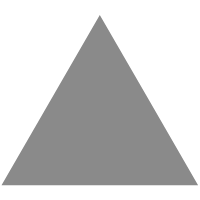
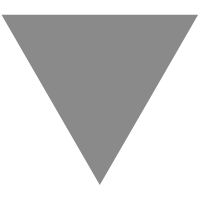
What is an IIFE?
source link: https://www.tuicool.com/articles/hit/eYvIr22
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
This post was originally published on my blog Fundamentals of Code
Feeling a Little IIFE?
I have covered modules in previous posts, and this week I wanted to create a short synapse of Immediately Invoked Function Expressions or often referred to as IIFE (pronounced if-ee or iffy ) statements. These are similar to modules in that they help to encapsulate variable and eliminate the potential for global pollution of values. If you are curious about some additional examples and how to use the module pattern check out my previous post , especially the revealing module portion.
Example of An IIFE
Let's take a look at what an IIFE looks like, and then dissect further.
(function(){ const iife = "IIFE" console.log(iife) })(); // console.log(iife)//reference error exception will be thrown
Notice the basic creation of an IIFE looks like the following (function(){//...code goes here}());
. This is all that is needed to wrap our code in the function call. Don't forget the trailing anonymous function ()
. When the browser's javascript compiler parses our code, it will execute this snippet immediately. In the module pattern, we are assigning this IIFE declaration to a variable to use later, and returning or exposing public functions and properties.
Working on Global Properties
A use case for IIFE's is when we want to work on a global variable without modifying the variable. We can do a variety of things with this in the IIFE, it has a closure of its own and accepts a parameter in the callback at its tail. Notice in this example, We declare a variable, and then pass it in at the end. The IIFE has a parameter that will then work on the passed in a variable without modifying the global variable. Pretty nifty right!
let x = 0; (function(y){ y = y + 1; console.log(`y = ${y}`);//1 //note: we have modified y as a parameter passed in without modifying our global variable. console.log(`inside IIFE x = ${x}`);//0 })(x); console.log(`x = ${x}`);//0
Another thing to consider is passing in the window and document objects.
(function(window, document ) { // You can now reference the window and document in a local scope console.log(document) }(window, document));
Return values from an IIFE's
You can also return values from an IIFE in the following way.
const val = (function(){ return "A value from our IIFE" }()); console.log(val);//Note that you can also return much more complicated objects if needed
This is the power if IIFE's and fundamentally how the revealing module pattern works. For an example of that please refer to my previous post on modules . As I mentioned earlier this pattern is used extensively by modules, as it enforces a self-contained scope within the closure. This is key to for this pattern as it allows for them to be reusable, maintainable, and reduce namespace pollution.
Legacy or Die!
I understand too, that this pattern is not as widely used within more modern applications, however, you should be aware of this as it will appear in older code bases, and there still may be some use cases for this pattern.
Once again thanks for reading and I hope you find this useful. Please share and comment. Let me know if you still use IIFE's!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK