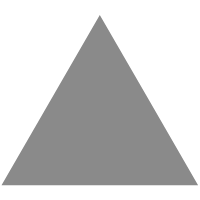
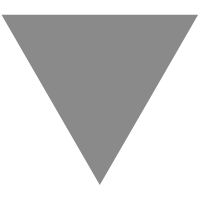
Context Menu with Table View iOS Tutorial
source link: https://www.tuicool.com/articles/hit/63q2aqr
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A long-press gesture displays a Context Menu, which gives the user the ability to use cut/copy/paste operations on the selected object. By default, the Context Menu is disabled on a Table View. In this tutorial the context menu will be enabled to copy the text of the Table View Cell, this text can then be pasted into a Text Field. This tutorial is made with Xcode 10 and built for iOS 12.
Open Xcode and create a new Single View App.

For product name, use IOSContextMenuTableViewTutorial and then fill out the Organization Name and Organization Identifier with your customary values. Enter Swift as Language and choose Next.

Open The Main.storyboard file and drag a Table View from the Object Library to the top of the main View. Select the Table View, go to the Attribute Inspector and in the Table View section change the Prototype Cells field to a value of 1

Select the Table View Cell and go to the Attribute Inspector. In the Table View Cell section set the Identifier to "cell".

Select the Table View, select the Pin button on the bottom-right of the storyboard and pin the Table View on the top, left and right. Also select the Height Attribute to give the Table View a fixed height. Select Items of New Constraints at the Update Frames dropdown box. Next, select Add 4 Constraints.

Drag a Text Field from the Object Library and place it right below the Table View. Ctrl and drag from inside the Text Field to the Table View. Hold Down Shift and select "Vertical Spacing" and "Center Horizontally".

Select the Text Field, select the Pin button on the bottom-right of the storyboard and pin the Text Field to the left and right.

The View Controller needs to be the delegate of the Table View. Select the TableView, Ctrl and Drag to the View Controller icon at the top of the main View. Select Datasource. Repeat this step and select delegate.

Repeat this also for the Text Field to make the View Controller the delegate for it. Go to the ViewController.swift file and change the class declaration line to
class ViewController: UIViewController, UITableViewDelegate, UITableViewDataSource, UITextFieldDelegate {
Add the following properties
var pasteBoard = UIPasteboard.general var tableData = ["dog","cat","fish"] }
The pasteBoard property will be used for copy-paste operations and the tableData holds the contents which will be displayed on the Table View Cells. Next, change the Table View delegate methods.
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { return tableData.count } func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) cell.textLabel?.text = tableData[indexPath.row] return cell }
The Table View will be filled with the three values from the TableData array. To enable the Context Menu the following three delegate methods must be implemented.
func tableView(_ tableView: UITableView, shouldShowMenuForRowAt indexPath: IndexPath) -> Bool { return true } func tableView(_ tableView: UITableView, canPerformAction action: Selector, forRowAt indexPath: IndexPath, withSender sender: Any?) -> Bool { if (action == #selector(UIResponderStandardEditActions.copy(_:))) { return true } return false } func tableView(_ tableView: UITableView, performAction action: Selector, forRowAt indexPath: IndexPath, withSender sender: Any?) { let cell = tableView.cellForRow(at: indexPath) pasteBoard.string = cell!.textLabel?.text }
The tableView: shouldShowMenuForRowAt method must return true to display the Context Menu when a Table View Cell is long-pressed. In the tableView:canPerformAction:forRowAt method only the copy item is displayed. The tableView:performAction:forRowAt:withSender method copies the selected text into the pasteBoard.
Finally, implement the textFieldShouldReturn method to dismiss the keyboard when pressing enter when in the editing mode in the Text Field.
func textFieldShouldReturn(_ textField: UITextField) -> Bool { self.view.endEditing(true) return false }
Build and Runthe project, Long-press a Table View Cell and Select the copy item. Paste the text inside the Text Field.

You can download the source code of the IOSContextMenuTableViewTutorial at the ioscreator repository on Github .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK