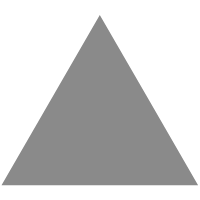
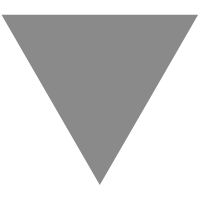
Android-LayoutParams的那些事
source link: https://tryenough.com/android-layoutparams?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
LayoutParams是什么?
LayoutParams是View用来告诉它的父控件如何放置自己的,LayoutParams是ViewGroup类里的一个静态内部类。
ViewGroup.LayoutParams仅仅描述了这个View想要的宽度和高度。可以理解成LayoutParams是子View告诉父View自身宽高的桥梁。
可取的值:
- fill_parent
强制性使子视图的大小扩展至与父视图大小相等(不含 padding )
- match_parent
与fill_parent相同,用于Android 2.3 & 之后版本
- wrap_content
自适应大小,强制性地使视图扩展以便显示其全部内容(含 padding )
不同ViewGroup的继承类对应着不同的ViewGroup.LayoutParams的子类,例如:ViewGroup.MarginLayoutParams, WindowManager.LayoutParams等。
实际使用的一个例子
Activity:
public class MoveActivity extends AppCompatActivity { private ImageView mIv; private TextView mTvBlue; private TextView mTvGreen; private ViewGroup.LayoutParams mVgLp; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_move); mIv = (ImageView) findViewById(R.id.mIv); mTvBlue = (TextView) findViewById(R.id.mTvBlue); mTvGreen = (TextView) findViewById(R.id.mTvGreen); mVgLp = mIv.getLayoutParams(); findViewById(R.id.mTvMoveRight).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { ViewGroup.LayoutParams vg_lp = mIv.getLayoutParams(); // RelativeLayout.LayoutParams 不可抽取为成员变量 RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(vg_lp); params.addRule(RelativeLayout.ALIGN_PARENT_RIGHT, R.id.mIv); mIv.setLayoutParams(params); //使layout更新 } }); findViewById(R.id.mTvMoveLeft).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // 左 (默认处于顶部,所以看起来是左上) RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(mVgLp); params.addRule(RelativeLayout.ALIGN_PARENT_LEFT); mIv.setLayoutParams(params); } }); findViewById(R.id.mTvCenter).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // 居中 RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(mVgLp); params.addRule(RelativeLayout.CENTER_IN_PARENT); mIv.setLayoutParams(params); } }); findViewById(R.id.mTvLeftVer).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // 左部垂直居中 RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(mVgLp); params.addRule(RelativeLayout.ALIGN_PARENT_LEFT|RelativeLayout.CENTER_VERTICAL); mIv.setLayoutParams(params); } }); findViewById(R.id.mTvRightBot).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // 右下 RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(mVgLp); params.addRule(RelativeLayout.ALIGN_PARENT_BOTTOM); params.addRule(RelativeLayout.ALIGN_PARENT_RIGHT); mIv.setLayoutParams(params); } }); // 绿在蓝右边 findViewById(R.id.mTvaGRightB).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { ViewGroup.LayoutParams vgLp = mTvGreen.getLayoutParams(); RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(vgLp); params.addRule(RelativeLayout.RIGHT_OF,R.id.mTvBlue); mTvGreen.setLayoutParams(params); } }); // 绿在蓝下边 findViewById(R.id.mTvGElowB).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { ViewGroup.LayoutParams vgLp = mTvGreen.getLayoutParams(); RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(vgLp); params.addRule(RelativeLayout.BELOW,R.id.mTvBlue); mTvGreen.setLayoutParams(params); } }); } }
布局xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout android:id="@+id/activity_main" xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:orientation="vertical" tools:context="com.amqr.movetest.MoveActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <TextView android:id="@+id/mTvMoveLeft" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#6600ff00" android:padding="10dp" android:layout_margin="10dp" android:text="黑色最左!!"/> <TextView android:id="@+id/mTvMoveRight" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:background="#6600ff00" android:layout_margin="10dp" android:text="黑色最右!!"/> <TextView android:id="@+id/mTvCenter" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#6600ff00" android:padding="10dp" android:layout_margin="10dp" android:text="黑色居中"/> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <TextView android:id="@+id/mTvLeftVer" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:background="#6600ff00" android:layout_margin="10dp" android:text="黑色靠左垂直居中"/> <TextView android:id="@+id/mTvRightBot" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#6600ff00" android:padding="10dp" android:layout_margin="10dp" android:text="黑色右下角"/> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <TextView android:id="@+id/mTvaGRightB" android:layout_width="wrap_content" android:layout_height="wrap_content" android:padding="10dp" android:background="#6600ff00" android:layout_margin="10dp" android:text="绿在蓝右边"/> <TextView android:id="@+id/mTvGElowB" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#6600ff00" android:padding="10dp" android:layout_margin="10dp" android:text="绿在蓝下边"/> </LinearLayout> <RelativeLayout android:layout_width="match_parent" android:layout_height="200px" android:background="#66ff0000" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="RelativeLayout" android:layout_centerInParent="true" /> <ImageView android:id="@+id/mIv" android:layout_width="30dp" android:layout_height="40px" android:background="#000" android:layout_centerHorizontal="true" /> <TextView android:id="@+id/mTvBlue" android:layout_width="20dp" android:layout_height="20dp" android:text="蓝" android:gravity="center" android:background="#660000ff" /> <TextView android:id="@+id/mTvGreen" android:layout_width="20dp" android:layout_height="20dp" android:text="绿" android:gravity="center" android:background="#6600ff00" android:layout_below="@id/mTvBlue" /> </RelativeLayout> </LinearLayout>
热度: 4
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK