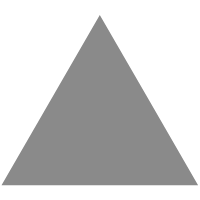
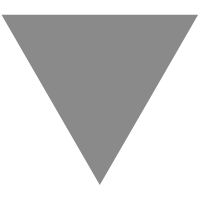
Ionic 4.1 and React: Navigation
source link: https://www.tuicool.com/articles/hit/JbAZ7nb
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
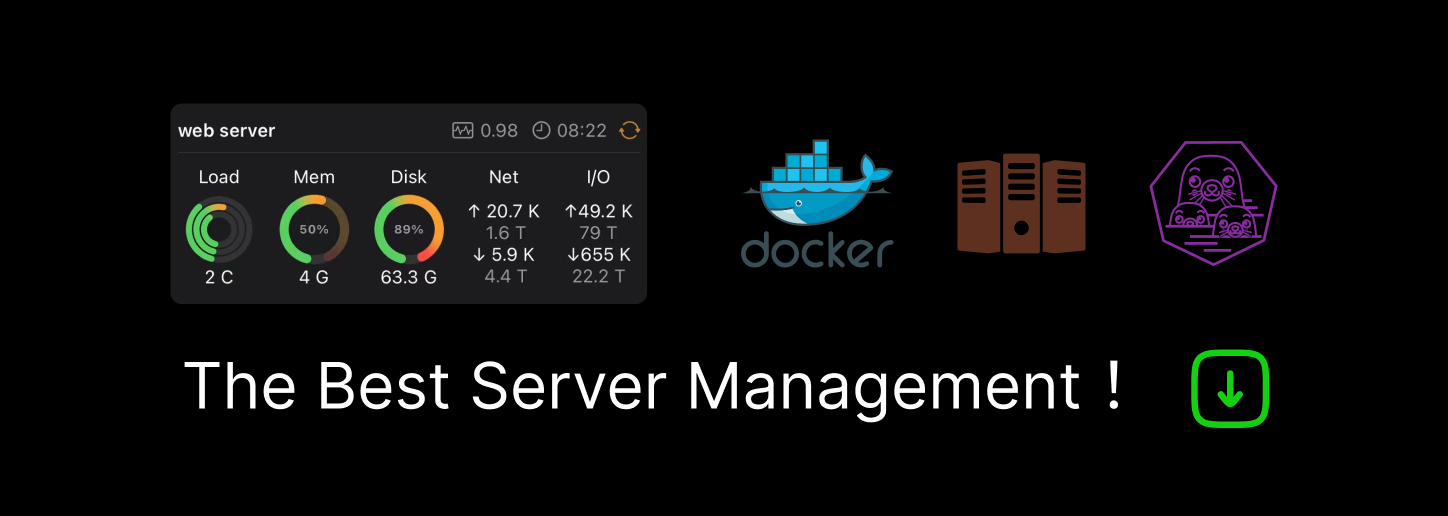
Navigating between pages is a core feature of any mobile application. Let’s look at how we can achieve this with React, React Router and Ionic 4.
For this article, I’m going to assume that you have a React and Ionic 4 application already up and running. If you haven’t done this yet - visit my article on Alligator.io that covers this already .
The core dependencies we’ll need for this project are the following:
$ npm i @ionic/core @ionic/react react-router react-router-dom
:crocodile: Alligator.io recommends ⤵
Fullstack Advanced React & GraphQL by Wes BosCreating Our Pages
For routing to work, we’ll need some pages to route between. Let’s create two new files at src/pages/HomePage.js
and src/pages/BlogPage.js
This allows us to define a HomePage
:
import React from 'react'; import { IonToolbar, IonTitle, IonContent, IonCard, IonHeader, IonCardHeader, IonCardTitle, IonCardSubtitle, IonCardContent, IonButton } from '@ionic/react' const HomePage = () => ( <> <IonHeader> <IonToolbar color="primary"> <IonTitle>Home Page</IonTitle> </IonToolbar> </IonHeader> <IonContent> <IonCard> <IonCardHeader> <IonCardSubtitle>Home Page</IonCardSubtitle> <IonCardTitle>Let's take a look at the blog</IonCardTitle> </IonCardHeader> <IonCardContent> <p>Sounds like a great idea. Click the button below!</p> <IonButton>Blog</IonButton> </IonCardContent> </IonCard> </IonContent> </> ) export default HomePage
And a BlogPage
:
import React from 'react'; import { IonToolbar, IonTitle, IonContent, IonCard, IonHeader, IonCardHeader, IonCardTitle, IonCardSubtitle, } from '@ionic/react' const BlogPage = () => ( <> <IonHeader> <IonToolbar color="primary"> <IonTitle>Blog Page</IonTitle> </IonToolbar> </IonHeader> <IonContent> <IonCard> <IonCardHeader> <IonCardSubtitle>Vue.js</IonCardSubtitle> <IonCardTitle>Ionic 4 and Vue.js</IonCardTitle> </IonCardHeader> </IonCard> <IonCard> <IonCardHeader> <IonCardSubtitle>REACT</IonCardSubtitle> <IonCardTitle>Ionic 4 and React</IonCardTitle> </IonCardHeader> </IonCard> <IonCard> <IonCardHeader> <IonCardSubtitle>ANGULAR</IonCardSubtitle> <IonCardTitle>Ionic 4 and Angular</IonCardTitle> </IonCardHeader> </IonCard> </IonContent> </> ) export default BlogPage
Defining Routes
We can then define the routes for our application inside of App.js
:
import React, { Component } from 'react'; import { BrowserRouter as Router, Route } from 'react-router-dom'; import { IonApp, IonPage, IonRouterOutlet } from '@ionic/react'; import HomePage from './pages/HomePage'; import BlogPage from './pages/BlogPage'; import './App.css'; class App extends Component { render() { return ( <Router> <div className="App"> <IonApp> <IonPage id="main"> <IonRouterOutlet> <Route exact path="/" component={HomePage} /> <Route path="/blog" component={BlogPage} /> </IonRouterOutlet> </IonPage> </IonApp> </div> </Router> ); } } export default App;
While we could just define our Route
with react-router-dom
only, placing the Route
inside of an IonRouterOutlet
will enable animations within route changes.
Navigating Between Two Pages
Navigating between two pages is therefore as easy as taking history
from props
and pushing a new Page
onto the stack.
Let’s update the HomePage
to accommodate this:
const HomePage = ({history}) => ( <> <IonHeader> <IonToolbar color="primary"> <IonTitle>Home Page</IonTitle> </IonToolbar> </IonHeader> <IonContent> <IonCard> <IonCardHeader> <IonCardSubtitle>Home Page</IonCardSubtitle> <IonCardTitle>Let's take a look at the blog</IonCardTitle> </IonCardHeader> <IonCardContent> <p>Sounds like a great idea. Click the button below!</p> <IonButton onclick={(e) => { e.preventDefault(); history.push('/blog')}}>Blog</IonButton> </IonCardContent> </IonCard> </IonContent> </> )
Pretty simple to achieve as you can see! Here’s the results of our work:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK