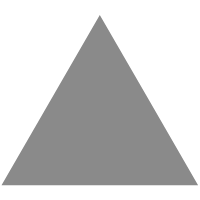
21
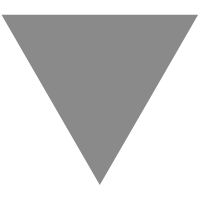
学习Golang的HTTP中间件机制
source link: https://huoding.com/2019/01/31/716?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
因为 Golang 内置的 net/http 天生就支持 HTTP 中间件机制,所以即便不用 gin 之类的 Web 框架,我们也可以写出扩展性很好的 Web 应用。
假如你不了解 Golang 的 HTTP 中间件机制的话,那么可以把它看成是一个洋葱:

通过洋葱看中间件
每一个中间件都是一层洋葱皮,其中每一个中间件都可以改变请求和响应,我们可以很自然的把不同的业务逻辑放到不同的洋葱皮里,下面看看例子:
package main import ( "net/http" ) func foo(next http.HandlerFunc) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("foo(")) next(w, r) w.Write([]byte(")")) } } func bar(next http.HandlerFunc) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("bar(")) next(w, r) w.Write([]byte(")")) } } func test(w http.ResponseWriter, r *http.Request) { w.Write([]byte("test")) } func main() { http.Handle("/test", foo(bar(test))) http.ListenAndServe(":8080", nil) }
运行结果显示如下,它形象的说明了中间件的执行过程:
foo(bar(test))
联想一下洋葱的结构,基本就能明白 Golang 的 HTTP 中间件机制了,不过不爽的是构建方式不易扩展,假如中间件很多的话,视觉上会呈现出复杂的嵌套,比如:
middleware(middleware(middleware(middleware(handler))))
我可不想维护这样的代码,下面看看如何简化编码方式:
func main() { http.Handle("/test", new(foo).pipe(bar).process(test)) http.ListenAndServe(":8080", nil) } type middleware func(http.HandlerFunc) http.HandlerFunc type pipeline struct { middlewares []middleware } func new(ms ...middleware) pipeline { return pipeline{append([]middleware(nil), ms...)} } func (p pipeline) pipe(ms ...middleware) pipeline { return pipeline{append(p.middlewares, ms...)} } func (p pipeline) process(h http.HandlerFunc) http.HandlerFunc { for i := range p.middlewares { h = p.middlewares[len(p.middlewares)-1-i](h) } return h }
对比一下在修改代码前后调用方式发生了什么改变,:
- 修改前:foo(bar(test))
- 修改后:new(foo).pipe(bar).process(test)
虽然表面上看好像代码更长了,但是对代码的可维护性而言,这并不是问题的关键,重要的是通过使用 Pipeline 模式,我们把原本嵌套结构改成了链式结构。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK