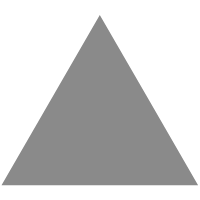
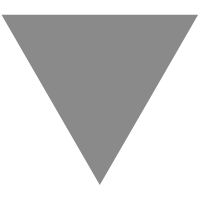
Picking an Interactive Map Theme With Vue.js
source link: https://www.tuicool.com/articles/hit/6rEJBjA
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A few weeks ago, Jayson Delancey had written an awesome tutorial around switching between visual themes for a map on demand using React. This is useful when it comes to scenarios such as switching between day and night mode on a map depending on the local time or similar. The problem with this is that his tutorial was written with React when there are a bunch of other popular web frameworks available.
In this tutorial, we’re going to see almost the same material that Jayson demonstrated, but this time using the Vue.js JavaScript framework.
Before getting too far ahead of ourselves, what we plan to accomplish can be seen in the following animated image:
The above image only scratches the surface of what can be accomplished, but, as you can see, we have a map with a set of buttons below it. Clicking each of the buttons will give the map a different visual theme.
Building a Map Component With the Vue.js Framework
Showing a map with Vue.js isn’t new. I had written about the basics in a tutorial titled, Showing a HERE Map with the Vue.js JavaScript Framework , and it accomplishes half of what we’re trying to do.
Rather than going through the steps in detail numerous times, we’re going to get a project up and running with a map component without the details.
With the Vue CLI installed and configured, execute the following:
vue create theme-switcher
Choose the defaults when creating the project and when it is created, open the project’s public/index.html file and change it to contain the following:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width,initial-scale=1.0"> <link rel="icon" href="<%= BASE_URL %>favicon.ico"> <title>theme-switcher</title> <link rel="stylesheet" type="text/css" href="https://js.api.here.com/v3/3.0/mapsjs-ui.css?dp-version=1526040296" /> </head> <body> <noscript> <strong>We're sorry but theme-switcher doesn't work properly without JavaScript enabled. Please enable it to continue.</strong> </noscript> <div id="app"></div> <!-- built files will be auto injected --> <script type="text/javascript" src="https://js.api.here.com/v3/3.0/mapsjs-core.js"></script> <script type="text/javascript" src="https://js.api.here.com/v3/3.0/mapsjs-service.js"></script> <script type="text/javascript" src="https://js.api.here.com/v3/3.0/mapsjs-ui.js"></script> <script type="text/javascript" src="https://js.api.here.com/v3/3.0/mapsjs-mapevents.js"></script> </body> </html>
With the appropriate stylesheets and JavaScript libraries included, the map component can be created. Create a src/components/HereMap.vue file within the project and include the following:
<template> <div class="here-map"> <div ref="map" ></div> </div> </template> <script> export default { name: "HereMap", data() { return { platform: {}, map: {} }; }, props: { appId: String, appCode: String }, mounted() { this.platform = new H.service.Platform({ app_id: this.appId, app_code: this.appCode, }); var layers = this.platform.createDefaultLayers(); this.map = new H.Map( this.$refs.map, layers.normal.map, { center: {lat: 37.73987, lng: -121.42618}, zoom: 14, } ); var events = new H.mapevents.MapEvents(this.map); var behavior = new H.mapevents.Behavior(events); var ui = H.ui.UI.createDefault(this.map, layers); } } </script> <style scoped></style>
The above component, when used, will give you an interactive map centered on a location. To use this component, open the project’s src/App.vue file and include the following:
<template> <div id="app"> <HereMap appId="APP-ID-HERE" appCode="APP-CODE-HERE" /> </div> </template> <script> import HereMap from './components/HereMap.vue' export default { name: "app", components: { HereMap } } </script> <style> body { background-color: #F0F0F0; } </style>
At this point in time, we’re caught up. If you run the application, a map should appear, assuming that you’ve changed the placeholder appId
and appCode
values with your own from the HERE Developer Portal .
If you’d like to get more details on anything that we did above, I encourage you to read the previous tutorial that I wrote on the subject.
Watching for Changes to a Component Property and Reacting
With the map component functional, we want to be able to change the theme on demand. There are many ways to do this, but to remain as similar to the React example as possible, we’re going to watch our properties for changes and update the theme when changes happen.
In the project’s src/components/HereMap.vue file, you’re going to want to add another possible props
to the list:
props: { theme: String, appId: String, appCode: String },
The theme
will be our current active theme. To watch for changes to this variable and react to them, we can make use of the watch
object in Vue.js:
watch: { theme(newVal, oldVal) { var tiles = this.platform.getMapTileService({ "type": "base" }); var layer = tiles.createTileLayer( "maptile", newVal, 256, "png", { "style": "default" } ); this.map.setBaseLayer(layer); } }
When a change to the theme
variable happens, we are going to create a new tile layer with the selected theme and default style. There are quite a few styles that can be chosen beyond the default.
To see these changes in action, we can revisit the src/App.vue file. In the src/App.vue file, make it look like the following:
<template> <div id="app"> <HereMap :theme="theme" appId="APP-ID-HERE" appCode="APP-CODE-HERE" /> <br /> <button v-on:click="switchTheme('normal.day')">Normal - Day</button> <button v-on:click="switchTheme('normal.day.grey')">Normal - Day (Grey)</button> <button v-on:click="switchTheme('normal.day.transit')">Normal - Day (Transit)</button> <button v-on:click="switchTheme('normal.night')">Normal - Night</button> <button v-on:click="switchTheme('normal.night.grey')">Normal - Night (Grey)</button> <button v-on:click="switchTheme('reduced.night')">Reduced - Night</button> <button v-on:click="switchTheme('reduced.day')">Reduced - Day</button> </div> </template> <script> import HereMap from './components/HereMap.vue' export default { name: "app", data() { return { theme: "normal.day" }; }, components: { HereMap }, methods: { switchTheme(theme) { this.theme = theme; } } } </script> <style> body { background-color: #F0F0F0; } </style>
A few things have been added since we last modified this file. We’ve created a theme
variable with a default value. This theme
variable is not directly connected to the theme
property we saw in the component, it is just coincidentally named the same. The switchTheme
method will set this variable to something new.
Within the HTML, we have several buttons with string values to be passed to the switchTheme
method. Because the theme
variable is bound to the theme
property, when changes happen, the watch
will trigger within the component itself.
Conclusion
You just saw how to use Vue.js to dynamically switch between HERE map themes. As previously mentioned, this tutorial takes Jayson Delancey’s example to new places because it uses Vue.js instead of React.js. In the future we’ll explore doing the same, but with Angular.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK