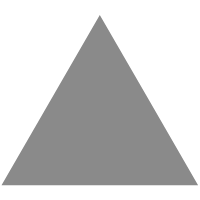
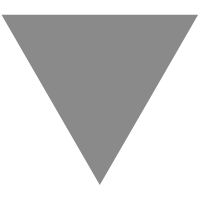
Algorithms & Data Structures Explained and Implemented in JavaScript
source link: https://www.tuicool.com/articles/hit/3mQRJrY
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Data Structures and Algorithms in JavaScript
This repository covers the implementation of the classical algorithms and data structures in JavaScript.
Usage
You can clone the repo or install the code from NPM:
npm install dsa.js
and then you can import it into your programs or CLI
const { LinkedList, Queue, Stack } = require('dsa.js');
For a full list of all the exposed data structures and algorithms see .
Book
You can check out the dsa.js book that goes deeper into each topic and provide additional illustrations and explanations.
- Algorithmic toolbox to avoid getting stuck while coding.
- Explains data structures similarities and differences.
- Algorithm analysis fundamentals (Big O notation, Time/Space complexity) and examples.
- Time/space complexity cheatsheet.
The book text is available to read here:
Data Structures
We are covering the following data structures.
Linear Data Structures
-
Arrays: Built-in in most languages so not implemented here. Post .
-
Linked Lists: each data node has a link to the next (and previous). Code | Post .
-
Queue: data flows in a "first-in, first-out" (FIFO) manner. Code | Post
-
Stacks: data flows in a "last-in, first-out" (LIFO) manner. Code | Post .
Non-Linear Data Structures
-
Trees: data nodes has zero or more adjacent nodes a.k.a. children. Each node can only have one parent node otherwise is a graph not a tree. Code | Post
-
Binary Trees: same as tree but only can have two children at most. Code | Post
-
Binary Search Trees(BST): same as binary tree, but the nodes value keep this order
left < parent < rigth
. Code | Post -
AVL Trees: Self-balanced BST to maximize look up time. Code | Post
-
Red-Black Trees: Self-balanced BST more loose than AVL to maximize insertion speed. Code
-
-
Maps: key-value store.
-
Graphs: data nodes that can have a connection or edge to zero or more adjacent nodes. Unlike trees, nodes can have multiple parents, loops. Code | Post
Algorithms
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK