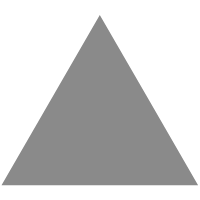
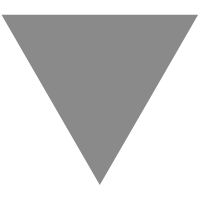
An Extensive Kotlinx Serializer Library For Serialization | Android | Kotlin
source link: https://ahsensaeed.com/kotlinx-serialization-library-android-kotlin/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
An Extensive Kotlinx Serializer Library For Serialization
In IO 2019 Hadi Hariri talked about a library called Kotlinx.Serialization. Frankly speaking before than, I’ve never known any serialization library officially provided by Kotlin and we could use platform supported serialization libraries with Kotlin classes(eg. Gson for JVM/Android).
Now there’s a library called Kotlinx.Serialization provided by Kotlin officials. It is a runtime library it uses generated code to serialize object without reflection, cross-platform and support multiple formats.
To learn more about reflection check out this post.
Add Dependency For Gradle Project
Setting up your project to use the serialization is quite simple. First, add the following classpath to your project-level build.gradle file.
buildscript { ext.kotlin_version = '1.3.31' repositories { jcenter() } dependencies { classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" classpath "org.jetbrains.kotlin:kotlin-serialization:$kotlin_version" } }
Next, add the plugin inside the app-level build.gradle file.
apply plugin: 'kotlinx-serialization' apply plugin: 'kotlin' // 'kotlin-android' for Android-specific or kotlin-multiplatform' for multiplatform projects
Finally, add the dependency for Kotlinx Serialization runtime library.
dependencies { compile "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version" compile "org.jetbrains.kotlinx:kotlinx-serialization-runtime:0.11.0" }
Add Dependency For Maven Project
In order to add Kotlinx Serialization into your maven project. Please do check out the Maven section inside the GitHub repo.
Serializing Data Class
Serialization on data classes can be done quite easily. Let’s see a quick example of it.
@Serializable data class Person constructor( private val id: Int = 0, private val name: String, private val height: Float ) fun main() { val person = Person(name = "Ahsen Saeed", height = 5.9f) println(Json.stringify(Person.serializer(), person)) } // Output of above program {"id":0,"name":"Ahsen Saeed","height":5.9}
Like the above, you can see I have a Person data class and added the @Serializable to it, which is part of Kotlin serialization library. And in the main function the Json.stringify method simply converts a serializable object to Json string.
Now let’s see an example where we convert a well-formed Json back to data class.
@Serializable data class Person constructor( private val id: Int = 0, private val name: String, private val height: Float ) fun main() { val personJson = "{\"id\":0,\"name\":\"Ahsen Saeed\",\"height\":5.9}" println(Json.parse(Person.serializer() ,personJson)) } // Output of above program Person(id=0, name='Ahsen Saeed', height=5.9)
You see this time I do Json.parse, passing again the serializer
for Person class, and then the actual Json that I wanna parse.
The ease and simplicity which the Kotlinx Serialization gives us to convert Json to object and object to Json is absolutely amazing.
Note: This can as well work with simple classes.
SERIALIZING Collection of DATA CLASS
The next thing I wanna show you the quick example of how we can we convert our list of Persons into simple Json String.
@Serializable data class Person constructor( private val id: Int = 0, private val name: String, private val height: Float ) val persons = listOf(Person(name = "Ahsen Saeed", height = 5.9f), Person(4, "Shehryar Khan", 5.7f)) fun main() { println(Json.stringify(Person.serializer().list, persons)) } // Output of the above program [{"id":0,"name":"Ahsen Saeed","height":5.9},{"id":4,"name":"Shehryar Khan","height":5.7}]
Now convert back to our List<Person> from Json.
@Serializable data class Person constructor( private val id: Int = 0, private val name: String, private val height: Float ) fun main() { val personsJson = "[{\"id\":0,\"name\":\"Ahsen Saeed\",\"height\":5.9},{\"id\":4,\"name\":\"Shehryar Khan\",\"height\":5.7}]" val persons = Json.parse(Person.serializer().list, personsJson) println(persons) } // Output of above program [Person(id=0, name=Ahsen Saeed, height=5.9), Person(id=4, name=Shehryar Khan, height=5.7)]
Kotlinx Serialization Converter For Retrofit
Android developers who would like to use this Kotlinx Serialization library for converting the Json response into objects when working with Retrofit. An adapter is available created by mighty Jake Wharton. You can found more info of adapter here on this link. First, add the dependency.
implementation 'com.jakewharton.retrofit:retrofit2-kotlinx-serialization-converter:0.4.0'
Now add a converter factory when building you Retrofit
instance using the asConverterFactory
extension function:
val contentType = MediaType.get("application/json") val retrofit = Retrofit.Builder() .baseUrl("https://203.***.***.**:***/api/") .addConverterFactory(Json.asConverterFactory(contentType)) .build()
Note: The serialization won’t work unless you add the @Serializable to your response classes and on @Body
type of class.
Currently Kotlinx Serialization supports three formats and many more will come in future.
- Protobuf formats
There so much more inside the Kotlinx.Serialization library you can read more here on official GitHub repo.
If you like this article press the ♥️ icon below.
Thank you for being here and keep reading…
More Resources
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK