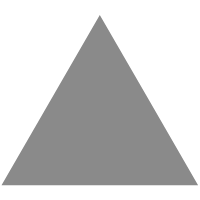
52
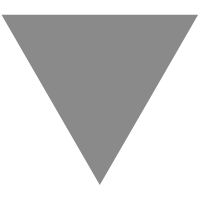
与go邂逅(二)——go当中的基本程序结构
source link: https://studygolang.com/articles/20042?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
前言
学习一门语言的时候,难免从最简单的程序结构学起,这些东西在掌握了一门别的开发语言的情况(如大名鼎鼎的java),就会显得如鱼得水了,下面会把我学习一些简单例子分享出来。
基本程序结构
快速为一些变量赋值
const ( NUM1 = 1 + iota NUM2 NUM3 NUM4 ) //输出结果:1,2,4,8 func TestPrint(t *testing.T) { t.Log(NUM1, NUM2, NUM3, NUM4) }
快速的实现一些数值交换
//数值交换 func TestExchange(t *testing.T) { //也可以这样定义变量:var aa int = 1 a := 1 b := 2 t.Log(a, b) //交换数值 b, a = a, b t.Log(a, b) }
类型转换
//给类型命名 type typeInt int64 func TestInt(t *testing.T) { var a int64 = 2 var b int32 = 3 //类型不可转 //a = b var c = typeInt(3) t.Log(a, b, c) }
实现斐波拉切数列的两种方式
//斐波拉切 func TestFibList(t *testing.T) { var a int = 1 var b int = 1 t.Log(a) for i := 0; i < 5; i++ { t.Log(b) tmp := a + b a = b b = tmp } } //斐波拉切 递归 func TestFibRecursion(t *testing.T) { t.Log(FibRecursion(5)) } func FibRecursion(i int) (result int) { if i == 1 || i == 2 { return 1 } return FibRecursion(i-1) + FibRecursion(i-2) }
数组比较,和java不同,不是比较指针,可以比较值的
//数组比较 func TestCompareArray(t *testing.T) { a := [...]int{1, 2, 3, 4} b := [...]int{1, 2, 2, 4} c := [...]int{1, 2, 3, 4} t.Log(a == b) //false t.Log(a == c) //true }
go也是有指针的,但是没有细看,只是写个例子看下结果
func TestPoint(t *testing.T) { var a int64 = 1 var aPtr = &a t.Log(a, aPtr)// 1 0xc420018230 //打印类: int64 *int64 t.Logf("%T %T", a, aPtr) }
string的默认值
func TestString(t *testing.T) { //默认值是"" 不是java的那种null var str string t.Log("+" + str + "+")//输出++ }
for循环
//for循环 go当中原来没有while func TestFor(t *testing.T) { n := 5 for n > 0 { t.Log(n) n-- } } //for循环实现冒泡排序 func TestForSort(t *testing.T) { a := [...]int{3, 5, 2, 6, 4, 8, 2, 9,1,23,2,34,4,55,11} for i := 0; i < len(a)-1; i++ { for j := 0; j < len(a)-i-1; j++ { if a[j] > a[j+1] { tmp := a[j] a[j] = a[j+1] a[j+1] = tmp } } } t.Log(a)//[1 2 2 2 3 4 4 5 6 8 9 11 23 34 55] }
go当中的条件判断,写起来还是很爽的
//比较 func TestCondition(t *testing.T){ //可以条件结果赋值给变量 if a:=3>2;a{ t.Log("3>2") } // GOOS is the running program's operating system target: // one of darwin, freebsd, linux, and so on. switch runtime.GOOS{ //自带break case "darwin": t.Log("darwin") case "freebsd": t.Log("freebsd") case "linux": t.Log("linux") default: t.Log("default") } switch { case 4>2: t.Log("4>2") case 4<2: t.Log("4<2") } }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK