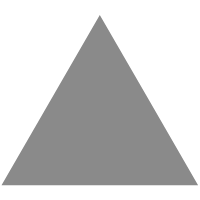
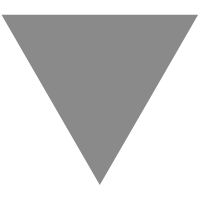
JavaScript Notifications Tutorial
source link: https://www.tuicool.com/articles/hit/ye2ANvq
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
JavaScript Notifications

JavaScript Notifications are a way to show messages to the user on their computer, even if they are busy doing something else.
They can be used to notify someone that they have a new message from someone, such as an email, social media or chat message. They can also be used for calendar reminders. Notifications can also be used in annoying ways, for example for marketing or site updates that people probably don’t care about too much.
With notifications, the end user is in control. They must opt-in to receive notifications from your site, and they can turn off notifications at any time. In this tutorial, we will cover the basic parts of the Notifications API which will let you send notifications while your site is open in a browser tab (even if it isn’t the current one) and even if the browser is hidden behind other windows.
There is a more advanced usage of the Notifications API that allows you to push messages to users who are not currently visiting your site. That is quite complex to set up and often website owners will subscribe to services to do this for them. If you are interested Google has a tutorial on this here: https://developers.google.com/web/fundamentals/codelabs/push-notifications/
The first thing to know about notifications is that it is quite a modern feature and you might have some visitors to a site with an older browser that doesn’t support them. Therefore it is good to check if notifications are supported, and you can do that by checking if the window
object has a property called Notification
:
if (!("Notification" in window)) { // Code to run if notifications are not // supported by the visitor's browser } else { // Code to run otherwise }
Next, we want to check if we have specific permission from the visitor to show notifications. If we do then we can show a notification my simply construction a Notification
object:
if (!("Notification" in window)) { // Code to run if notifications are not // supported by the visitor's browser } else { if (Notification.permission === "granted") { var notification = new Notification("This is an important message."); } }
If permission hasn’t been granted there are two possible reasons:
- The user hasn’t yet chosen to accept notifications from your site.
- The user has chosen to block notifications from your site.
If it is the first case, then you can request the user to make that choice. If it is the second, we can’t do anything because they have blocked us, and so their browser will ignore any requests to ask for permissions.
Here is the code updated to ask for permissions if the user hasn’t already denied them:
Notification.requestPermission().then(function (permission) { if (permission === "granted") { var notification = new Notification("This is an important message."); } });
Here we call Notification.requestPermission()
which will ask the user for permissions. Because there could be a wait for this to happen, the method returns apromise which means we can supply a function that will be called once the decision has been made.
Because it is a promise, we will pass this callback function to then()
and if they decide to grant permission we then make a new notification.
Putting this all together, we can create a function that will try to show a notification message, requesting permission if necessary:
function showMessage(message) { if (!("Notification" in window)) { // Code to run if notifications are not // supported by the visitor's browser } else { if (Notification.permission === "granted") { var notification = new Notification(message); } else if (Notification.permission !== "denied") { Notification.requestPermission().then(function (permission) { if (permission === "granted") { var notification = new Notification(message); } }); } } } showMessage("This is an important message.");
It is fine to copy the function above as-is into your JavaScript file and then just use showMessage
whenever you please. This way the first time any message from anywhere in your JavaScript needs to show, it will ask the user for permission, and then after, if granted it will just show the message(s).
Summary
This is a quick intro to the simpler way of using notifications in JavaScript. The simpler way requires your web page to be open for the user to see the notifications. The more complicated way requires push notifications and there is a link to a Google page about that in this article.
Recommend
-
36
Update Note : Keegan Rush updated this tutorial for Xcode 10 and Swift 4.2. Jack Wu wrote the original tutorial. iOS developers love to imagine users using their awesome app constantly. But, of course...
-
15
If you’ve used a mobile device for the last decade, you’ve likely encountered innumerable of push notifications . Push notifications allow apps to broadcast alerts to users — even if they’re not actively using t...
-
3
Push Notifications and Local Notifications - Tutorial T...
-
6
The final code for this tutorial can be found
-
3
The Flask Mega-Tutorial, Part XXI: User Notifications Posted by on under...
-
17
A minimalist JavaScript library for toast notifications.NotyfA minimalistic JavaScript library for toast notifications. Responsive, A11Y, dependency-free. Tiny (~3KB).Easy integration with React, Angular and...
-
24
ToastMaker Toast Maker is a simple and very lightweight javascript library for showing toast notification messages on web page. It provides multiple configurations to customize the toast styling(font, ba...
-
2
Disclaimer: What the title means by 'in less than 10 lines of code' is referring to the number of lines of JavaScript code. You know those annoying notifications popping up at the least...
-
1
Display Desktop Notifications Using JavaScriptDisplay Desktop Notifications Using JavaScriptOctober 26th 2022 New Story5...
-
111
#StopRussianAggresion - support Ukraine! Fractal animation You can code this colorful, real-time animated fractal in only 32 lines of Javascript code! 1. The fractal We'll use the Julia fractal. If you're not inter...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK