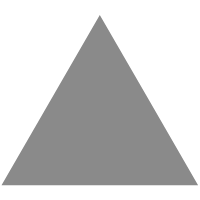
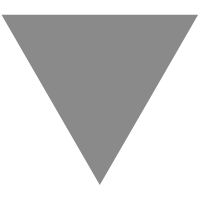
GitHub - statsbotco/cubejs-client: ? Serverless Analytics Framework
source link: https://github.com/statsbotco/cubejs-client
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Website • Blog • Slack • Twitter
Cube.js is an analytics framework for modern applications. It supplies building blocks that let developers build custom and large-scale analytics frontends without spending the time on a complex analytics backend infrastructure.
- Cube.js is visualization agnostic. It means you can use your favorite chart library, like Chart.js, Recharts, C3.js or any other.
- Cube.js Data Schema works as an ORM for your analytics. It allows to model everything from simple counts to cohort retention and funnel analysis.
- It is designed to work on top of your database, so all your data stays with you. All major SQL databases are supported.
This repository contains Cube.js Javascript and React clients. The Cube.js Server itself is not yet open-sourced. We are working hard to make it happen.
Contents
Examples
Demo Code Description Examples Gallery examples-gallery Examples Gallery with different visualizations libraries Stripe Dashboard stripe-dashboard Stripe Demo Dashboard built with Cube.js and Recharts AWS Web Analytics aws-web-analytics Web Analytics with AWS Lambda, Athena, Kinesis and Cube.jsTutorials
- Building a Serverless Stripe Analytics Dashboard
- Building E-commerce Analytics React Dashboard with Cube.js and Flatlogic
- Building Open Source Google Analytics from Scratch
Architecture
Cube.js acts as an analytics backend, taking care of translating business logic into SQL and handling database connection.
The Cube.js javascript Client performs queries, expressed via dimensions, measures, and filters. The Server uses Cube.js Schema to generate a SQL code, which is executed by your database. The Server handles all the database connection, as well as pre-aggregations and caching layers. The result then sent back to the Client. The Client itself is visualization agnostic and works well with any chart library.
Getting Started
1. Create Free Statsbot Account
Cube.js Cloud is provided by Statsbot, you can sign up for a free account here.
2. Connect Your Database
All major SQL databases are supported. Here the guide on how to connect your database to Statsbot.
3. Define Your Data Schema
Cube.js uses Data Schema to generate and execute SQL. It acts as an ORM for your analytics and it is flixible enough to model everything from simple counts to cohort retention and funnel analysis. Read Cube.js Schema docs.
4. Visualize Results
Generate a Cube.js token within Statsbot UI. 1. Go to Data Sources2. Click Edit next to your database
3. Select Cube.js API
Now you are ready to use this library to add analytics features to your app.
Installation
Vanilla JS:
$ npm i --save @cubejs-client/core
React:
$ npm i --save @cubejs-client/core $ npm i --save @cubejs-client/react
Example Usage
Vanilla Javascript.
Instantiate Cube.js API and then use it to fetch data:
const cubejsApi = cubejs('eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpIjozODU5NH0.5wEbQo-VG2DEjR2nBpRpoJeIcE_oJqnrm78yUo9lasw'); const resultSet = await cubejsApi.load({ measures: ['Stories.count'], timeDimensions: [{ dimension: 'Stories.time', dateRange: ['2015-01-01', '2015-12-31'], granularity: 'month' }] }) const context = document.getElementById("myChart"); new Chart(context, chartjsConfig(resultSet));
Using React
Import cubejs
and QueryRenderer
components, and use them to fetch the data.
In the example below we use Recharts to visualize data.
import React from 'react'; import { LineChart, Line, XAxis, YAxis } from 'recharts'; import cubejs from '@cubejs-client/core'; import { QueryRenderer } from '@cubejs-client/react'; const cubejsApi = cubejs('eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpIjozODU5NH0.5wEbQo-VG2DEjR2nBpRpoJeIcE_oJqnrm78yUo9lasw'); export default () => { return ( <QueryRenderer query={{ measures: ['Stories.count'], dimensions: ['Stories.time.month'] }} cubejsApi={cubejsApi} render={({ resultSet }) => { if (!resultSet) { return 'Loading...'; } return ( <LineChart data={resultSet.rawData()}> <XAxis dataKey="Stories.time"/> <YAxis/> <Line type="monotone" dataKey="Stories.count" stroke="#8884d8"/> </LineChart> ); }} /> ) }
Security
Cube.js can be used in two modes:
- Without security context. It implies same data access permissions for all users.
- With security context. User or role-based security models can be implemented using this approach.
You're provided with two types of security credentials:
- Cube.js Global Token. Has format like
eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpIjozODU5NH0.5wEbQo-VG2DEjR2nBpRpoJeIcE_oJqnrm78yUo9lasw
. Can be passed tocubejs()
. - Cube.js Secret. Has format like
cjs_38594_sPEWwPkVtTEEjTs9AkpicdUcw26R58ueo2G4rRZ-Wyc
. Should be used to sign JWT tokens passed tocubejs()
.
NOTE: Cube.js Global Token should be used only for publicly available data or for testing purposes. For production use please generate expirable tokens using Cube.js Secret.
Cube.js tokens used to access an API are in fact JWT tokens.
Cube.js Global Token is not an exception and generated for your convenience.
Cube.js Global Token is JWT token signed with Cube.js Secret that has minimal payload like { i: 38594 }
.
Cube.js Global Token provides no security context so it has all possible rights for querying.
Besides Cube.js Global Token you can use Cube.js Secret to generate customer API tokens to restrict data available for querying on your server side and embed it in web page that renders query results.
For example to generate customer API tokens with cjs_38594_sPEWwPkVtTEEjTs9AkpicdUcw26R58ueo2G4rRZ-Wyc
secret you should provide minimal payload { i: 38594 }
which is your key identifier required for token verification.
Security context can be provided by passing u
param for payload.
For example if you want to pass user id in security context you can create token with payload:
{ "i": 38594, "u": { "id": 42 } }
In this case { id: 42 }
object will be accessible as USER_CONTEXT
in cube.js Data Schema.
Learn more: Data Schema docs.
NOTE: We strongly encourage you to use
exp
expiration claim to limit life time of your public tokens. Learn more: JWT docs.
API
cubejs(apiKey)
Create instance of CubejsApi
.
apiKey
- API key used to authorize requests and determine SQL database you're accessing. To get a key signup for here here.
CubejsApi.load(query, options, callback)
Fetch data for passed query
. Returns promise for ResultSet
if callback
isn't passed.
query
- analytic query. Learn more about it's format below.options
- options object. Can be omitted.progressCallback(ProgressResult)
- pass function to receive real time query execution progress.
callback(err, ResultSet)
- result callback. If not passedload()
will return promise.
QueryRenderer
<QueryRenderer />
React component takes a query, fetches the given query, and uses the render prop to render the resulting data.
Properties:
query
: analytic query. Learn more about it's format below.cubejsApi
:CubejsApi
instance to use.render({ resultSet, error, loadingState })
: output of this function will be rendered byQueryRenderer
.resultSet
: AresultSet
is an object containing data obtained from the query. If this object is not defined, it means that the data is still being fetched.ResultSet
object provides a convient interface for data munipulation.error
: Error will be defined if an error has occurred while fetching the query.loadingState
: Provides information about the state of the query loading.
ResultSet.rawData()
Returns query result raw data returned from server in format
[ { "Stories.time":"2015-01-01T00:00:00", "Stories.count": 27120 }, { "Stories.time":"2015-02-01T00:00:00", "Stories.count": 25861 }, { "Stories.time":"2015-03-01T00:00:00", "Stories.count": 29661 }, //... ]
Format of this data may change over time.
Query Format
Query is plain JavaScript object, describing an analytics query. The basic elements of query (query members) are measures
, dimensions
, and segments
. You can learn more about Cube.js Data Schema here.
The query member format name is CUBE_NAME.MEMBER_NAME
, for example dimension email in the Cube Users would have the following name Users.email
.
Query has following properties:
measures
: An array of measures.dimensions
: An array of dimensions.filters
: An array of filters.timeDimensions
: A convient way to specify a time dimension with a filter. It is an array of objects with following keysdimension
: Time dimension name.dateRange
: An array of dates with following format '2015-01-01', if only one date specified the filter would be set exactly to this date.granularity
: A granularity for a time dimension, supports following valuesday|week|month|year
.
segments
: An array of segments. Segment is a named filter, created in the Data Schema.limit
: A row limit for your query. The hard limit is set to 5000 rows by default.
{ measures: ['Stories.count'], dimensions: ['Stories.category'], filters: [{ dimension: 'Stories.dead', operator: 'equals', values: ['No'] }], timeDimensions: [{ dimension: 'Stories.time', dateRange: ['2015-01-01', '2015-12-31'], granularity: 'month' }], limit: 100 }
License
Cube.js Client is MIT licensed.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK