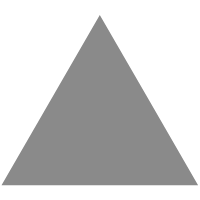
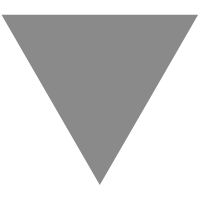
Displaying an Interactive HERE Map With NativeScript and Angular
source link: https://www.tuicool.com/articles/hit/3a6bymA
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Over the past few months, I've written several pieces of content around developing web applications using Angular that included interactive HERE maps as well as tutorials around using HERE maps in progressive web applications using the Ionic Framework. In both these circumstances, the HERE JavaScript SDK was highlighted because we were essentially building web applications, regardless if they were bundled for mobile or not.
What if we wanted to change this and build a native Android or iOS application that made use of a cross-platform framework?
In this tutorial, we're going to see how to develop an Android and iOS mobile application that makes use of NativeScript and Angular .
Before going forward, I have to make it clear that there is no official NativeScript plugin or SDK for HERE . The plugin that I'll be using in this tutorial was built by an awesome member of our community, Osei Fortune . With enough demand, I'm sure this plugin will get even better.
What we plan to accomplish in this tutorial can be seen in the following animated image:
As you can see, we're displaying an interactive map, placing a marker, and interacting with that marker. The Angular code we use with NativeScript can be used in both Android and iOS to create a native application.
Creating a Fresh NativeScript Project With the HERE Plugin Dependencies
To keep this tutorial simple and easy to understand, we're going to work with a new project. The assumption is that you're using version 5.1.1 or higher of the Telerik NativeScript Shell (TNS).
From the command line, execute the following:
tns create here-project --template tns-template-blank-ng
The above command will create a project that uses an Angular template. NativeScript supports other frameworks as well, but we won't get into that here. With the project created, execute the following to install the community-driven HERE plugin:
tns plugin add nativescript-here
More details on this plugin can be found on Osei Fortune's official GitHub repository .
Before we start showing and interacting with maps, we need to do some configuration steps. Open your project's App_Resources/Android/src/main/AndroidManifest.xml file and include the following:
<meta-data android:name="com.here.android.maps.appid" android:value="APP-ID-HERE"/> <meta-data android:name="com.here.android.maps.apptoken" android:value="APP-TOKEN-HERE"/>
The above lines should be placed within the <application>
tags, but not as attributes. To get more perspective on how this AndroidManifest.xml file should look, see the following:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="__PACKAGE__" android:versionCode="10000" android:versionName="1.0"> <supports-screens android:smallScreens="true" android:normalScreens="true" android:largeScreens="true" android:xlargeScreens="true"/> <uses-sdk android:minSdkVersion="17" android:targetSdkVersion="__APILEVEL__"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.INTERNET"/> <application android:name="com.tns.NativeScriptApplication" android:allowBackup="true" android:icon="@drawable/icon" android:label="@string/app_name" android:theme="@style/AppTheme"> <meta-data android:name="com.here.android.maps.appid" android:value="APP-ID-HERE"/> <meta-data android:name="com.here.android.maps.apptoken" android:value="APP-TOKEN-HERE"/> <activity android:name="com.tns.NativeScriptActivity" android:label="@string/title_activity_kimera" android:configChanges="keyboardHidden|orientation|screenSize" android:theme="@style/LaunchScreenTheme"> <meta-data android:name="SET_THEME_ON_LAUNCH" android:resource="@style/AppTheme" /> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name="com.tns.ErrorReportActivity"/> </application> </manifest>
The two lines that we've added have placeholder values for our tokens. You'll need to create a free HERE developer account to obtain your tokens. When you obtain your tokens, make sure you generate them as Android and iOS, not REST and JavaScript.
With the AndroidManifest.xml file out of the way, we need to make the plugin a little more Angular friendly.
Open the project's src/app/app.module.ts file and include the following two lines:
import { registerElement } from "nativescript-angular/element-registry"; registerElement("HereMap", () => require("nativescript-here").Here);
What we're doing is we're making the XML component a little easier to use. We're registering the tag names so that we can use them with Angular.
At this point in time, the plugin is properly configured. We can now start development.
Showing a HERE Map With NativeScript and Angular
Because we're using a basic project, we're only going to have one page to work in and no additional routes. We're going to add the TypeScript logic before we work with our XML.
Open the project's src/app/home/home.component.ts file and include the following:
import { Component, OnInit } from "@angular/core"; import { HereMarker } from "nativescript-here"; @Component({ selector: "Home", moduleId: module.id, templateUrl: "./home.component.html" }) export class HomeComponent implements OnInit { public constructor() { } public ngOnInit(): void { } public onMapReady(event) { const map = event.object; map.addMarkers(<HereMarker[]>[{ id: 1, latitude: 37.7397, longitude: -121.4252, title: "Tracy, CA", description: "The best place in California!", draggable: true, onTap: (marker) => { const updatedMarker = Object.assign({}, marker, { selected: !marker.selected }); map.updateMarker(updatedMarker); } }]); } }
What we're paying attention to is the onMapReady
method. When using a HERE map, we can't interact with it until it has fully initialized. When the map is ready, it will call this onMapReady
method.
In the onMapReady
method, we are adding a single marker and giving it information as well as a tap event. When we tap on this marker, the title
and description
will show.
The onMapReady
method is not absolutely necessary to show our map. To show our map, open the project's src/app/home/home.component.html file and include the following:
<ActionBar class="action-bar"> <Label class="action-bar-title" text="{N} HERE Example"></Label> </ActionBar> <GridLayout class="page"> <HereMap mapStyle="normal" (mapReady)="onMapReady($event)" id="map" zoomLevel="10" disableZoom="false" disableScroll="false" latitude="37.7397" longitude="-121.4252"> </HereMap> </GridLayout>
Notice that we're providing the onMapReady
method as an XML attribute. We're also providing basic setup information for showing the map in our application.
Conclusion
You just saw how to include a HERE map within your NativeScript -based Android and iOS application. I have to reiterate that this is a plugin that is maintained by our community member, Osei Fortune , and he made an awesome first iteration at it. If you found it valuable, I encourage you to send him a message to thank him for his work!
If you're interested in the Ionic Framework alternative that I demonstrated, check out my tutorial titled, Display an Interactive HERE Map in an Ionic Framework Application .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK