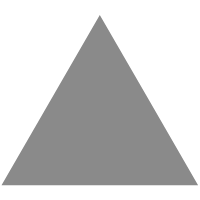
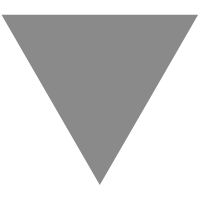
CRUD Operations Using Pure React
source link: https://www.tuicool.com/articles/hit/vQR7ZjI
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.


Now that we have already written same todo application usingVanilla JavaScript andAngular, its time to take this same example a little further and use even more popular framework i.e. react. This example assumes that you already installed node and create-react-app on your system.
First of all lets create new react app with below command.
create-react-app todo
Give it a few seconds and then you should have a todo folder in your file system. CD into that folder.
First thing is that create a new file called Todo.js inside src/ folder and put below initial code into it:
import React, { Component } from 'react';
class Todo extends Component { render() { return(<h1>This message is from Todo component</h1>) } }
export default Todo;
First we are importing React and Component from react core.
Then creating Todo component which extends from Component .
Todo component has a render method which renders JSX with h1 element and text “This message is from Todo component”.
Finally we are exporting this component in order to use it in the rest of our project.
Now open src/App.js file and import our newly created Todo component after the import of App.css file.
Once there, now use this component inside render method of App component.
import React, { Component } from 'react'; import logo from './logo.svg'; import './App.css';
// import Todo component here import Todo from './Todo';
class App extends Component {
constructor(props) { super(props);
this.state = { show: false }; }
render() { // use Todo component inside render method. return ( <div className="App"> <Todo /> </div> ); } }
export default App;
Now that we have the basic Todo Component file and imported in App component and used it. Its time to add some mockData . This time we are going to use React states to work with our data. This makes things easier in order to perform CRUD operations and update the view accordingly. Add below code to Todo component:
class Todo extends Component { state = { edit: false, id: null, mockData: [{ id: '1', title: 'Buy Milk', done: false, date: new Date() }, { id: '2', title: 'Meeting with Ali', done: false, date: new Date() }, { id: '3', title: 'Tea break', done: false, date: new Date() }, { id: '4', title: 'Go for a run.', done: false, date: new Date() }] } }
State is like a data store to the ReactJS component. It is mostly used to update the component when user performed some action like clicking button
, typing some text
, pressing some key
, etc.
Above state can also be placed inside constructor. Choose whichever method you like.
As you can see, state in React is simply a javascript object with properties such as edit , id and mockData . Edit property is a boolean through which will be used to show and hide edit form to edit particular item in mockData . ID property is used to set id of current item inside mockData to perform update operation.
Now that we have the mockData added to state which is also called initial state , time to add JSX. If you would like to know more about JSX then head over here for more details. However, it is a syntax extension to JavaScript which produces React elements to render data on pages.
JSX lists all the items in mockData i.e. perform R operation of CRUD. To do that add below render method to the class.
render() { return ( <div> <form onSubmit={this.onSubmitHandle.bind(this)}> <input type="text" name="item" className="item" /> <button className="btn-add-item">Add</button> </form> <ul> {this.state.mockData.map(item => ( <li key={item.id}> {item.title} <button onClick={this.onDeleteHandle.bind(this, item.id)}>Delete</button> <button onClick={this.onEditHandle.bind(this, item.id, item.title)}>Edit</button> <button onClick={this.onCompleteHandle}>Complete</button> </li> ))} </ul> </div> ); }
Render method is simple, first it has the form which is used to add new item into todo list. This form has onSubmit event and it calls onSubmitHandle method which we will write later in this component.
Then we have ul and simply map through all the items inside mockData and present the title and add same buttons as in our previous examples i.e. Delete, Edit and Complete. Now if you run your application using “npm start” command you should see something like this.


Now that R operation is completed, It is time to add create operation which is C in CRUD. Add onSubmitHandle method to Todo Component like below.
onSubmitHandle(event) { event.preventDefault();
this.setState({ mockData: [...this.state.mockData, { id: Date.now(), title: event.target.item.value, done: false, date: new Date() }] });
event.target.item.value = ''; }
onSubmitHandle method is called when the Add button is clicked. Here we use setState method on Todo’s state which is:
setState()
schedules an update to a component’s state
object. When state changes, the component responds by re-rendering.
Here setState method is called to reset the state of Todo Component which has mockData. It simple appends new item taken from the input field. Finally, set the value of the input field to empty.


Go ahead and refresh the app in your browser and type “Hike time” or anything you want and press ADD button. You should be able to see the new item at the bottom of the list like above.
Now that C is done, time for D which is Delete. Simple add onDeleteHandle method to Todo component like below.
onDeleteHandle() { let id = arguments[0];
this.setState({ mockData: this.state.mockData.filter(item => { if (item.id !== id) { return item; } }) }); }
This method is triggered when delete button is clicked as you can see we are binding this and item.id to onDeleteHandle . this keyword is necessary so that we have access to current scope to access the state of Todo Component with this keyword whereas the id part is used to delete that particular item. In order access the item.id, we are going to use arguments[0] object. Once we have the id. Then set the state and filter through mockData and find the the item that needs to be deleted and return all the item except the one that needs to be deleted.
Go ahead and refresh your browser and press delete on first item and you should see that it is delete like below screenshot.


That’s all for the delete part. Update part as usual consist of 2 parts. First show the edit form when edit button is pressed then perform update operation.
To show and hide edit form we are going to use edit property we added to state. So add below renderEditForm method to the component.
renderEditForm() { if (this.state.edit) { return <form onSubmit={this.onUpdateHandle.bind(this)}> <input type="text" name="updatedItem" className="item" defaultValue={this.state.title} /> <button className="update-add-item">Update</button> </form> } }
What it does is that it checks the edit state and based on that it returns editForm which is JSX syntax of form.
Now call above method in render method inside return keyword just above current form like below:
{this.renderEditForm()}
Now that part is out of our way, time to manipulate the edit property. Add below onEditHandle method to Todo Component:
onEditHandle(event) { this.setState({ edit: true, id: arguments[0], title: arguments[1] }); }
This method is triggered when Edit button is pressed. We are binding three parameters i.e. this , id , and title . this keyword is used to reference the current component and set the id property to id of the current item being edited and edit to true and add title property to the state which we will access later in this component. Now that once we have this code in our component. Go to browser, refresh and click on edit button for the first item which will show edit form. Like below:


This form has an input field and update button. Now its time to handle the U part of CRUD. When UPDATE button, in edit form shown above, is pressed below method will be triggered:
onUpdateHandle(event) { event.preventDefault();
this.setState({ mockData: this.state.mockData.map(item => { if (item.id === this.state.id) { item['title'] = event.target.updatedItem.value; return item; }
return item; }) });
this.setState({ edit: false }); }
Add above method to your Todo Component. This set the state of the component, map through mockData inside the state and find the item that need to be updated and set its title with the new title. Finally, set edit property of the state to false to hide the form. That is it. Now run the your code in your browser and try to update first item you should be able to see updated title.


Final method is used to set the item to complete state. Add below method which does exactly that.
onCompleteHandle() { let id = arguments[0];
this.setState({ mockData: this.state.mockData.map(item => { if (item.id === id) { item['done'] = true; return item; }
return item; }) }); }
Above method sets done property of the item in mockData to true. This is pretty much the same as in our previous two example in Vanilla JavaScript and Angular blogs.
Now to make this work, add below code to “li” to set its class based on “done” property state in mockData.
className={ item.done ? 'done' : 'hidden' }
Now refresh your browser and press complete button you should be able to see below changes.


Below is basic CSS that needs to be added to index.css file on order to display done items on the screen.
.done { text-decoration: line-through; }
That is it. As you can see that reactjs is even more component centric solution to modern javascript apps. Next task for you would be to split the form element into its own components so that we do not need to use two form elements for update and add operation. That should be simple and fun task for everyone.
To get complete code clone below repository.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK