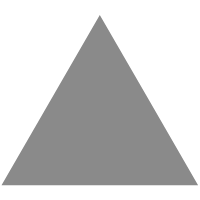
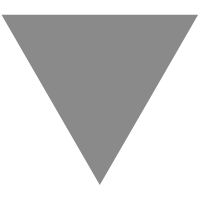
Use MongoDB Node.js Native Driver Without Mongoose
source link: https://www.tuicool.com/articles/hit/UFFZRbf
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
MongoDB is a popular NoSQL database. It is a document database that stores data in flexible, JSON-like documents. It is published under AGPL.
MongoDB is a distributed database at its core, so it's high availability, horizontal scaling, and geographic distribution are built in and easy to use.
This post is a simple article on how to access MongoDB through the Mongo Node.js Driver 2.4. The Node.js driver provides both callback-based and promise-based operations. The complete API reference available on the MongoDB site .
Using Mongoose to access MongoDB will enforce strict schema and performance overhead on our development efforts. It breaks the performance or flexibility that MongoDB provides. Instead, we can try to use the Mongo Node.js driver which gives all the performance benefits of Mongo. It is also flexible to add any property on the fly without any code changes, e.g. ensuring that product metadata has different keys.
As a novice JavaScript developer, I started creating basic operations with promise and await and also used callback options without any help from any libraries.
Let's start by creating a project with npm init
and install the MongoDB driver.
npm install [email protected] --save
The MongoDao JS file which interacts with and connects to MongoDB performs the database operations, does the error handling, and then does the callback operations.
The app.js file, which is the client to the dao file, will send/get the data, invoke the DB operations, and wire all the operations in line to do it.
The app file first creates the MongoDao
object with the URL and then connects asynchronously. After a successful connection, it connects to the requested database name.
function MongoDao(mongoUri, dbname) { var _this = this; var options = { useNewUrlParser: true }; _this.mongoClient = new MongoClient(mongoUri, options); return new Promise(function(resolve, reject) { _this.mongoClient.connect(function(err, client) { assert.equal(err, null); console.log("mongo client successfully connected \n"); _this.dbConnection = _this.mongoClient.db(dbname); resolve(_this); }); }); }
This asynchronous operation is wrapped in a promise which is executed synchronously by await,
which then waits
for this promise to be fulfilled, like below.
async function main() { mongoDao = await new MongoDao(url, dbName); insertFn(); } main();
The above code also invokes an insert function which passes the collection name and document to mongoDao
.
const insertFn = function() { //insert and then inserted record will be read mongoDao.insertDocument(collectionName, doc, updateFn); }
The insert function in MongoDao will insert the document with the insertOne
Mongo Driver method and then, through the callback, it retrieves the same document and prints it.
MongoDao.prototype.insertDocument = function(collectionName, doc, callback) { var _this = this; this.dbConnection.collection(collectionName).insertOne(doc, function(err, result) { assert.equal(null, err); console.log(" Below doc inserted successfully"); _this.printDocument(collectionName, doc, callback); }); }
The printDocument
method is a Dao method which retrieves the documents and filter based on the doc JSON passed. It will print the record and invoke the callback, which is a updaten function ( mongoDao.insertDocument(collectionName, doc, updateFn);
).
MongoDao.prototype.printDocument = function(collectionName, doc, callback) { this.dbConnection.collection(collectionName).find({}).filter(doc) .toArray(function(err, docs) { console.log(docs[0]); console.log("\n"); callback(); }); }
Tghe insert function does the insert and then invokes the callback provided, to which I have added an update function to update the inserted doc itself.
const updateFn = function() { var updateDoc = { "$set": { "mobile": 9629230494 } }; mongoDao.updateDocument(collectionName, doc, updateDoc, deleteFn); }
In MongoDao, this will invoke the updateOne
API method to perform this operation and check for the error and then print the number of records updated.
MongoDao.prototype.updateDocument = function(collectionName, doc, updateDocument, callback) { var _this = this; this.dbConnection.collection(collectionName).updateMany(doc, updateDocument, function(err, result) { assert.equal(null, err); console.log(result.result.ok + " document updated successfully"); _this.printDocument(collectionName, doc, callback); callback(); }); }
After the document has successfully been updated, it invokes the delete function as shown above ( mongoDao.updateDocument(collectionName, doc, updateDoc, deleteFn);
).
In the delete function, the document with key values is used to locate the document passed as parameters, so the deleteOne
API method will be used to delete it.
MongoDao.prototype.deleteDocument = function(collectionName, doc, callback) { this.dbConnection.collection(collectionName).deleteOne(doc, function(err, result) { assert.equal(null, err); console.log(result.result.ok + " document deleted successfully"); callback(); }); }
The client part of calling this Dao delete method is obvious so it has been skipped.
The working code is available at the below git path.
https://github.com/nagappan080810/nodejsmongodb
It also contains other files, so please look at the below two files for these operations.
-
mongodao.js - All the database operations, error handling, and invoking of call back functions.
-
app.js - Client for the mongodao.js which passes the data and retrieves the data.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK