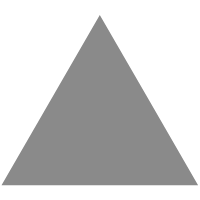
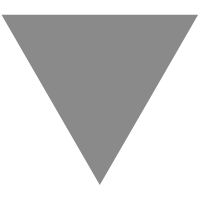
How We Built React Carousel
source link: https://www.tuicool.com/articles/hit/6FnAzev
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
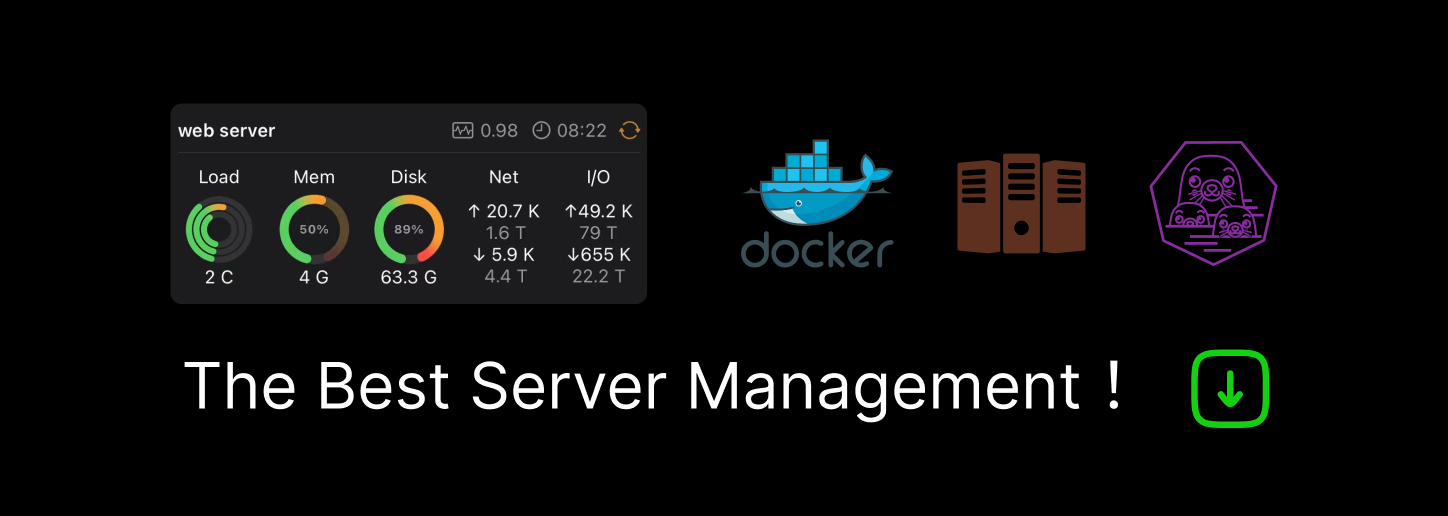
At Brainhub, for the most part, we useReact JS to build our user interfaces. The growing size of the React ecosystem gives us a greater variety of existing open-source components. On top of that, thanks to React’s modularity and focus on component reusability, we can utilize components between different projects.
Carousel components are a staple in many applications, and no wonder — they are a great way to draw focus to images without overwhelming the user or cluttering the page. Unfortunately, already existing React carousel components had issues which made them unsuitable for our purposes.
This resulted essentially having to reinvent the wheel in every project and building a carousel from scratch or using inferior solutions for a relatively simple problem.
We wanted a React carousel that did not depend on any large external libraries (such as jQuery) and was extensible and lightweight . We also needed it to be customizable enough to fit the large number of applications we worked on, but simultaneously good enough out of the box that we did not need to worry about reinventing the wheel.
Failing to find a React carousel like that in the existing ecosystem, we decided to create our own.
The main objectives when building react-carousel were to:
- Avoid using external libraries — we focused on making the carousel as lightweight and fast as possible (at the time of writing it is only 11KB gzipped).
- Allow for maximum pluggability — we want to let the user replace existing parts of the carousel if he or she so chooses without hassle.
- Provide sensible defaults — our React carousel should be usable and pretty right out of the box.
Managing complexity
The core of react-carousel deals exclusively with controlling whatever is inside. That is, essentially, everything you see — images, arrows — can be switched out for custom components and linked to the carousel with minimal effort .
This is the entire code driving the React carousel in the gif above.
import React from "react"; import Carousel from "@brainhubeu/react-carousel"; import "@brainhubeu/react-carousel/lib/style.css"; import Image1 from "./assets/1.jpg"; import Image2 from "./assets/2.jpg"; import Image3 from "./assets/3.jpg"; const App = () => ( <div className="App" style={{ width: "600px", margin: "auto", padding: "50px" }} > <Carousel arrows infinite> <img src={Image1} /> <img src={Image2} /> <img src={Image3} /> </Carousel> </div> ); export default App;
You will notice that the React carousel works just by wrapping any number of React components into a <Carousel /> component. <Carousel /> itself takes a number of props which deal with its behavior (you can see the full list of available options in the docs ).
A common design pattern in react-carousel is to expose API with different levels of complexity, depending on how much customization is required. For instance, if the user wishes to add arrows to a newly created bare carousel, he or she can add the boolean arrows prop that will render the default arrows.
However, if a greater level of customization is desired, the user can instead add arrowLeft and arrowRight props. Each of them takes a React component that will determine the appearance of the arrows.
Aside from the arrows, there are many more aspects of the carousel that can be modified this way – such as dots showing the position of the carousel, thumbnails, draggability, number of carousel slides per page and more.
Controlled component
Another helpful property of react-carousel is that you can use it as a fully controlled component – meaning you can control it from the parent component through props rather than solely through user input.
This means you can use the full power of React to interact with it from other places in your app or, if necessary, couple it with otherReact libraries like Redux to make your carousel even more powerful.
Of course, using the carousel as a controlled component is entirely optional and you can still just throw a few slides at it without any props and it still is going to work.
React carousel under the hood
Beyond giving the user many options for customization, react-carousel does several things which ensure good performance and usability right out of the box.
First, all the movement in the carousel is implemented using smooth CSS animations . We put in a lot of effort to ensure that we cover special cases and do not compromise on good appearance. Events like scrolling by multiple slides are made to look to impress.
On top of that, we made sure that the carousel is fully responsive and renders well on any device and any screen size. The carousel always resizes itself to the largest available width and looks great on mobile, tablet and desktop. This is complemented by smooth touch controls.
In short…
We think this is the best React carousel available. Feel free to check it out yourself! View react-carousel on GitHub.

Marek Chotoborski
Marek Chotoborski is a JavaScript Full Stack Developer at Brainhub (a software house building awesome Node.js web and mobile apps).
Recommend
-
46
One of the problems in web development today is the entangling of different layers. Not only do we face strong coupling to multiple dependencies, but we also wire logical code directly to some styling or presentation laye...
-
8
Looped carousel for React Native Full-fledged "infinite" carousel for your next react-native project. Supports iOS and Android. Based on react-native framework by Faceboo...
-
18
react-native-imaged-carousel-card Fully customizable & Lovely Imaged Carousel Card for React Native. Installation Add the dependency: npm i react-native-imaged-carousel-card Peer D...
-
11
slidr-that-rock Carousel mobile application in React Native using Expo To run the app locally - git clone https://github.com/Lamisa-zamzam/slidr-that-rock cd slidr-that-rock npm i expo start
-
5
Carousel A smooth image carousel built with React Native's FlatList component Oc...
-
6
Carousel Test lib React Native Snap Carousel Nov 03, 2021...
-
10
react-native-simple-carousel Installation npm i --save @wecraftapps/react-native-simple-carousel Basic usage import { SimpleCarousel } from '@wecraftapps/react-nat...
-
6
React-carousel this is react-carousel component and it suport Responsive layout!(suport gesture operation!) 这是个react轮播图组件,并且它是响应式的!(支持手势操作!) demo...
-
8
React Native Swipeable Carousel A simple swipeable carousel created on the top of react-native-pager-view Preview
-
7
Building a WebGL Carousel with React Three Fiber and GSAP Learn how to create an interactive 3D carousel using WebGL, React Three Fiber, and GSAP with this step-by-step tutorial.
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK