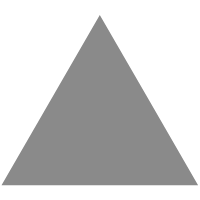
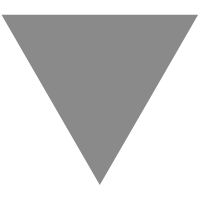
JavaScript parseInt Tutorial
source link: https://www.tuicool.com/articles/hit/iuaQZnV
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
JavaScript parseInt

JavaScript’s parseInt
is a function that will turn any string into an integer (whole number), such as 0, 1, 2, 3 or -1, -2, -3, etc.
Like many things in JavaScript, parseInt
will do it’s best with the input you give it, so for example it will remove spaces and ignore text after the number. It will also ignore anything after the decimal point. For example "10.99"
is converted to 10. If it can’t convert a number it will return NaN which is a symbol that means “Not a Number”.
There is a ‘gotcha’ when using parseInt
, in that if the number begins with zero, it will assume it is an octal (base 8) number and you might get results you don’t expect. For example:
parseInt("010"); // Result: 8 - because it begins with 0 // so the rest of the string "10" is treated // as octal (base 8).
Thankfully there is a way around this. The second parameter to parseInt
is the radix, which is the base you want to use when converting. If you set this to 10, it will always give you the result you expect, treating the string as a decimal.
Here are some examples of using parseInt:
parseInt("10"); // Result: 10 parseInt("10.00"); // Result: 10 parseInt("10.33"); // Result: 10 parseInt("34 45 66"); // Result: 34 parseInt(" 60 "); // Result: 60 parseInt("40 years"); // Result: 40 parseInt("He was 40"); // Result: NaN parseInt("10", 10); // Result: 10 parseInt("010"); // Result: 8 - because it begins with 0 parseInt("010", 10); // Result: 10 - because base 10 is specified // and that is more important than it // beginning with a 0. parseInt("10", 8); // Result: 8 - because base 8 is specified parseInt("0x10"); // Result: 16 - because it begins with 0x parseInt("10", 16); // Result: 16 - because base 16 is specified
Ant that’s it. parseInt
is useful for taking something a person has typed in and treating it like a whole number. Or data in a JSON response from the server that has integer values in strings that you need to use like numbers.
Keep in mind that JavaScript doesn’t have a Integer Type. The result from parseInt has the type “Number” and it happens to be an Integer or NaN. You are free to take the result and add 0.5 to that number, or multiply it by 1.1, etc.
Summary
This was a quick introduction to parseInt
, a handy tool to have when dealing with strings that contain numbers. It will convert a string to a whole number. In most cases you should specify the radix of 10 so it always does what you expect.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK