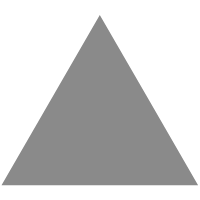
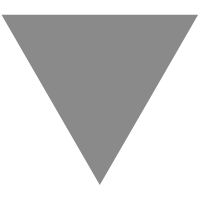
Using React Hooks To Create Awesome Forms
source link: https://www.tuicool.com/articles/hit/JziuuuE
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Using React Hooks To Create Awesome Forms
Learn How To Build Custom React Hooks and Use Them to Create Great Forms


In one of my previous posts, I showed you how to use React’s latest feature that everyone is talking about — React Hooks !
If you have read this post, then you now know how to use the useState , useEffect , and the useContext hooks in your React App.
But real-world apps are not going to be so simple. Eventually, you will need to figure out how to use these hooks together.
When you use two or more basic hooks together, you are creating something called as a custom hook !
In this post, I will show you how to create and use a custom hook in your React App by building something that you have used almost every day in your life! A Form.
Forms are something that are considered easy to build. If you have worked in html, then all you need to do is create a form
tag and add a few input
tags and a submit
type button, and voila! You have a form ready!
<form> <label>Username</label> <input type="text"/> <label> Passworld</label> <input type="password"/> <button type="password">Login</button> </form>
If you create this form, nothing is going to happen. Creating forms is easy, but creating useful forms is difficult.
Let’s start by creating a simple signup form in React and see how we can make it better using React Hooks.
If you would check out the entire source code for this post, then click here:
Getting Started
To start, let’s create a new React App project directory in your system. Normally this is where I ask you to install the create-react-app
CLI in your system and use it to build the empty React project. But I have recently fallen in love with the npx
utility tool that comes with the Node.JS library. With npx
, you can use the latest version of React to build the project, without having to install the latest version in your system.
So go to your command terminal and write the following command to build a React project named hooks-form
.
$ npx create-react-app hooks-form
If you run the yarn start
command in your terminal, then you will get this in your browser.


But we don’t need any of this. So let’s clean up our files in the code editor (I :heart: VS Code ). To be on safer side, delete all the files inside the src
folder except for the index.js
file.
Inside the index.js
, remove the import statements for all the deleted files and add new import statement for Signup.js
file as shown below:
import React from 'react';
import ReactDOM from 'react-dom';
import Signup from './Signup';
ReactDOM.render(<Signup/>, document.getElementById('root'));
We don’t have a Signup.js
file created. So let’s take of it and create an empty functional component named Signup
inside it.
<em>import</em> React <em>from</em> 'react';
const Signup = () => {
return null;
}
<em>export</em> <em>default</em> Signup;
It is very important that the Signup
component is a functional component instead of a regular class component, because Hooks can only work on functional component. That’s their whole thing! To give a functional component the powers of a class component.
Building the Form
Let’s build our signup form in the Signup.js
file. Eventually, we are going to add state
to this component using the useState
hook. But right now, this component is returning null
. Let’s replace that with our actual form
:
<em>import</em> React <em>from</em> 'react';
const Signup = () => {
return (
<form>
<div>
<label>First Name</label>
<input type="text" name="firstName" required />
<label>Last Name</label>
<input type="text" name="lastName" required />
</div>
<div>
<label>Email Address</label>
<input type="email" name="email" required />
</div>
<div>
<label>Password</label>
<input type="password" name="password1"/>
</div>
<div>
<label>Re-enter Password</label>
<input type="password" name="password2"/>
</div>
<button type="submit">Sign Up</button>
</form>
)
}
export default Signup;
With that, the form is ready!


I know that this isn’t very visually appealing. But I am going to stick to main objective of this post and show you how to use React Hooks in this form and not waste time and space by working the stylesheet code.
From HTML point of view, the form is ready. But it doesn’t do anything other than ensure that the input fields have some value inside it, thanks to the required
attribute that added to them.
Let’s add some functionality to our form by creating a custom React Hook!
Creating Custom Hooks
Tip: Learn more about writing custom hooks and see these10 custom hooks you can use off-the-shelf.
Any and every form on the Internet has these two event handlers:
input
Let’s create a new custom hook of our own that we can use to handle the submission and input change events. Start by creating a new file named CustomHooks.js
. I will be writing all the custom hooks for this post inside this file. Start by creating a new functional component named useSignUpForm
inside this file.
<em>import</em> React <em>from</em> 'react';
const useSignUpForm = () => {
return null;
}
export default useSignUpForm;
It is very important that the name of this functional component starts with “use”. This functional component is actually going to be our custom hook. And in order for React to recognize any custom hooks in our apps, their name should start with “use”.
Let’s take a look at what we want our custom hook to do for us. First, it should use the built-in useState
hook to keep track of all input values inside our form. So make sure that you import this hook from react
.
import React, <strong>{useState}</strong> from 'react';
The functional component will then take callback
as an input parameter. Callback is a function that gets called whenever the user submits the form.
const useSignUpForm = (callback) => {
return null;
}
We will then use the useState
hook to initialize a state variable and its setter function.
const useSignUpForm = (callback) => {
const [inputs, setInputs] = useState({});
return null;
}
Next, create a function that manages the submit event. This function should simply prevent the default behavior of the browser (which is usually to refresh the page) and call the callback
function.
const useSignUpForm = (callback) => {
const [inputs, setInputs] = useState({});
const handleSubmit = (event) => {
if (event) {
event.preventDefault();
}
callback();
}
return null;
}
Similarly, we need a function have manages the event where the user gives some input. This event will be triggered every time the user enters some input. We will also use the setInputs
function from the hook to update the inputs
state variable with the user’s input.
const handleInputChange = (event) => { event.persist(); setInputs(inputs => ({...inputs, [event.target.name]: event.target.value})); }
And finally, we need to return the handleSubmit
, handleInputChange
, and the inputs
from the custom hook. The functional component will look like this:
const useSignUpForm = (callback) => {
const [inputs, setInputs] = useState({});
const handleSubmit = (event) => {
if (event) {
event.preventDefault();
}
}
const handleInputChange = (event) => {
event.persist();
setInputs(inputs => ({...inputs, [event.target.name]: event.target.value}));
}
return {
handleSubmit,
handleInputChange,
inputs
};
}
With that, the custom hook is ready! But we still need to connect it to our SignUp Form.
Connecting the Hook to the Form
At this point, we have our form and the custom hook ready. But these two things are completely oblivious about the other’s existence. Let’s solve it by import the custom hook into the SignUp.js
file.
import useSignUpForm from './CustomHooks';
The next step is to initialize this custom hook, similar to how we initialized the useState
hook earlier. So inside the SignUp
component, write the following line of code. Take care that this is not inside the return
function of the component.
const {inputs, handleInputChange, handleSubmit} = useSignUpForm();
Here we are simply, destructuring the object that is returned to us by the hook so that we can easily use the state variable and the event handler functions inside the form
element.
First, let’s add the handleSubmit
function to the form
‘s onSubmit
attribute.
<form <strong>onSubmit={handleSubmit}</strong>>
Then to the input
elements inside this function, add the handleInputChange
to the onChange
attribute and add the value
attribute to the element as shown below:
<form onSubmit={handleSubmit}>
<div>
<label>First Name</label>
<input type="text" name="firstName" onChange={handleInputChange} value={inputs.firstName} required />
<label>Last Name</label>
<input type="text" name="lastName" onChange={handleInputChange} value={inputs.lastName} required />
</div>
<div>
<label>Email Address</label>
<input type="email" name="email" onChange={handleInputChange} value={inputs.email} required />
</div>
<div>
<label>Password</label>
<input type="password" name="password1" onChange={handleInputChange} value={inputs.password1}/>
</div>
<div>
<label>Re-enter Password</label>
<input type="password" name="password2" onChange={handleInputChange} value={inputs.password2}/>
</div>
<button type="submit">Sign Up</button>
</form>
The form is finally ready! It does everything a normal form should be able to do. It will wait untill all the required
inputs are filled by the user. Then when the user clicks on the button, it will trigger the submit
event. Also when the user types something in an input field, it will store that value in a state variable.
But you as the user are not able to see anything happening in the browser when the button is clicked.
If you remember, we had passed the callback
function as a parameter to the handleSubmit
function. So I can simply define this callback
function in the Signup.js
file like this:
const signup = () => {
alert(`User Created!
Name: ${inputs.firstName} ${inputs.lastName}
Email: ${inputs.email}`);
}
const {inputs, handleInputChange, handleSubmit} = useSignUpForm( signup);
Now when you go to the browser:


Exporting with Bit
Then, I exported the component to bit.dev and- walla! Our reusable component is ready to be used in all my apps! Awesome :) You can install it with NPM/Yarn or use Bit to develop it right from your own project. Cheers.
Conclusion
Thank you for reading this post! I hope it helped you understand React Hooks a little better and you are now able to build your own custom React Hooks. I have turned this form into a reusable component with some really nice styling and everything. You can get the code for it here:
To learn more about React Hooks, check out my other post on it:
And if you want to learn how build awesome forms with Redux, check out:
Build Awesome Forms In React Using Redux-Form
How to build great forms in React using Redux-forms. Tutorial. blog.bitsrc.io
Please feel free to comment here or hit me up on Twitter if you have any thoughts to share with me! Cheers :beer:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK