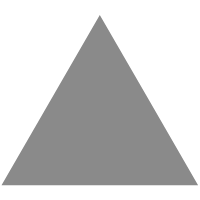
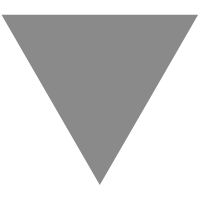
Reliable Synchronization of Web Clients: Introducing Resgate
source link: https://www.tuicool.com/articles/fmEzQ3Y
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
REST APIs are nice. Simple. Stateless. Scalable. But nowadays, your reactive web client's view (React / Vue.js / Angular) is expected to be updated in real time. No F5 reloading.
Often you add an event stream to send events whenever something changes. But then you have two channels sending information regarding the same data. And this is where the problems start:
How do I synchronize the REST API with the event stream?
And lost connections. Perhaps I should buffer the stream for reconnects?
Maybe I shouldn't send all events to all clients all the time. Let's use topics.
Can I filter unauthorized events? Let's use more topics!
Is it even possible to make search or pagination queries with real-time updates?
Can I simplify the client-side event handling code somehow?
Will this scale?
Why can’t it be made simpler?!
Being the lead developer of the cloud offering at a leading provider of contact center solutions, I had to deal with these issues. And I've seen multiple other companies trying to solve the same problem as well. Let me introduce a new solution.
Resgate : Realtime API Gateway
Resgate
Resgate is a Realtime API Gateway , written in Go. It is a single executable file that requires little to no configuration. Taking advantage of Go's concurrency model, it is highly performant with event latencies < 1ms. By acting as a bridge between the web clients and your service (or microservices), it will fetch resources, forward method calls, and handle events, while also providing access control, synchronization, caching, and more.
Clients connect to Resgate using either HTTP or WebSocket, to make requests. Resgate in turn keeps track of which resources each client has requested, keeping it reliably updated for as long as needed.
Services (illustrated by Node.js and Go below) will listen for requests over a NATS server , a highly performant messaging system. NATS acts as service discovery (or rather makes it redundant), and enables near limit-less scaling. In essence, it is a simple, efficient, publish/subscribe message broker.
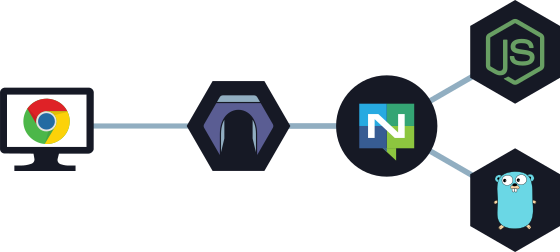
Writing a Service
You write a service pretty much as usual, but instead of listening for HTTP requests, the requests will come over NATS. However, you still have those desirable REST traits:
No framework requirements. No database requirements. No language requirements.
Below are two JavaScript (Node.js) snippets showing how to serve a resource, example.model
, using HTTP in comparison with using Resgate:
Using HTTP (with Express ):
var model = { message: "Hello, World!" }; // Listen to HTTP GET requests app.get('/example/model', (req, resp) => { resp.end(JSON.stringify(model)); });
Using Resgate (with NATS ):
var model = { message: "Hello, World!" }; // Listen to get requests over NATS nats.subscribe('get.example.model', (msg, reply) => { nats.publish(reply, JSON.stringify({ result: { model }})); });
Pretty similar, right?
And updating the resource is as simple as sending an event:
// Updating the model model.message = "Hello, Resgate!"; nats.publish('event.example.model.change', JSON.stringify({ values: { message: model.message } }));
That’s it!
Now, let’s take a look at the client-side.
Writing a Client
Fetching data from Resgate can either be done though HTTP, in which case it acts pretty much like an old school REST API:
GET /api/example/model
{ "message": "Hello, World!" }
Or it can be done using ResClient , a JavaScript library that gets both data and real-time updates through WebSocket:
let client = new ResClient('ws://api.example.com'); client.get('example.model').then(model => { console.log(model.message); // Hello, World! });
But when using ResClient, the data is updated in real-time!
let changeHandler = function() { console.log(model.message); // Hello, Resgate! } // Subscribe to changes model.on('change', changeHandler); // Unsubscribe to changes model.off('change', changeHandler);
No extra code is needed for updating the data. ResClient handles all that for you as the data is reliably synchronized despite disconnects, service restarts, and upgrades.
Summary
With Resgate you create REST and real-time APIs for the same effort of doing a REST API the traditional way. The main benefit is having your data synchronized seamlessly across your reactive web clients.
If you are interested in knowing more, visit the Resgate.io website. There you can find guides, examples, live demos, and more.
Note: Resgate and all related tools are released under the MIT license.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK