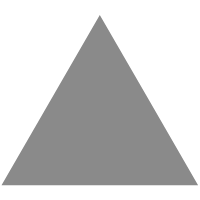
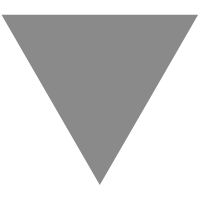
GitHub - Awalz/SwiftyDraw: A simple, lightweight drawing framework written in Sw...
source link: https://github.com/Awalz/SwiftyDraw
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
SwiftyDraw
Overview
SwiftyDraw is a simple, light-weight drawing framework written in Swift. SwiftyDraw is built using Core Gaphics and is very easy to implement.
Requirements
- iOS 9.1+
- Swift 4
Installation
Cocoapods:
SwiftyDraw is available through CocoaPods. To install it, simply add the following line to your Podfile:
pod 'SwiftyDraw'
Manual Installation:
Simply copy the contents of the Source folder into your project.
Usage
Using SwiftyDraw is very simple:
Getting Started:
Simply create a SwiftyDrawView and add it to your View Controller:
let drawView = SwiftyDrawView(frame: self.view.frame) self.view.addSubview(drawView)
By default, the view will automatically respond to touch gestures and begin drawing. The default color is black.
To disable drawing, simply set the isEnabled
property to false:
drawView.isEnabled = false
Brushes
For drawing, we use Brush
to keep track of styles like width
, color
, etc.. We have multiple different default brushes, you can use as follows:
drawView.brush = Brush.default
The default brushed are:
public static var `default`: Brush { get } // black, width 3 public static var thin : Brush { get } // black, width 2 public static var medium : Brush { get } // black, width 7 public static var thick : Brush { get } // black, width 10 public static var marker : Brush { get } // flat red-ish, width 12 public static var eraser : Brush { get } // white, width 8; currently this fakes an eraser by using the canvas' background color to draw
Adjusted Width Factor
SwiftyDrawView
supports drawing-angle-adjusted brushes. Effectively, that means, if the user (using an Pencil) draws with the tip of the pencil, the brush will reduce its width a little. If the user draws at a very low angle, with the side of the pencil, the brush will be a little thicker.
You can modify this behavior by setting adjustedWidthFactor
of a brush. If you increase the number (to, say, 5
) the changes will increase. If you reduce the number to 0
, the width will not be adjusted at all.
The default value is 1
which causes a slight increase in width.
This is an opt-in feature. That means, in shouldBeginDrawingIn
, you need to manually put drawingView.brush.adjustWidth(for: touch)
, to make it work.
That is, because you might not want to use it if you have implemented a zoom feature and want to disable it while the user is zooming to get better results.
Further Customization:
For more customization, you can modify the different properties of a brush to fit your needs.
Line Color:
The color of a line stroke can be changed by adjusting the color
property of a brush. SwiftyDraw accepts any UIColor:
drawView.brush.color = .red
or
drawView.brush.color = UIColor(colorLiteralRed: 0.75, green: 0.50, blue: 0.88, alpha: 1.0)
Line Width:
The width of a line stroke can be changed by adjusting the width
property of a brush. SwiftyDraw accepts any positive CGFloat:
drawView.brush.width = CGFloat(5.0)
Line Opacity:
The opacity of a line stroke can be changed by adjusting the lineOpacity
property. SwiftyDraw accepts a CGFloat between 0. and 1.0:
drawView.brush.opacity = CGFloat(0.5)
Editing
Clear All:
If you wish to clear the entire canvas, simply call the clear
function:
drawView.clear()
Drawing History:
drawView.undo()
...and redo:
drawView.redo()
Delegate
SwiftyDraw has delegate functions to notify you when a user is interacting with a SwiftDrawView. To access these delegate methods, simply make your View Controller conform to the SwiftyDrawViewDelegate
protocol:
class ViewController: UIViewController, SwiftyDrawViewDelegate
There are five delegate methods
/// SwiftyDrawViewDelegate called when a touch gesture should begin on the SwiftyDrawView using given touch type func swiftyDraw(shouldBeginDrawingIn drawingView: SwiftyDrawView, using touch: UITouch) -> Bool /// SwiftyDrawViewDelegate called when a touch gesture begins on the SwiftyDrawView. func swiftyDraw(didBeginDrawingIn drawingView: SwiftyDrawView, using touch: UITouch) /// SwiftyDrawViewDelegate called when touch gestures continue on the SwiftyDrawView. func swiftyDraw(isDrawingIn drawingView: SwiftyDrawView, using touch: UITouch) /// SwiftyDrawViewDelegate called when touches gestures finish on the SwiftyDrawView. func swiftyDraw(didFinishDrawingIn drawingView: SwiftyDrawView, using touch: UITouch) /// SwiftyDrawViewDelegate called when there is an issue registering touch gestures on the SwiftyDrawView. func swiftyDraw(didCancelDrawingIn drawingView: SwiftyDrawView, using touch: UITouch)
Contribution
This is a project built by Awalz and maintained & improved by LinusGeffarth.
If you would like to propose any enhancements, bug fixes, etc., feel free to create a pull request or an issue respectively.
Contact
If you have any questions, or just want to say hi, you can reach out to me via Twitter, or email.
LICENSE
SwiftyDraw is available under the MIT license. See the LICENSE file for more info.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK