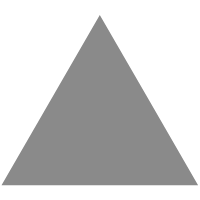
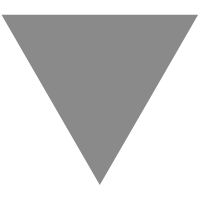
Single File Source Code With Java 11
source link: https://www.tuicool.com/articles/hit/nARRZzn
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
One of the many salient features of Java 11 is the ability to directly run a single file of Java source code without the need to compile the source code first. Complementing JShell , this feature helps in learning Java and in writing simple utility applications and CLIs.
Say you created a HelloWorld.java file that, you guessed it, prints "Hello World:"
public class HelloWorld { public static void main (String... args) { System.out.println("Hello World"); } }
With JDK 11 installed, you can run this file using the "java" launcher:
java HelloWorld.java
The "java" launcher in JDK 11 has been updated to operate in four modes:
- Launch a class file
- Launch the main class of a jar
- Launch the main class of a module
- Launch the class declared in a single source file
The "java" launcher identifies the single file mode when one of the following two conditions match:
- The first item passed to it is the class file with the ".java" extension
- The "--source" option is specified
Shebang Support
As mentioned earlier, the single file mode can be useful to create small utility programs. The Shebang ("#!") mechanism available on Unix based systems is a perfect fit to run such single file utility programs. A shebang file to invoke the Java launcher using single file mode should begin with:
#!/path/to/java --source version
The shebang line can include other launcher options like adding additional classpath jars. Of course, the file should be marked as executable. Note that when you include the shebang line, your single file source code name need not follow the Java class naming conventions. For example, the HelloWorld.java can be renamed to "hello".
Shebang support is not natively available in Windows
Equipped with this knowledge, let’s create a small program that runs Tomcat as an Embedded (TED) server. Download the embedded tomcat zip file and unzip the required jars into a "lib" folder. Next, create a file named "ted" and mark it as executable:
touch ted && \ chmod +x ted
Add the following code to the file:
#!/usr/bin/java --class-path ./lib/* --source 11 import org.apache.catalina.Wrapper; import org.apache.catalina.startup.Tomcat; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.File; import java.io.IOException; public class TomcatEmbedded { private static final String EMPTY = ""; public static void main(String... args) throws Exception { File baseFolder = new File(System.getProperty("user.dir")); File appsFolder = new File(baseFolder, "apps"); Tomcat tomcat = new Tomcat(); tomcat.setBaseDir(baseFolder.getAbsolutePath()); tomcat.setPort(8080); tomcat.getHost().setAppBase(appsFolder.getAbsolutePath()); // Call the connector to create the default connector. tomcat.getConnector(); tomcat.addWebapp(EMPTY, "."); Wrapper wrapper = tomcat.addServlet(EMPTY, "hello", new HelloServlet()); wrapper.setLoadOnStartup(1); wrapper.addMapping("/*"); tomcat.start(); tomcat.getServer().await(); } private static class HelloServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { resp.setStatus(200); var writer = resp.getWriter(); writer.write("Hello World"); writer.flush(); writer.close(); } } }
Save the file and run the file as a regular executable:
./ted
Navigate to http://localhost:8080 in your browser to see the utility program in action. Granted, "ted" isn’t useful in its current incarnation but one can extend it to build a small developer "Dropbox" utility program to share files across VMs.
The possibilities to create self-contained utility programs in Java are endless with this new single file source code feature.
Happy coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK