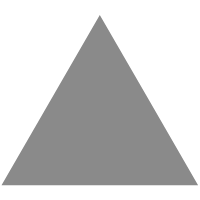
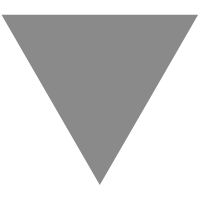
Test HTTP Requests without Mock Server
source link: https://www.tuicool.com/articles/hit/EZJVfmI
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
HTTP client that provides object-oriented interface for building HTTP requests. The benefits of this library are that it is easily customizable, declarative and immutable. It can also bewithout mock servers which gives a performance edge.
Similar libraries: cactoos-http , jcabi-http
<dependency> <groupId>hr.com.vgv.verano</groupId> <artifactId>verano-http</artifactId> <version>1.0</version> </dependency>
Get a Url
JsonObject json = new JsonBody.Of( new Response( "http://example.com", new Get( "/items", new QueryParam("name", "John"), new Accept("application/json") ) ) ).json();
Post to a Server
new Response( "http://example.com", new Post( "/items", new Body("Hello World!"), new ContentType("text/html"), ) ).touch();
Touch
method executes the HTTP
request towards the server.
Using form parameters:
new Response( "http://example.com", new Post( "/items", new FormParam("name","John"), new FormParam("foo","bar"), ) ).touch();
Response handling
Extraction of response parameters can be done using *.Of
classes:
Response response = new Response( "http://example.com", new Get("/items") ); Map<String, List<String>> headers = new Headers.Of(response).asMap(); // Extraction of headers from response String cookie = new Cookie.Of("cookieName", response).asString(); String body = new Body.Of(response).asString();
You can make assertions on received responses like this:
new Response( "http://exmpl.com", new Get("/items"), new ExpectedStatus( 200, new FailWith("Cannot fetch from exmpl") ) ).touch();
Serialization and Deserialization
The library provides following types of request body serialization:
Response body deserialization can be achieved using their accompanied *.Of
classes.
Wire
Verano-http runs on ApacheWire
which encapsulates Apache http client . You can provide additional configuration to the wire:
Wire wire = new ApacheWire( "http://exmpl.com", new Proxy("127.0.0.1", 8000), new SslTrusted() ); new Response( wire, new Get("/items") ).touch();
You can also provide http parameters to the wire:
new Response( new ApacheWire( "http://exmpl.com", new ContentType("application/json"), new Header("foo", "bar"), ), new Get("/items") ).touch();
Testing
Http requests can be tested through MockWire
without using a http server. MockWire
works in conjunction with hamcrest matchers in a following way:
MockWire wire = new MockWire( new MockAnswer( new PathMatch(MatchesPattern.matchesPattern("/*")), new Response(new Status(201)) ) ); sendRequest(wire); wire.verify( new PostMatch( new PathMatch(new IsEqual<>("/items")), new BodyMatch(new StringContains("text")) ) );
Contribution
You can contribute by forking the repo and sending a pull request. Make sure your branch builds without any warnings/issues:
mvn clean install -Pqulice
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK