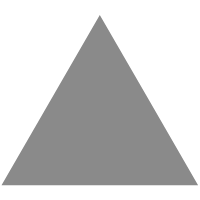
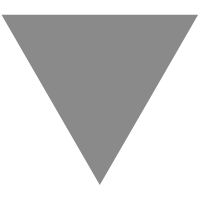
Format Data Into GeoJSON With JavaScript to Be Used With HERE XYZ
source link: https://www.tuicool.com/articles/iYVFRbB
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
If you're new to HERE XYZ or to the mapping space in general, you might not be familiar with GeoJSON. While GeoJSON is still JSON, it is formatted as part of a specification for geographic features.
We're going to see how to take ordinary location data and parse it into GeoJSON using JavaScript.
To get an idea of what we want to accomplish, take a look at the following image:

We're going to accept input and spit out GeoJSON data. Now, most of what we're going to do is completely overkill because we don't need a visual component and pretty much everything can be done in just a few lines of code. We're just going to make it interesting.
Somewhere on your computer, create a new index.html file and include the following boilerplate code:
<html> <body style="background-color: #DDD"> <table style="width: 800px"> <tr> <td>Input</td> <td>Output</td> </tr> <tr> <td valign="top" style="width: 50%; height: 400px"> <p> <label>Latitude:</label> <input type="text" id="latitude" value="37.7397" /> </p> <p> <label>Longitude:</label> <input type="text" id="longitude" value="-121.4252" /> </p> <p> <input type="text" id="propertyname1" value="date" /> <input type="text" id="propertyvalue1" value="20190509" /> </p> <p> <input type="text" id="propertyname2" value="foo" /> <input type="text" id="propertyvalue2" value="bar" /> </p> <p> <button type="button" onclick="createGeoJSON()">Create GeoJSON</button> </p> </td> <td valign="top" style="width: 50%; height: 400px"> <textarea id="output" style="width: 100%; height: 400px"></textarea> </td> </tr> </table> <script src="https://cdnjs.cloudflare.com/ajax/libs/geojson/0.5.0/geojson.min.js"></script> <script> // Logic here... </script> </body> </html>
In the above code we are adding some very basic form elements and importing GeoJSON.js . You don't need to be using browser-based HTML to make use of GeoJSON.js.
In the HTML form, we have two rows of input fields which we're going to use to represent dynamic user input for both the name and the value. We're doing this so we can add custom data to the GeoJSON.
Now let's start working with the data.
In the index.html file, add the following to the <script>
tags:
<script> const createGeoJSON = () => { const data = [ { lat: document.getElementById("latitude").value, lng: document.getElementById("longitude").value } ]; data[0][document.getElementById("propertyname1").value] = document.getElementById("propertyvalue1").value; data[0][document.getElementById("propertyname2").value] = document.getElementById("propertyvalue2").value; const dataGeoJSON = GeoJSON.parse(data, { Point: ["lat", "lng"] }); document.getElementById("output").value = JSON.stringify(dataGeoJSON, null, 4); } createGeoJSON(); </script>
The createGeoJSON
method will create an array of objects to be parsed. At a minimum, each object should contain a latitude and longitude. Next, we are taking our dynamic fields and adding them as properties to sit next to the latitude and longitude values. In theory, our data
array would look like the following:
[ { lat: 37, lng: -121, foo: "bar", date: "20190509" } ]
After we parse the data, we are choosing to update the <textarea>
with the final result, which would look something like the following:
{ "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry": { "type": "Point", "coordinates": [ -121.4252, 37.7397 ] }, "properties": { "date": "20190509", "foo": "bar" } } ] }
When it comes to HERE XYZ, the above GeoJSON data would work. However, there are other features that we can take advantage of that are specific to XYZ. For example, we can introduce tagging of our data. Tags are searchable components within our data, so if I created a tag based on a date, then I could search for all data that has that particular tag. To do this, we can make our code look like the following:
const data = [ { lat: document.getElementById("latitude").value, lng: document.getElementById("longitude").value, "@ns:com:here:xyz": { tags: [document.getElementById("propertyvalue1").value, document.getElementById("propertyvalue2").value] } } ];
Notice that we've introduced an @ns:com:here:xyz
property which is an object with various tags. It is up to you what you want to add here, or anything at all.
Conclusion
You just saw how to work with GeoJSON data in JavaScript which can later be used with HERE XYZ . Like I mentioned at the beginning, pretty much everything in this tutorial was overkill, but it does paint an easy picture on how to get your data ready.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK