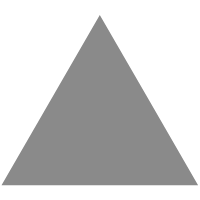
41
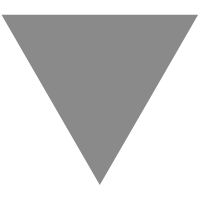
二叉树的基本运算2
source link: https://studygolang.com/articles/18195?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
这一篇是接上一篇文章 二叉树的基本运算
二叉树的遍历
二叉树遍历分为三种:前序、中序、后序:
- 前序遍历:根结点 -> 左子树 -> 右子树
- 中序遍历:左子树 -> 根结点 -> 右子树
- 后序遍历:左子树 -> 右子树 -> 根结点
另外还有一种 层次遍历 ,即每一层都从左向右遍历。
譬如,对于下面的二叉树
前序遍历:abdefgc
中序遍历:debgfac
后序遍历:edgfbca
层次遍历:abcdfeg
实现方法
因为树的定义本身就是递归定义,因此采用递归的方法去实现树的三种遍历不仅容易理解而且代码很简洁。而对于树的遍历若采用非递归的方法,就要采用 栈 去模拟实现。在三种遍历中,前序和中序遍历的非递归算法都很容易实现, 非递归后序遍历 实现起来相对来说要难一点
中序遍历
go实现
// 中序遍历,用栈实现 func inOrderBinaryTree1(root *BinaryTreeNode) { if root == nil { return } stack := []*BinaryTreeNode{} top := -1 for top >= 0 || root != nil { for root != nil { stack = append(stack, root) top++ root = root.lChild } item := stack[top] stack = stack[:top] top-- // 出栈 fmt.Print(item.data) if item.rChild != nil { root = item.rChild } } }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK