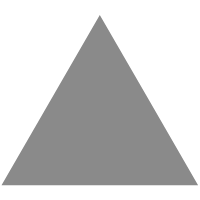
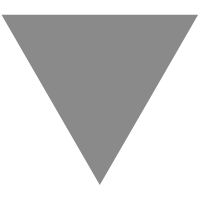
Making Animations In React Native— The Simplified Guide
source link: https://www.tuicool.com/articles/hit/q2M36fq
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Now, what are we gonna is create animations like Fade
, slideUp
, slideDown
, rotate
..etc. using the animations API .
A good to mention we will be comparing the examples we are going to create with rnal (react native animations library) the library I’ve created a library that does that all the kind of the animations you may want use. learn more here !
First, of all to start using animations, we are using Animated
module that react-native provides to do the magic things for us :dizzy:
When building components, I’d advise using Bit to share and manage them across projects as a team- so you can build faster and stay in sync. Try it:


LayoutAnimation !!!
import {Animated} from "react-native";
Let’s start first with Fade
:
Fade animation


The Animated
method takes parameters as values and turns those values into animated values then we can use those values to animate our Components, okay! show me the code! :

Let’s break it down!
First, we need to initialize a value so we can give this value to the Animated
method :
state = {
fadeValue: Animated.Value(0)
};
Then let’s create a function called _start
that will start our animations:
_start = () => {
return Animated.timing(this.state.fadeValue, {
toValue: 1, // output
duration: 3000, // duration of the animation
}).start();
};
What we just did here? we used timing
method from Animated
, it takes some values:
start()
Now we have an animated value, the next step is to use it to animate the component. we use Animated.View
as a wrapper to our component in order to make the animations happen !
<Animated.View
style={{
opacity: this.state.fadeValue,
height: 250,
width: 200,
margin: 5,
borderRadius: 12,
backgroundColor: "#347a2a",
justifyContent: "center"
}}
>
<Text style={styles.text}>Fade </Text>
</Animated.View>
You see we passed the fadeValue
to the opacity property so we can handle the opacity of the element so when the component start appears it happens smoothly and in an animated behavior! and that gives us the fade
animation !
Now let's call the _start()
function when an action is taken , in our case we call the function when button
is clicked. you can trigger the function whenever you like for example within componentDidMount()
.
<TouchableOpacity style={styles.btn} onPress={() => this._start()}>
Doing the Fade animation with rnal :
rnal made things for us more easy to create the fade animation:
First, let’s install the package :
With Yarn
hit yarn add rnal
, or npm
run npm i rnal
Then Import The Fade
element :

And:


Fade has startWhen
props, it’s a type of boolean
and it determines when the animation should start by default it starts when the component mount, you can discover more options to customize your animations, check out the docs .
Let’s try with something more awesome like making the component goes from the bottom or SlideDown
animation. we will use the same code above but we need to change some values! and we will use interpolate
method to interpolate the AnimatedValue cool let’s do it :tophat:
SlideDown animation

Replace gif with the slideDown animation


The interpolate
method takes an Object of properties.
-
inputRange
: The start point of theanimatedValue
: for example, we want thetranslateX
to start from 1 level :
inputRange:[0,1]
-
outputRange
: gives us an outputRange based on theinputRange
transform:[
{translateY:animatedValue.interpolate({
inputRange:[0,1],
outputRange:[-600,0]})} ]
Doing the same with react-native-animations-library ! is more much simple, there is the SlideDown
element so you can wrap the component you want to animate!

There are cases when need to make the animations to run indefinitely, Animated gives us Animated.loop()
method to make this happen let’s see how it works! the best example to demonstrate that is to create a spinner!

Here we go!


What we just did is give the Animated.loop
our animations as an argument to run it in a loop cycle.
With react-native animation library(rnal) , you can just add inifinite
props to make this happen!
import { Rotate } from "rnal";
<Rotate infinite>
<View
style={{
height: 50,
width: 50,
margin: 5,
borderWidth: 2,
borderColor: "#888",
borderBottomColor: "#8bffff",
borderRadius: 50,
justifyContent: "center"
}}
/>
<Text style={styles.text}>Fade </Text>
<View />
</Rotate>
If you take a look at the examples above, we don’t actually change much, the same function we just determine where we use then animatedValue
yeah it’s all about a value transformed to an animated value and allow us to do the animation we want using translate,scale, rotate
and all other supported animations :stuck_out_tongue: .
That was the way we run a single animation what about making a group of animations? sequence
and parallel
methods got you covred!
Run a group of animations
We can use sequence
or parallel
methods to run a group of animations, the two methods do almost the same job, the only difference is that parallel run the group of animations at once whereas the sequence
method blocks the next animation until the previous animation is finished and done, and if one of the animations stops or break the next animation won’t be able to run. you can explore more about the two methods in the official docs .

- Using
parallel
method:


With sequence
:


I think we are gonna stop at this point we don’t want to make this post longer. generally, there is much to talk about React Native animations and it’s a huge subject we won’t be able to cover it in one post we will have a chance to talk about other parts in upcoming articles but anyway we just covered the most parts of it and you will be able to create your own animations after reading this article. you can always use the react-native animations library I’ve created it’s so simple and easy to use. Thanks for reading, and please comment below!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK