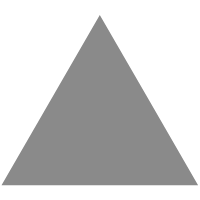
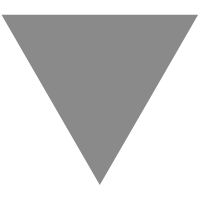
React Native Override Android Hardware Back Button Behavior
source link: https://www.tuicool.com/articles/hit/Qfyi6ff
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Hardware back button is a most important part of every android mobile phone. Back button gives us the functionality to going back in previous activity without any customization in application. If we would on the main home page activity screen then by default pressing back button would exit us from the app. In react native we can modify the android’s hardware back button behavior and override it according to our requirement. We can also disable android’s hardware back button. So in this tutorial we would learn about Override Android Hardware Back Button Behavior in react native.
What we are doing in this tutorial:
In this tutorial we would override the current hardware back button behavior and show a Alert dialog message asking user to leave the application. If user press the Yes button then we would use the BackHandler . exitApp ( ) inbuilt function to exit manually from application and if user press the No button then it will simply print a message in console.
Contents in this project React Native Override Android Hardware Back Button Behavior:
1. Import StyleSheet , Platform , View , Text , Alert and BackHandler component in App.js file.
import React, { Component } from 'react'; import { StyleSheet, Platform, View, Text, Alert, BackHandler } from 'react-native';
2. Create constructor() in your project. Here we would bind the back_Button_Press() function with current this object of class.
constructor(props) { super(props); this.back_Button_Press = this.back_Button_Press.bind(this); }
3. Create componentWillMount() function and adding event listener with BackHandler component. Using this we would define our function here which we would call on back button press.
componentWillMount() { BackHandler.addEventListener('hardwareBackPress', this.back_Button_Press); }
4. Create componentWillUnmount() function and again remove event listener with BackHandler component. It will remove the event listener on back button press event.
componentWillUnmount() { BackHandler.removeEventListener('hardwareBackPress', this.back_Button_Press); }
5. Creating back_Button_Press() main function. In this function we would create a Alert dialog message with YES and NO button. On YES button press event we would call the default BackHandler.exitApp() function. This function allow us the exit from the app. At last we would return true in function to enable back button override behavior.
back_Button_Press = () => { // Put your own code here, which you want to exexute on back button press. Alert.alert( ' Exit From App ', ' Do you want to exit From App ?', [ { text: 'Yes', onPress: () => BackHandler.exitApp() }, { text: 'No', onPress: () => console.log('NO Pressed') } ], { cancelable: false }, ); // Return true to enable back button over ride. return true; }
6. Create a simply text component in render’s return block.
render() { return ( <View style={styles.MainContainer}> <Text style={styles.TextStyle}>Press The Hardware Back Button to See Effect.</Text> </View> ); }
7. Creating Style.
const styles = StyleSheet.create({ MainContainer: { flex: 1, paddingTop: (Platform.OS) === 'ios' ? 20 : 0, alignItems: 'center', justifyContent: 'center', }, TextStyle: { color: '#000', textAlign: 'center', fontSize: 22, padding: 10 }
8. Complete source code for App.js file class.
import React, { Component } from 'react'; import { StyleSheet, Platform, View, Text, Alert, BackHandler } from 'react-native'; export default class App extends Component { constructor(props) { super(props); this.back_Button_Press = this.back_Button_Press.bind(this); } componentWillMount() { BackHandler.addEventListener('hardwareBackPress', this.back_Button_Press); } componentWillUnmount() { BackHandler.removeEventListener('hardwareBackPress', this.back_Button_Press); } back_Button_Press = () => { // Put your own code here, which you want to exexute on back button press. Alert.alert( ' Exit From App ', ' Do you want to exit From App ?', [ { text: 'Yes', onPress: () => BackHandler.exitApp() }, { text: 'No', onPress: () => console.log('NO Pressed') } ], { cancelable: false }, ); // Return true to enable back button over ride. return true; } render() { return ( <View style={styles.MainContainer}> <Text style={styles.TextStyle}>Press The Hardware Back Button to See Effect.</Text> </View> ); } } const styles = StyleSheet.create({ MainContainer: { flex: 1, paddingTop: (Platform.OS) === 'ios' ? 20 : 0, alignItems: 'center', justifyContent: 'center', }, TextStyle: { color: '#000', textAlign: 'center', fontSize: 22, padding: 10 } });
Screenshots:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK