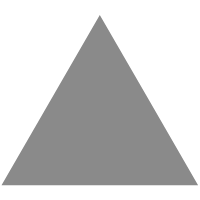
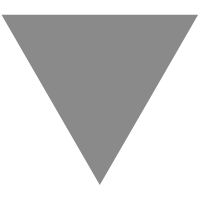
Getting Started With React Redux Using React
source link: https://www.tuicool.com/articles/hit/BJVfiea
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
We will create simple reactjs application and connect with react redux
. React is popular front end framework to create single page application.The Redux is a state management tool.It helps you write applications that behave consistently, run in different environments (client, server, and native).
React Reduxis the official React binding for Redux. It lets your React components read data from a Redux store, and dispatch actions to the store to update data.
We will add employee module into this React Redux Example Tutorial .we can add new employee into state and stored into store object. we can also fetch all employee data from store and display into html table.
Table of Contents
- Why Do We Need Redux?
-
Create React Application
- Install React Dependencies
- How To Connect Redux With React Application
-
Listing Component Integration With React Redux
- Create Smart Component For Employee List
What is Redux
The Redux use the state of your application is kept in a store and each component can access any state that it needs from this store.
The Redux has following three main concept –
Store Reducers Action
Why Do We Need Redux?
The main difficulties in single page application is the sharing of data between different component.The Redux solve this issue using store the application state.
The file structure would be like below –
-
src
app.js AddEmployee.js Employee.jsactions
index.js models.jsreducers
index.js empReducer.jscontainers
CreateEmployee.js EmployeeList.js
There are two type component exist in react redux integration. The Dumb component
, which is static and, Smart component
which have all logic and connected to store.
Create React Application
We will create react application using below command –
create-react-app react-redux-app-example
Above command will created react react-redux-app-example
application and install all basic dependencies.
Install React Dependencies
This react example have following external dependencies and, need to install into our application using npm.
The bootstrap 4 will use to list and create form for employee module.
npm install bootstrap --save
Added below entry into src/index.js
file.
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
Installed redux and react-redux package for Redux functionality.
npm install redux react-redux --save
Installed node-uuid
package for uuto incremented value for id field.
npm install node-uuid --save
How To Connect Redux With React Application
We will follow following steps to connect redux with react state.
We will create actions/models.js
file and added below code into this file –
export const ADD_EMPLOYEE = 'ADD_EMPLOYEE';
We will create actions/index.js
file and added below code into this file –
import { ADD_EMPLOYEE} from './models'; import {default as UUID} from "node-uuid"; export const createEmployee = ({ name, age, salary }) => ({ type: ADD_EMPLOYEE, payload: { id:UUID.v4(), name, age, salary } });
We will create reducers/empReducer.js
file and added below code into this file –
import { ADD_EMPLOYEE} from '../actions/models'; export default function empReducer(state = [], action) { switch (action.type) { case ADD_EMPLOYEE: return [...state, action.payload]; default: return state; } }
We will create reducers/index.js
file and added below code into this file –
import { combineReducers } from 'redux'; import emps from './empReducer'; export default combineReducers({ emps: emps });
Now, Create smart add employee component into containers folder.Let’s create container/CreateEmployee.js
and added below code –
import { connect } from 'react-redux'; import { createEmployee } from '../actions'; import AddEmployee from '../AddEmployee'; const mapDispatchToProps = dispatch => { return { onAddEmployee: emp => { dispatch(createEmployee(emp)); } }; }; export default connect( null, mapDispatchToProps )(AddEmployee);
Now, Create Dumb components into app/AddEmployee
folder, We will add below code into this file.
import React from 'react'; class AddEmployee extends React.Component { state = { name: '', age : 0, salary :0 } //handle input changes inputChange = e => { this.setState({[e.target.name] : e.target.value}); }; handleSubmit = e => { console.log(this.state); e.preventDefault(); console.log('fffff'); if(this.state.name.trim() !== '') { this.props.onAddEmployee(this.state); this.handleReset(); } }; handleReset = e => { this.state = { name: '', age : 0, salary :0 } }; render() { return ( <div> <form onSubmit={ this.handleSubmit }> <div className="form-group"> <label htmlFor="emaployeename">Employee Name</label> <input type="text" className="form-control" placeholder="Employee Name" className="form-control" name="name" onChange={ this.inputChange } value={ this.state.name }/> </div> <div className="form-group"> <label htmlFor="emaployeeAge">Employee Age</label> <input type="text" placeholder="Employee Age" className="form-control" name="age" onChange={ this.inputChange } value={ this.state.age } /> </div> <div className="form-group"> <label htmlFor="emaployeeSalary">Employee Salary</label> <input type="text" placeholder="Employee Salary" className="form-control" name="salary" onChange={this.inputChange} value={this.state.salary } /> </div> <div> <button type="submit" className="btn btn-primary ml-2">Add Employee</button> <button type="button" className="btn btn-warning ml-2" onClick={ this.handleReset }> Reset </button> </div> </form> </div> ); }; } export default AddEmployee;
Create handler method and static html form to get input from user.
Now, we need to import this AddEmployee.js
file inside src/App.js
file.
import React from 'react'; import CreateEmployee from './containers/CreateEmployee'; function App() { return ( <div className="container"> <div className="row"> <div className="col-md-6"> <CreateEmployee /> </div> </div> </div> </div> ); } export default App;
Let’s run this react redux tutorial using npm start, if every thing fine, you will see add employee form.
Listing Component Integration With React Redux
Already, integrated add employee features with this react redux tutorial.Now, we will display all employee list into home page.
Create Smart Component For Employee List
We will EmployeeList.js
file into components/
folder, this file will have employee data iteration logic and bind data with tr
element.
import React from 'react'; import { connect } from 'react-redux'; import Emp from '../Employee'; function EmployeeList({ emps, onDelete }) { console.log(emps); return ( <div> {emps.map(emp => { return ( <Emp emp={ emp } key={ emp.id } /> ); })} </div> ); } const mapStateToProps = state => { return { emps: state.emps }; }; export default connect( mapStateToProps, mapDispatchToProps )(EmployeeList);
We will create employee dumb component under app/Employee.js
folder.This file will have static data with html elements.
import React from 'react'; export default ({ emp: { id, name, age, salary }, onDelete }) => { return ( <tr key={id}> <td>{ name }</td> <td>{ age }</td> <td>{ salary }</td> </tr> ) };
Bind Employee List With Main Container
We will call employee list component into main app.js
container.
... import EmpList from './containers/EmployeeList'; function App() { return ( ..... <table className="table table-bordered"> <thead> <tr> <th scope="col">Name</th> <th scope="col">Age</th> <th scope="col">Salary</th> </tr> </thead> <tbody> <EmpList/> </tbody> </table> ... ); } export default App;
Save the file and go to the http://localhost:3000/
If everything configured and coded fine then we can be able to add and display the employee.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK