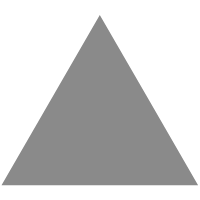
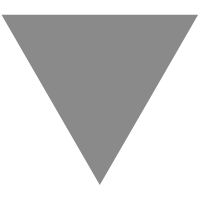
GitHub - kylebrowning/swift-nio-apns: An HTTP/2 APNS library built on swift-nio
source link: https://github.com/kylebrowning/swift-nio-apns
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
NIOApns
A non-blocking Swift module for sending remote Apple Push Notification requests to APNS built on http/2, SwiftNIO for use on server side swift platforms.
- Pitch discussion: Swift Server Forums
- Proposal: SSWG-0006
Installation
To install NIOSAPNS
, just add the package as a dependency in your Package.swift
dependencies: [ .package(url: "https://github.com/kylebrowning/swift-nio-http2-apns.git", .upToNextMinor(from: "0.1.0") ]
Getting Started
let group = MultiThreadedEventLoopGroup(numberOfThreads: 1) let apnsConfig = try APNSConfiguration(keyIdentifier: "9UC9ZLQ8YW", teamIdentifier: "ABBM6U9RM5", signingMode: .file("/Users/kylebrowning/Downloads/AuthKey_9UC9ZLQ8YW.p8"), topic: "com.grasscove.Fern", environment: .sandbox) let apns = try APNSConnection.connect(configuration: apnsConfig, on: group.next()).wait() let alert = Alert(title: "Hey There", subtitle: "Full moon sighting", body: "There was a full moon last night did you see it") let aps = APSPayload(alert: alert, badge: 1, sound: .normal("cow.wav")) let notification = APNSNotification(aps: aps) let res = try apns.send(notification, to: "de1d666223de85db0186f654852cc960551125ee841ca044fdf5ef6a4756a77e").wait() try apns.close().wait() try group.syncShutdownGracefully()
APNSConfiguration
APNSConfiguration
is a structure that provides the system with common configuration.
public struct APNSConfiguration { public var keyIdentifier: String public var teamIdentifier: String public var signingMode: SigningMode public var topic: String public var environment: APNSEnvironment public var tlsConfiguration: TLSConfiguration public var url: URL { switch environment { case .production: return URL(string: "https://api.push.apple.com")! case .sandbox: return URL(string: "https://api.development.push.apple.com")! } }
Example APNSConfiguration
let apnsConfig = try APNSConfiguration(keyIdentifier: "9UC9ZLQ8YW", teamIdentifier: "ABBM6U9RM5", signingMode: .file("/Users/kylebrowning/Downloads/AuthKey_9UC9ZLQ8YW.p8"), topic: "com.grasscove.Fern", environment: .sandbox)
SigningMode
SigningMode
provides a method by which engineers can choose how their certificates are signed. Since security is important we extracted this logic into three options. file
, data
, or custom
.
public enum SigningMode { case file(String) case data(Data) case custom(APNSSigner) } extension SigningMode { public func sign(digest: Data) throws -> Data { switch self { case .file(let filePath): return try FileSigner(url: URL(fileURLWithPath: filePath)).sign(digest: digest) case .data(let data): return try DataSigner(data: data).sign(digest: digest) case .custom(let signer): return try signer.sign(digest: digest) } } }
Example Custom SigningMode that uses AWS for private keystorage
public class CustomSigner: APNSSigner { public func sign(digest: Data) throws -> Data { return try AWSKeyStore.sign(digest: digest) } public func verify(digest: Data, signature: Data) -> Bool { // verification } } let customSigner = CustomSigner() let apnsConfig = APNSConfig(keyId: "9UC9ZLQ8YW", teamId: "ABBM6U9RM5", signingMode: .custom(customSigner), topic: "com.grasscove.Fern", env: .sandbox)
APNSConnection
APNSConnection
is a class with methods thats provides a wrapper to NIO's ClientBootstrap. The swift-nio-http2
dependency is utilized here. It also provides a function to send a notification to a specific device token string.
Example APNSConnection
let apnsConfig = ... let apns = try APNSConnection.connect(configuration: apnsConfig, on: group.next()).wait()
Alert
Alert
is the actual meta data of the push notification alert someone wishes to send. More details on the specifcs of each property are provided here. THey follow a 1-1 naming scheme listed in Apple's documentation
Example Alert
let alert = Alert(title: "Hey There", subtitle: "Full moon sighting", body: "There was a full moon last night did you see it")
APSPayload
APSPayload
is the meta data of the push notification. Things like the alert, badge count. More details on the specifcs of each property are provided here. THey follow a 1-1 naming scheme listed in Apple's documentation
Example APSPayload
let alert = ... let aps = APSPayload(alert: alert, badge: 1, sound: .normal("cow.wav"))
Custom Notification Data
Apple provides engineers with the ability to add custom payload data to each notification. In order to facilitate this we have the APNSNotification
.
Example
struct AcmeNotification: APNSNotification { let acme2: [String] let aps: APSPayload init(acme2: [String], aps: APSPayload) { self.acme2 = acme2 self.aps = aps } } let apns: APNSConnection: = ... let aps: APSPayload = ... let notification = AcmeNotification(acme2: ["bang", "whiz"], aps: aps) let res = try apns.send(notification, to: "de1d666223de85db0186f654852cc960551125ee841ca044fdf5ef6a4756a77e").wait()
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK