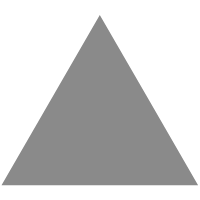
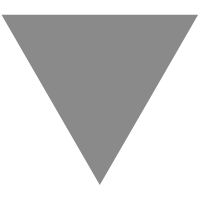
Python之在函数中使用列表作为默认参数
source link: https://segmentfault.com/a/1190000018034734?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
本文将介绍笔者在工作中遇到的Python的一个坑,那就是使用列表作为默认参数。
我们知道,在Python中,列表(list)是可变对象,所以列表的内容可能会在函数内改变。另一个需要注意的是,使用列表作为函数的默认参数时,列表的内容变化情况。
首先,我们看以下的代码例子:
def add(x, lst=[]): if x not in lst: lst.append(x) return lst def main(): list1 = add(1) print(list1) list2 = add(2) print(list2) list3 = add(3, [11, 12, 13, 14]) print(list3) list4 = add(4) print(list4) main()
你可能以为输出的结果会是:
[1] [2] [11, 12, 13, 14, 3] [4]
但事实上,该程序输出的结果是:
[1] [1, 2] [11, 12, 13, 14, 3] [1, 2, 4]
这是为什么呢?函数add的功能是当x不在列表中时,将x追加给列表lst。当函数第一次执行时,参数lst的默认值[]被创建。这个默认值只会被创建一次。add(1)将1加到lst。当函数再次被调用时,lst是[1]而不是[],因为lst只被创建一次。当参数的lst为[11,12,13,14]时,lst就是[11,12,13,14]。list4调用函数时,使用默认参数,因此,现在默认参数lst为[1,2]。
为了更好地理解调用情况,可以在add函数中输出lst的id,如以下代码:
def add(x, lst=[]): print(id(lst)) if x not in lst: lst.append(x) return lst def main(): list1 = add(1) print(list1) list2 = add(2) print(list2) list3 = add(3, [11, 12, 13, 14]) print(list3) list4 = add(4) print(list4) main()
输出结果如下:
4469603648 [1] 4469603648 [1, 2] 4469670472 [11, 12, 13, 14, 3] 4469603648 [1, 2, 4]
可以看到,list1, list2, list4调用时默认参数的id并没有发生改变,而list3的id发生了改变。
这是Python使用列表作为默认参数的一个坑。那么,如何避免踩坑呢?如果想要使用默认列表在每次函数调用时都是[],可以像下面的程序那样修改函数参数:
def add(x, lst=None): if lst is None: lst = [] if x not in lst: lst.append(x) return lst def main(): list1 = add(1) print(list1) list2 = add(2) print(list2) list3 = add(3, [11, 12, 13, 14]) print(list3) list4 = add(4) print(list4) main()
输出的结果如下:
[1] [2] [11, 12, 13, 14, 3] [4]
本次分享到此结束,感谢阅读~
注意:本人现已开通微信公众号: Python爬虫与算法(微信号为:easy_web_scrape), 欢迎大家关注哦~~
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK