabel
{
if (!_autographLabel) {
_autographLabel = [[UILabel alloc]init];
_autographLabel.font = [UIFont systemFontOfSize:13];
_autographLabel.numberOfLines = 0;
_autographLabel.text = @"愿你不知人间疾苦,过得无拘无束";
}
return _autographLabel;
}
- (UIButton*)pushButton
{
if (!_pushButton) {
_pushButton = [UIButton buttonWithType:(UIButtonTypeCustom)];
[_pushButton setBackgroundImage:[UIImage imageNamed:@"force_icon"] forState:UIControlStateNormal];
}
return _pushButton;
}
复制代码
二、实现方式
1.SEL
1.h文件
//指定初始化方法
- (instancetype)initWithFrame:(CGRect)frame target:(id)target sel:(SEL)action;
复制代码
2.m文件
- (instancetype)initWithFrame:(CGRect)frame target:(id)target sel:(SEL)sel
{
self = [super initWithFrame:frame];
if (self) {
//背景颜色
self.backgroundColor = [UIColor groupTableViewBackgroundColor];
//子视图
[self initUI];
[self.pushButton addTarget:target action:sel forControlEvents:(UIControlEventTouchUpInside)];
}
return self;
}
复制代码
3.controller文件
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
PersonalCenterView *centerView = [[PersonalCenterView alloc]initWithFrame:CGRectMake(0, 88, [UIScreen mainScreen].bounds.size.width, 100) target:self sel:@selector(clickEnter)];
[self.view addSubview:centerView];
}
- (void)clickEnter
{
//处理点击事件
NSLog(@"箭头被点击了~");
}
复制代码
1.h文件
#import <UIKit/UIKit.h>
NS_ASSUME_NONNULL_BEGIN
@protocol PersonalCenterViewDelegate <NSObject>
- (void)clickEnter;
@end
@interface PersonalCenterView : UIView
//代理实现
@property (nonatomic, weak) id<PersonalCenterViewDelegate>delegate;
@end
NS_ASSUME_NONNULL_END
复制代码
2.m文件
//pushButton点击事件
- (void)clickAction:(UIButton*)button
{
if ([self.delegate respondsToSelector:@selector(clickEnter)]) {
[self.delegate clickEnter];
}
}
复制代码
3.controller文件
@interface ViewController ()<PersonalCenterViewDelegate>
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
//代理实现通信
PersonalCenterView *centerView = [[PersonalCenterView alloc]initWithFrame:CGRectMake(0, 88, [UIScreen mainScreen].bounds.size.width, 100)];
centerView.delegate = self;
[self.view addSubview:centerView];
}
- (void)clickEnter
{
//处理点击事件
NSLog(@"箭头被点击了~");
}
复制代码
3.block
1.h文件
//指定初始化方法
- (instancetype)initWithFrame:(CGRect)frame clickBlock:(void(^)(void))clickBlock;
复制代码
2.m文件
- (instancetype)initWithFrame:(CGRect)frame clickBlock:(void(^)(void))clickBlock
{
self = [super initWithFrame:frame];
if (self) {
//背景颜色
self.backgroundColor = [UIColor groupTableViewBackgroundColor];
//子视图
[self initUI];
self.clickBlock = clickBlock;
}
return self;
}
- (void)clickAction:(UIButton*)button
{
if(self.clickBlock)
{
self.clickBlock();
}
}
复制代码
3.controller文件
PersonalCenterView *centerView = [[PersonalCenterView alloc]initWithFrame:CGRectMake(0, 88, [UIScreen mainScreen].bounds.size.width, 100) clickBlock:^{
//处理点击事件
NSLog(@"箭头被点击了~");
}];
复制代码
4.kvo
1.m文件
- (UIButton*)pushButton
{
if (!_pushButton) {
_pushButton = [UIButton buttonWithType:(UIButtonTypeCustom)];
[_pushButton setBackgroundImage:[UIImage imageNamed:@"force_icon"] forState:UIControlStateNormal];
[_pushButton addTarget:self action:@selector(clickAction:) forControlEvents:(UIControlEventTouchUpInside)];
}
return _pushButton;
}
- (void)clickAction:(UIButton*)button
{
//触发kvo
button.selected = !button.selected;
}
复制代码
2.controller文件
//采用facebook开源的第三方 备注:这里这是拿来举例说明通信方式 这样的场景不适合
PersonalCenterView *centerView = [[PersonalCenterView alloc]initWithFrame:CGRectMake(0, 88, [UIScreen mainScreen].bounds.size.width, 100)];
FBKVOController *KVOController = [FBKVOController controllerWithObserver:self];
[KVOController observe:centerView.pushButton keyPath:@"selected" options:(NSKeyValueObservingOptionNew) block:^(id _Nullable observer, id _Nonnull object, NSDictionary<NSString *,id> * _Nonnull change) {
NSLog(@"%@",change);
//处理点击事件 无需判断 因为按钮被点击就会走
NSLog(@"箭头被点击了~");
}];
复制代码
5.ReactiveCocoa
1.h文件
@property (nonatomic,strong) RACSubject* clickSubject;
复制代码
2.m文件
- (instancetype)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
self.clickSubject = [RACSubject subject];
//背景颜色
self.backgroundColor = [UIColor groupTableViewBackgroundColor];
//子视图
[self initUI];
}
return self;
}
- (UIButton*)pushButton
{
if (!_pushButton) {
_pushButton = [UIButton buttonWithType:(UIButtonTypeCustom)];
[_pushButton setBackgroundImage:[UIImage imageNamed:@"force_icon"] forState:UIControlStateNormal];
[[_pushButton rac_signalForControlEvents:UIControlEventTouchUpInside] subscribeNext:^(UIButton * button) {
[self.clickSubject sendNext:button];
}];
}
return _pushButton;
}
复制代码
3.controller文件
PersonalCenterView *centerView = [[PersonalCenterView alloc]initWithFrame:CGRectMake(0, 88, [UIScreen mainScreen].bounds.size.width, 100)];
[centerView.clickSubject subscribeNext:^(UIButton *button) {
//处理点击事件
NSLog(@"箭头被点击了~");
}];
复制代码
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK