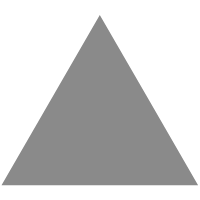
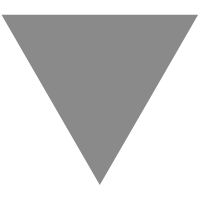
ESLint + Development Workflow
source link: https://www.tuicool.com/articles/fyU3ieE
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.


What is ESLint?
It is a linting tool for Javascript.
What problem does ESLint solve?
It automates the process of identifying some common mistakes/nitpicks involved in your JS code, based on predefined patterns which are inspired by some of the best practices in JS.
Why do you need to use it?
I am sure you have been there leaving review comments on a PR, similar to ones like:
“please use ES6 string literal here.”
“please make sure you use this variable a const as it never being reassigned after the declaration.”
“please use ES6 spread operator here.”
and the list goes on!
ESLint to the rescue! it will make sure you will never have to worry about leaving another such review comment on a PR ever again. cool, isn't it!
What I am trying to convey here is that ESLint will help you in adopting some sort of coding standard for your JS codebase period.
How to get started with it, quickly
Trust me it is easier than you think to get started with using ESLint in your awesome codebase. its time to make it even more awesome!
you need to install ESLint first
npm i --save-dev eslint
this will save ESLint as your development dependency. after all, you need ESLint only for your development purpose.
Its time you run ESLint on your codebase to check possible errors you have made so far.
npx eslint .
or
./node_modules/.bin/eslint .
above commands will make sure you are always running the local version of ESLint which is installed inside your codebase instead of the globally installed version of ESLint if any.
Note: I will use the npx way of doing things further in this post.
Now you might have gotten an error saying,
No ESLint configuration found.
No need to panic as ESLint requires a config file to be set up for it to start showing off. let's create one now,
npx eslint --init
this will ask you some series of question on how you want to make use of ESLint in your codebase. I have captured one for you,


I think this would be suitable for your project and definitely a good start if you don’t know exactly what you want from ESLint at this moment.
generated config would look something like this

now assume we have some Javascript code as below and see what ESLint make of this.

let's try to run ESLint now
// this will run ESLint on this file only npx eslint ./eslinttest.js
// this will run ESLint on all JS files in your project directory npx eslint **/*.js
below is the output from ESLint


We just caught a few of those things which needed your review comments for the fix! Woohoo!
Okay, now that we know our code has some issues how do we fix this? ESLint got your back here as well. it can fix some of the above issues for you, which can be done by running
npx eslint --fix ./eslinttest.js
fix option will command ESLint to automatically fix those errors which it will catch during the pass.
Note: ESLint may not fix automatically all of the errors it encounters in your code.
Let's see how our JS code looks after we asked ESLint to fix our errors by itself.

Great! ESLint got us covered in some places like it fixed string concatenation with ES6 string template feature and replaced single quotes with double quotes(this is ESLint default you can change it to use single quotes). but it didn’t remove console statements by itself because ESLint knows its limits! ;)
So far it’s been great. now, what if you want all the var declarations to be replaced with ES6 let/const declarations?
Well with ESLint its as simple as modifying a rule inside configuration. if you check the config we previously created and search for no-var you will find
rule: { ... ... no-var: "off", ... ... }
Well, this means the rule no-var is been turned off from checking by ESLint during the pass. before we turn this rule on, we need to understand about ESLint rule.
ESLint rule possibly can have below 3 values:
0 = off, 1 = warn, 2 = error.
So we want ESLint to throw an error whenever it encounters var declarations. for that, we will change the config to:
rule: { ... ... no-var: "error", ... ... }
Now when we run ESLint will behave the way you expected and throws an error whenever it encounters var. if you run it with fix option then it will automatically replace all var with const/let based on their usage in the code. so cool!.
Fitting ESLint in your development workflow
Now, this is an important thing which can impact your development workflow especially if you work in a team which is usually the case most of the time.
The point here is how big is your codebase at this moment while you are reading this thinking you want to introduce ESLint. by big codebase, I mean the number of lines of JS in your code and the number of developers who are working on it.
If you are just starting out then its a no brainer you choose to start using ESLint from day one. If not then you need to consider two factors before you use ESLint:
eslint:recommended
I will explain the way we went on about it,
- At first, we generated an HTML lint report which had all the existing errors in our codebase caught be ESLint. which can be generated by
npx eslint --ext .js -f html -o ./lint-report/errors.html **/*.js
the report looks something like this.


using this is straight forward and self-explanatory. if not you can visit the official documentation about the rule for more details, which is again provided in this report.
2. We decided to fix these errors first before we go about enforcing ESLint somehow in our development workflow.
3. All the errors are fixed at this point!. Now we placed a lint check during code commit which will make sure the code which is about to get into our codebase is passing all the lint checks. which can be done with the help of something we call it as pre-commit hooks. it is again quite simple to achieve this, follow the below steps.
install husky
npm i husky --save-dev
create a pre-commit hook like one below in package.json file
"husky": {
"hooks": {
"pre-commit": "./node_modules/.bin/eslint --ext .js src/** --fix"
}
}
this will make sure all your staged code is sent for a lint test. so now you have successfully automated this process which makes sure no code enters your codebase with lint errors in them.
Well, you have made it! as I mentioned earlier it was not that complicated after all dealing with ESLint.
Okay, that is all I had in my mind. hope you have found this post useful!.
Let's say together -> ESLint rocks!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK