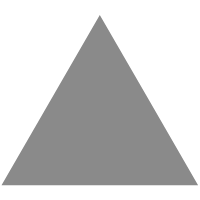
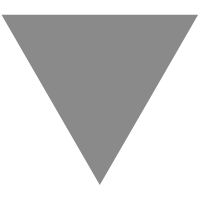
GitHub - gcla/gowid: Compositional widgets for terminal user interfaces, written...
source link: https://github.com/gcla/gowid
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Terminal User Interface Widgets in Go
Gowid provides widgets and a framework for making terminal user interfaces. It's written in Go and inspired by urwid.
Widgets out-of-the-box include:
- input components like button, checkbox and an editable text field with support for passwords
- layout components for arranging widgets in columns, rows and a grid
- structured components - a tree, an infinite list and a table
- pre-canned widgets - a progress bar, a modal dialog, a bar graph and a menu
- a VT220-compatible terminal widget, heavily cribbed from urwid ?
All widgets support interaction with the mouse when the terminal allows.
Gowid is built on top of the fantastic tcell package.
There are many alternatives to gowid - see Similar Projects
Installation
go get github.com/gcla/gowid/...
Examples
Make sure $GOPATH/bin
is in your PATH (or ~/go/bin
if GOPATH
isn't set), then tab complete "gowid-" e.g.
gowid-fib
Here is a port of urwid's palette example:
Here is urwid's graph example:
And urwid's fibonacci example:
Finally a demonstration of gowid's terminal widget, a port of urwid's terminal widget:
Hello World
This example is an attempt to mimic urwid's "Hello World" example.
package main import ( "github.com/gcla/gowid" "github.com/gcla/gowid/widgets/divider" "github.com/gcla/gowid/widgets/pile" "github.com/gcla/gowid/widgets/styled" "github.com/gcla/gowid/widgets/text" "github.com/gcla/gowid/widgets/vpadding" ) //====================================================================== func main() { palette := gowid.Palette{ "banner": gowid.MakePaletteEntry(gowid.ColorWhite, gowid.MakeRGBColor("#60d")), "streak": gowid.MakePaletteEntry(gowid.ColorNone, gowid.MakeRGBColor("#60a")), "inside": gowid.MakePaletteEntry(gowid.ColorNone, gowid.MakeRGBColor("#808")), "outside": gowid.MakePaletteEntry(gowid.ColorNone, gowid.MakeRGBColor("#a06")), "bg": gowid.MakePaletteEntry(gowid.ColorNone, gowid.MakeRGBColor("#d06")), } div := divider.NewBlank() outside := styled.New(div, gowid.MakePaletteRef("outside")) inside := styled.New(div, gowid.MakePaletteRef("inside")) helloworld := styled.New( text.NewFromContentExt( text.NewContent([]text.TextContentSegment{ text.StyledContent("Hello World", gowid.MakePaletteRef("banner")), }), text.Options{ Align: gowid.HAlignMiddle{}, }, ), gowid.MakePaletteRef("streak"), ) f := gowid.RenderFlow{} view := styled.New( vpadding.New( pile.New([]gowid.IContainerWidget{ &gowid.ContainerWidget{IWidget: outside, D: f}, &gowid.ContainerWidget{IWidget: inside, D: f}, &gowid.ContainerWidget{IWidget: helloworld, D: f}, &gowid.ContainerWidget{IWidget: inside, D: f}, &gowid.ContainerWidget{IWidget: outside, D: f}, }), gowid.VAlignMiddle{}, f), gowid.MakePaletteRef("bg"), ) app, _ := gowid.NewApp(gowid.AppArgs{ View: view, Palette: &palette, }) app.SimpleMainLoop() }
Running the example above displays this:
Documentation
Similar Projects
Gowid is late to the TUI party. There are many options from which to choose - please read https://appliedgo.net/tui/ for a nice summary for the Go language. Here is a selection:
- urwid - one of the oldest, for those working in python
- tview - active, polished, concise, lots of widgets, Go
- termui - focus on graphing and dataviz, Go
- gocui - focus on layout, good input options, mouse support, Go
- clui - active, many widgets, mouse support, Go
- tui-go - QT-inspired, experimental, nice examples, Go
Dependencies
Gowid depends on these great open-source packages:
- urwid - not a Go-dependency, but the model for most of gowid's design
- tcell - a cell based view for text terminals, like xterm, inspired by termbox
- asciigraph - lightweight ASCII line-graphs for Go
- logrus - structured pluggable logging for Go
- testify - tools for testifying that your code will behave as you intend
Contact
- The author - Graham Clark ([email protected])
License
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK