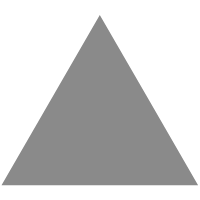
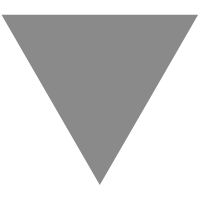
Learn React File Upload In 5 Minute
source link: https://www.tuicool.com/articles/YbY3eaz
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
This tutorial help to create file upload into react application.We will demonstrate step by step process to react file upload.
We will use nodejs API to file upload into server.The react application will use to upload file into client side and send file to the node api server.
Table of Contents
-
React Upload File in React
- How To Create React App
- Create File Upload UI
- React file upload component
React Upload File in React
Let’s integrate react file upload component into our react application.We will use nodejs application for upload file into server. Create Nodejs API to Upload file Using Multer example use to upload file into server.
We will use following dependencies into this project –
- Bootstrap 4 : We will use bootstrap for ui.
- ReactToastify : This libs are use to display alert notification.
- axios : Promise based HTTP client for the browser and node.js
How To Create React App
Let’s create react app using command line.The following command will create react scratch app –
npx create-react-app react-file-upload
We will cd into react project react-file-upload
–
cd react-file-upload
Now will install dependencies –
npm install bootstrap npm install react-toastify npm install axios
The bootstrap help to create ui based on bootstrap 4, react-toastify is use to display beautiful notification into react app and axios for HTTP client.
Now run application.
npm start
You can see react home page into browser http://localhost:3000
.
Let’s create file upload component into this application.
Create File Upload UI
I have taken reference from bootsnipp for UI, Lets add html into app.js
file.
import React, { Component } from 'react'; import axios from 'axios'; import { ToastContainer, toast } from 'react-toastify'; import './App.css'; class App extends Component { constructor(props) { super(props); this.state = { selectedFile: null } }; render() { return ( <div className="container"> <div className="row"> <div className="col-md-6"> <ToastContainer /> <form method="post" action="#" id="#"> <div className="form-group files"> <label>Upload Your File </label> <input type="file" name="file" className="form-control" onChange={this.onChangeHandler}/> </div> <div className="col-md-6 pull-right"> <button width="100%" type="button" className="btn btn-info" onClick={this.fileUploadHandler}>Upload File</button> </div> </form> </div> </div> </div> ); } } export default App;
Above HTML code, Added ToastContainer component to display notification.Created file input field to take user input and submit button for send request to server.
Added onchange
event into input field and associated method onChangeHandler
to set file information into state.
The fileUploadHandler
method associated with submit onclick
event.This method send request to nodejs server using axios HTTP client.
Now added upload form css styling into app.css
file –
.files input { outline: 2px dashed #92b0b3; outline-offset: -10px; -webkit-transition: outline-offset .15s ease-in-out, background-color .15s linear; transition: outline-offset .15s ease-in-out, background-color .15s linear; padding: 120px 0px 85px 35%; text-align: center !important; margin: 0; width: 100% !important; } .files input:focus{ outline: 2px dashed #92b0b3; outline-offset: -10px; -webkit-transition: outline-offset .15s ease-in-out, background-color .15s linear; transition: outline-offset .15s ease-in-out, background-color .15s linear; border:1px solid #92b0b3; } .files{ position:relative} .files:after { pointer-events: none; position: absolute; top: 60px; left: 0; width: 50px; right: 0; height: 56px; content: ""; background-image: url(https://image.flaticon.com/icons/png/128/109/109612.png); display: block; margin: 0 auto; background-size: 100%; background-repeat: no-repeat; } .color input{ background-color:#f1f1f1;} .files:before { position: absolute; bottom: 10px; left: 0; pointer-events: none; width: 100%; right: 0; height: 37px; content: " or drag it here. "; display: block; margin: 0 auto; color: #2ea591; font-weight: 600; text-transform: capitalize; text-align: center; }
React file upload component
We will create react file upload component functionality into app.js
file.
onChangeHandler=event=>{ var file = event.target.files[0]; console.log(file); console.log(this.validateSize(event)); if(this.validateSize(event)){ console.log(file); // if return true allow to setState this.setState({ selectedFile: file }); } } fileUploadHandler = () => { const data = new FormData() console.log(this.state.selectedFile); data.append('file', this.state.selectedFile) console.log(data); axios.post("http://localhost:8010/api/v1/upload", data) .then(res => { // then print response status toast.success('upload success') }) .catch(err => { // then print response status toast.error('upload fail') }) }; validateSize=(event)=>{ let file = event.target.files[0]; let size = 30000; let err = ''; console.log(file.size); if (file.size > size) { err = file.type+'is too large, please pick a smaller file\n'; toast.error(err); } return true };
The onChangeHandler
method takes file name from file input field, Also validate file using size, you can add many validation rule to validate file.
The validateSize
method is use validate file size.If size is exceeded then throw message and displaying using toaster.
The fileUploadHandler
method take file name from state and send request to node server.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK