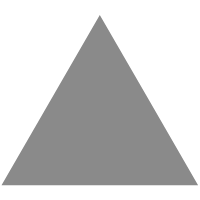
35
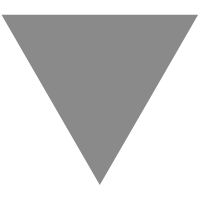
Golang学习笔记之结构体(struct)上
source link: https://studygolang.com/articles/16713?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
结构体(struct)是用户自定义的类型,它代表若干字段的集合。
• 值类型,赋值和传参会复制全部内容。可⽤ "_" 定义补位字段,⽀持指向⾃⾝类型的指针成员。
•结构体内字段⽀持 "=="、 "!=", 结构体就支持相应操作
•可⽤作 map 键类型。
一、定义
//包内使用首字母小写,包外使用首字母大写 type employee struct { firstName string lastName string age int }
二、初始化的几种方式
//初始化方式1 var emp1 employee emp1.firstName = "hello" emp1.lastName = "world" emp1.age = 20 fmt.Println(emp1) //初始化方式2利用键值方式 emp2 := employee{ firstName: "Sam", age: 25, lastName: "Anderson", //使用键值方式这个逗号不可以省略 } fmt.Println(emp2) //初始化方式3顺序初始化,必须包含全部字段,否则会出错。 emp3 := employee{"Thomas", "Paul", 29} fmt.Println(emp3)
三、支持匿名结构
//匿名结构,可⽤作结构成员或定义变量。 type file struct { name string size int attr struct { perm int owner int } } func main() { //匿名结构 f := file{ name: "test.txt", size: 1025, // attr: {0755, 1}, //组合文字中缺少类型 } f.attr.owner = 1 f.attr.perm = 0755 var attr = struct { perm int owner int }{2, 0755} f.attr = attr fmt.Println(f.attr.perm) }
四、匿名字段
•通俗来说是⼀个与成员类型同名 (不含包名) 的字段。
•被匿名嵌⼊的可以是任何类型,包括指针。
•不能同时嵌⼊某⼀类型和其指针类型,因为它们名字相同。
•匿名字段可以字段提升。可以像普通字段那样访问匿名字段成员,编译器从外向内逐级查找所有层次的匿名字段,直到发现目标或出错。但结构体匿名字段外层同名字段会遮蔽嵌⼊字段成员
//匿名字段 type person struct { string int employee } func main(){ //匿名字段会把类型用作变量名 p := person{"Hello", 20, emp1} p.string = "Other" fmt.Println(p, p.string) //{Other 20} Other fmt.Println(p.lastName)//等价于p.employee.lastName//输出:hello }
五、结构体嵌套
//结构体嵌套 type address struct { city, state string } type student struct { name string age int address address } func main(){ //结构体嵌套 var s student s.name = "小明" s.age = 18 s.address = address{ city: "北京", state: "中国", } fmt.Println(s) fmt.Println(s.name) fmt.Println(s.address.city) }
完整代码实例
package main import ( "fmt" ) //需要添加注释或者改成非导出变量(首字母小写) type employee struct { firstName string lastName string age int } //匿名字段 type person struct { string int employee } //结构体嵌套 type address struct { city, state string } type student struct { name string age int address address } //匿名结构,可⽤作结构成员或定义变量。 type file struct { name string size int attr struct { perm int owner int } } func main() { //初始化方式1 var emp1 employee emp1.firstName = "hello" emp1.lastName = "world" emp1.age = 20 fmt.Println(emp1) //初始化方式2利用键值方式 emp2 := employee{ firstName: "Sam", age: 25, lastName: "Anderson", //使用键值方式这个逗号不可以省略 } fmt.Println(emp2) //初始化方式3顺序初始化,必须包含全部字段,否则会出错。 emp3 := employee{"Thomas", "Paul", 29} fmt.Println(emp3) //结构体指针 emp5 := &employee{"Sam", "Anderson", 55} fmt.Println("First Name:", (*emp5).firstName) fmt.Println("Age:", (*emp5).age) //匿名字段会把类型用作变量名 p := person{"Hello", 20, emp1} p.string = "Other" fmt.Println(p, p.string) //{Other 20} Other fmt.Println(p.lastName)//等价于p.employee.lastName//输出:hello //匿名结构 f := file{ name: "test.txt", size: 1025, // attr: {0755, 1}, //组合文字中缺少类型 } f.attr.owner = 1 f.attr.perm = 0755 var attr = struct { perm int owner int }{2, 0755} f.attr = attr fmt.Println(f.attr.perm) //结构体嵌套 var s student s.name = "小明" s.age = 18 s.address = address{ city: "北京", state: "中国", } fmt.Println(s) fmt.Println(s.name) fmt.Println(s.address.city) }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK