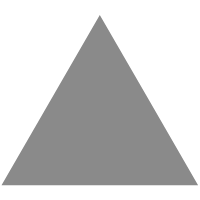
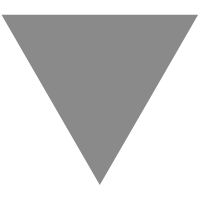
Must know concepts of React Router
source link: https://www.tuicool.com/articles/hit/UremYfm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Passing props to a Component
Suppose, you have some data in your parent component that you have to pass to a child component through a Route. The incorrect way to do this would be like:


Route doesn’t provide ‘ someData’ prop to the Pokemon Component, as React Router just ignores unknown props. So, how to pass props? One way to do would be to pass the component prop an inline function.


The above method works just fine, but has some gotchas to it and will lead to performance issues as mentioned in the docs. According to the react router documentation:-
When you use component, the router uses React.createElement to create a new React element from the given component. That means if you provide an inline function to the component prop, you would create a new component every render. This results in the existing component unmounting and the new component mounting instead of just updating the existing component.
So, what’s the proper way to do this ?? Luckily, React Router provides us with a render prop❤.


Simple, right? Using render prop is the suggested way of passing props with no performance issues as per the official docs.
Navigate Programmatically
Sometimes we want to navigate to a different route when a certain event happens or when a user performs some action. To handle this condition, I used to use history prop provided by the React Router.


After reading Tyler McGinnis article , I realized that this approach is not the best approach out there. One of the major issue related to this approach is, that this pattern works only when your component is rendered by React Router. And here is why, If your component is not rendered by React Router then the respective component doesn’t have access to the history props. One way to use history prop in a non router rendered component is given below


withRouteris a Higher Order Component which provides the respective component with the history prop . But again this approach is not very “Reactive”. Tyler McGinnis in his article mentioned
If you broke React down into three core principles, you’d get component composition, declarative UI, and state management, specifically, user event -> state change -> re-render. Because React Router’s vision is aligned with React’s, programmatically navigating with React Router should, by definition, align with those three core concepts.
And he proposed the best way to do this is using <Redirect />.


This method works in the right order and abides by the core react principles mentioned above. First, the event occurs(form submission), which leads to change in state, and change in the state leads to re-render of the component.
Protected Routes
You don’t want an unauthenticated user to access some protected component or data. So basically, how would you protect your component? You will make sure that when the unauthenticated user visits the protected component’s path, you don’t render it. Instead, redirect it to some other component. And here’s how you will achieve this


Above is a ‘ PrivateRoute ’ Component, which takes the protected component as props and renders it, if the user is authenticated, else it will redirect the user to the login page. And this component can be used for every component you want to protect.


Using a route config file
In your App component, you can have many Route components, which can make the App.js file messy. To overcome this situation, I store all my route data in an object array in a different file.


And map through the array in the App component:-


If you are interested, that’s how the nested routes would work in the above case. Please check out this video .
Conclusion
In this article, we learned about some cool stuff related to React Router. Though there are a lot more cool concepts which I’ll cover in my upcoming articles. Hope you liked this article, Please feel free to comment and ask anything. Thanks for reading :pray: :sparkling_heart:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK