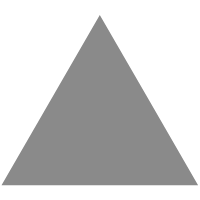
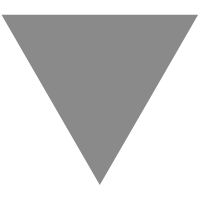
7 adorable web development tricks
source link: https://www.tuicool.com/articles/hit/Rv67NvA
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
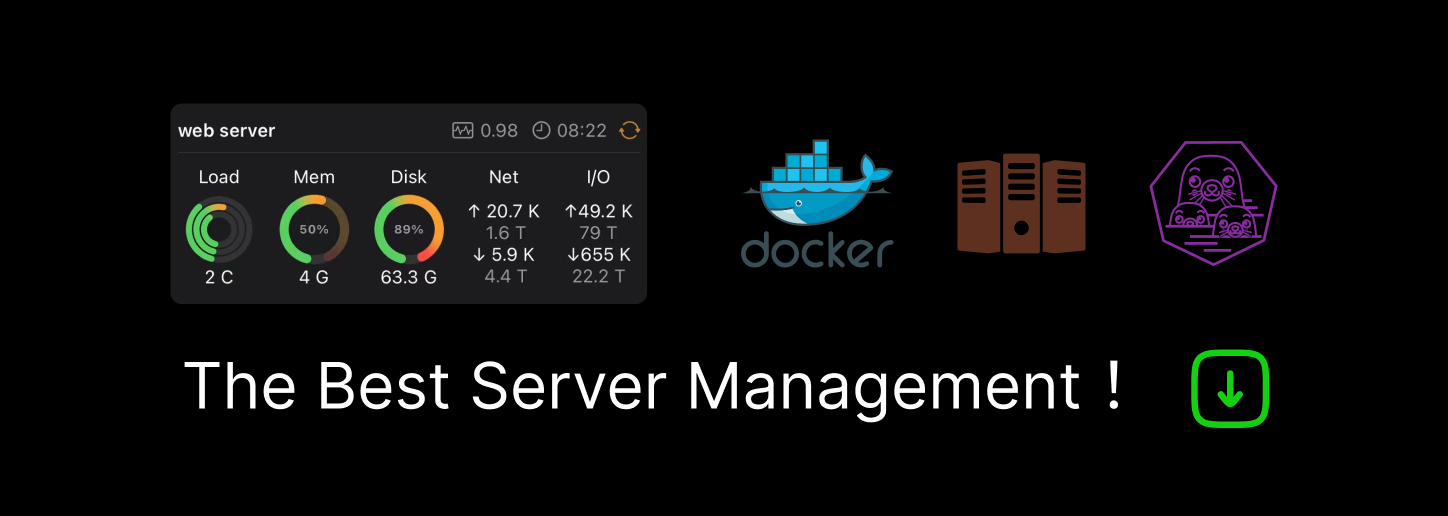
By now, all of major web development languages can be considered as matured . With more than 2 decades of development each, HTML , CSS and JavaScript are globally-respected standards of the web. But, that's just a mere fact leading us to the main topic of this post. Today, I'd like to demonstrate to you 7 interesting and lesser-known tips / tricks , that these 3 languages have developed over the years. Believe me or not - there's some stuff that even more-experienced web developers may not know about. Maybe it's just because not everything is equally useful... Anyway, let's dig deep and have some fun!
7. Boolean convertion
Type-safety and JavaScript can seem like two completely different concepts. Keeping track of all dynamic types in JS can be quite a hard task. Yet still, it can result in better performance, with the written code being easier to process by the JIT compiler. Using types other than boolean in e.g. conditional expressions is a common example of such mistakes. But, there's a trick for that!
Remember
logical NOT
operator ( !
)? It is a simple, fast and elegant way of converting given value to opposite boolean value. But what if we want our boolean to represent exactly the value that it would be (when represented as boolean)? Well... we've already got the opposite boolean, right? So, let's negate our value yet again and have full-fledged boolean value
... or just use the Boolean()
function from the start .
const falsyValue = 0; const truthyValue = 1; !!falsyValue; // false !!truthyValue; // true
6. Divide & round
There are quite a few operators in JS. Some of them are used extensively, while others are not. The second group often includes what's known as bitwise operators . They basically operate on individual bits ( binary numbers ) instead of any other numeric system. Many JS programmers know of their existence but don't really bother to use them. That's mainly because they might feel a little bit complex, to say the least, aren't really beginner-friendly and thinking them out can take a while.
But, bitwise operators have their advantages too! They surely allow the programmer to write the same code with shorter syntax , but only in special cases. Other than that, the fact that they operate on bits makes them, naturally, more performant solution . To give you a glimpse of what I mean, here's an example of dividing the number by 2 and rounding (flooring) the result VS the same operation with basic right shift operator.
const value = 543; const halfValue = value/2; // 271.5 Math.round(halfValue); // 272 Math.floor(halfValue); // 271 value >> 2; // 271
5. JSON formatting
Concept of JSON
is most probably known by all JS developers. It's something that's introduced right at the beginning of one's JS journey. Thus, the same applies for JSON.parse()
and JSON.stringify()
methods. As you surely know, they allow you to convert your JSON-compatible values to strings back-and-forth. But, the one trick that I bet you most likely didn't know, is the fact that with JSON.stringify()
, you can actually format your output
string.
The method, aside the value to be stringified, takes optional 2 arguments:
-
replacer
- function or an array of strings or numbers that are later used to whitelist the properties of passed value to later include them in the result string. When equal tonull
, and by default, it just takes all the properties in. -
spaces
- a number or a string with value and length limited to 10 respectively. It gives you an option to set the string or number of white spaces that you want to use to separate your object's properties inside the output string. If it's equal to 0, empty string ornull
(default), the output is left untouched.
Now, especially the second argument gives you a nice and simple way of prettifying your stringified values. Of course, it's by all means not the best solution for every problem, but at least it's there, ready to be used at any time.
const obj = {a:{b:1,c:2},d:{e:3,f:4}}; JSON.stringify(obj); // "{"a":{"b":1,"c":2},"d":{"e":3,"f":4}}" JSON.stringify(obj, null, 2); /* "{ "a": { "b": 1, "c": 2 }, "d": { "e": 3, "f": 4 } }" */
4. CSS centering
Centering elementswith CSS isn't a trick per se. In fact, it's a very common practice. But, the reality is, some developers (including me) often forget such simple things. And, to make matter worse, there's no the best and only way to this problem. That's because of incompatibilities across different browsers (especially IE).
Definitely one of the most wide-spread solutions that achieved large adoption is the use of Flexbox model . Below is an example of centering and aligning element's child right in the parent's center.
<div style="display:flex;justify-content:center;align-items:center;"> <div></div> </div>
Beyond the method above ( it doesn't have good support across different versions of IE ), I highly recommend you to check out the How to Center in CSS website, where you will be provided with the proper solution for the given case.
3. CSS variables
In the era of CSS preprocessors, web frameworks, and CSS-in-JS solution, the usage of plain CSS has definitely seen at least a small decline. That's not really a bad thing - as long as the listed solutions are doing better job. But, one feature that CSS preprocessors were well-known for, and recently landed into the vanilla CSS is variables !
:root { --main-bg-color: brown; } .className { background-color: var(--main-bg-color); display: block; color: white; }
The browser support for this feature looks quite good too! Anyway, it's good to know that some so-desired features are making their ways to the language itself. Yet, it doesn't match the versatility of any preprocessor or CSS-in-JS library. But still, it's good to know such a feature exists.
2. CSS support checks
Support for different features both in JS and CSS greatly varies across the browsers. And, while feature-checks
on the JS side aren't really that complex, things are different when it comes to CSS... or rather were. Introducing the @supports
CSS rule - the best solution for feature checks.
@supports (display: grid) { div { display: grid; } } @supports not (display: grid) { div { display: block; } }
Its whole syntax has a form of if-else statements
in which you can check whether given property-value pair is supported. All in all, it's just a nice solution for checking for support of different features, but only if @supports
itself is... supported
.
1. Styled styles
Now, for the number 1 trick, I clearly have to give the proper attribution to the source's author - @calebporzio .
Cool thing I just found out is possible in HTML. No practical use, just fun. pic.twitter.com/4zqQBMm46Z
— Caleb Porzio (@calebporzio) April 24, 2019
It basically all comes down to the fact, that you can style the style element, display its content, make it editable and viola! - you've got a somewhat live CSS editor
! As the author says - it may not have any actual use case but it's just awesome! Also, it sadly doesn't work the same way with the <script>
tag.
<style style="display: block" contenteditable> html { background: blue; } </style>
Useful?
So, what do you think of this short, but quite an interesting list? Do you like the picks? Have you already known some of them? Share your thoughts in the comment section and with a reaction below. Also, consider sharing the article itself! To stay up-to-date with the latest content from this blog, follow me on Twitter , on my Facebook page and sign up for the weekly newsletter . As always, thank you for reading and have a great day !
Recommend
-
41
turnoff.us is a geek comic site. Comics about Programming Languages, Web, Cloud, Linux, etc.
-
53
README.md
-
64
go-echarts :art: The adorable charts library for Golang. 如果一门语言可以用来写爬虫,那么它就需要...
-
86
README.md cast.sh
-
19
Ninja has his own adorable costume in 'Fall Guys'Ninja has his own adorable costume in 'Fall Guys'Jon Fing...
-
10
Volkswagen is developing an adorable EV charging robot It can travel around parking garages autonomously By
-
28
Review: AKKO World Tour Tokyo mechanical keyboard is as functional as it is adorable
-
4
Review: Sun Haven is an adorable farming sim for fantasy fans Grade: "I" for incomplete
-
7
bookmarklet 6 Useful Bookmarklets to Boost Web Development DigitalOcean joining forces with...
-
6
Right after “Where is the best place to learn?” perhaps the most commonly asked question I hear from folks getting into code is “What web development books should I get to learn?” Well, consider this an answer to that question as I’ve curated a li...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK