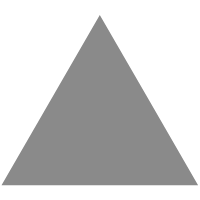
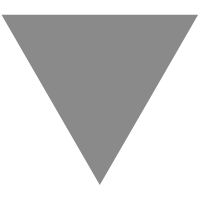
Making the backend of your React App configurable
source link: https://www.tuicool.com/articles/hit/VF7Jr2I
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Nowadays, the frontend and backend of a web application usually are separate parts – oftentimes implemented using different technologies – communicating with each other using HTTP or websockets. For simplicity and smaller deployments they are hostet on the same web server. There are several reasons to deploy them on different servers like load distribution, security, different environments running the same frontend with differing backends and so on.
To allow separate deployments without changing the frontend code per deployment we need to make the backend transparently configurable. Fortunately, this is relatively easy for frontend written in React and set up with create-react-app . To make this fully transparent for your frontend code we need to
- Make the backend URL configurable
- Replace the fetch() function to use the configured backend
- Activate the setup at the start of our app
Configuring a React App
Create-react-app provides a configuration mechanism with custom environment variables
using .env
-files. We can simply provide different env-files for our environments where we can configure different aspects of our application. In our use case this is the backend URL.
// The base url of the backend API. Add path prefix if the API does not run at the server root. REACT_APP_BACKEND_API_BASE_URL=http://some.other.server:5000
Inside our React App we can reference the configured values using {process.env.REACT_APP_BACKEND_API_BASE_URL}
.
Making the use of our configured backend transparent
In a modern JavaScript app the main mean to communicate with the backend is the fetch()-API . To make the use of our configured backend transparent we can replace the global fetch()-function with our version like so:
// remember the original fetch-function to delegate to const originalFetch = global.fetch; export const applyBaseUrlToFetch = (baseUrl) => { // replace the global fetch() with our version where we prefix the given URL with a baseUrl global.fetch = (url, options) => { const finalUrl = baseUrl + url; return originalFetch(finalUrl, options); }; };
That way all of our fetch() calls are re-routed to the configured backend.
Activating our fetch()-customization
Now that we have all the pieces of our infrastructure in place we need to activate the changes to fetch on application startup. So we add code like below to our index.js
:
// If we have a differing backend configured, replace the global fetch() if (process.env.REACT_APP_BACKEND_API_BASE_URL !== undefined && process.env.REACT_APP_BACKEND_API_BASE_URL !== '') { applyBaseUrlToFetch(process.env.REACT_APP_BACKEND_API_BASE_URL); }
Now all our calls to a relative URL will be prefixed with a configurable base and that way different backends can be used with the same application code.
Caveats
The above approach works nicely if you have exactly one backend for your app and do not fetch from other sources. If you do, you may want to expose the original fetch function as something like fetchExternal()
to be able to explicitly fetch from other sources.
In addition, if frontend and backend reside on different servers/sites using differring DNS-names you will have to configure CORS for your backends or your browser will refuse to make the requests!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK