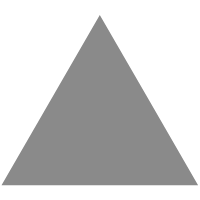
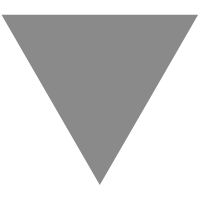
Android Better Logging using Timber Library
source link: https://www.tuicool.com/articles/hit/RZrAbm7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Timber is a logging utility class built on top of Android’s Log class. While in development, we usually end up writing lot of log statements and before the release, we’ll cleanup the log statements by removing them manually (even though logs can be disabled in release build). This tedious process can be avoided easily by using Timber.
Timber provides lots of other options as well. Let’s see how it can be used in our projects to maintain the logs better.
1. Timber
Below are few debug statements printed using default Log class.
int a = 100; Log.e("TAG", String.format("Integer a value is: %d", a)); String name = "Android Studio"; Log.e("TAG", String.format("My name is: %s", name));
The above same statements can be printed using Timber as below.
// integer int a = 100; Timber.d("Integer a value is: %d", a); String name = "Android Studio"; Timber.d("My name is: %s", name);
- You can notice here, the TAG is not passed to Timber as it automatically detects the class in which logs were written.
- Also String formatter is not used to format the statement as Timber can do it automatically for you.
2. Integrating Timber
Now let’s see how to integrate Timber library in your project making it available in every class.
1. Create a new project in Android Studio from File ⇒ New Project and select Basic Activity from templates.
2. Open build.gradle and add Timber dependency.Butterknife is optional here, but required for this example.
// timber implementation 'com.jakewharton.timber:timber:4.7.1' // butter knife implementation 'com.jakewharton:butterknife:8.8.1' annotationProcessor 'com.jakewharton:butterknife-compiler:8.8.1'
3. Timber has to be initialized as soon as app starts. So, Application class would be best place to do that. Create new class named MyApplication.java and extend the class from Application .
- Initialize Timber in onCreate method by planting a new Tree.
- Here Timber.DebugTree() prints logs in debug mode.
- If you want to catch exceptions in release mode, you can create a different Tree and plant it in release mode. This step is completely optional but if you want to send exceptions to a different service, this is the appropriate place to do it.
package info.androidhive.timber; import android.app.Application; import info.androidhive.timber.log.ReleaseTree; import timber.log.Timber; public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); if (BuildConfig.DEBUG) { Timber.plant(new Timber.DebugTree()); } else { Timber.plant(new ReleaseTree()); } } }
4. You can create a custom tree by extending the class from Timber.Tree . Here is the example of ReleaseTree.java class.
package info.androidhive.timber.log; import android.util.Log; import timber.log.Timber; public class ReleaseTree extends Timber.Tree { @Override protected void log(int priority, String tag, String message, Throwable t) { if (priority == Log.VERBOSE || priority == Log.DEBUG) { return; } // log your crash to your favourite // Sending crash report to Firebase CrashAnalytics // FirebaseCrash.report(message); // FirebaseCrash.report(new Exception(message)); } }
4. Finally add MyApplication to your <application> tag in your AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="info.androidhive.timber"> <application android:name=".MyApplication" ...> </application> </manifest>
5. Now, Timber is ready to use in your app. Below are few examples of Timber log statements demonstrating different scenarios.
package info.androidhive.timber; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.Toolbar; import android.widget.Toast; import butterknife.ButterKnife; import butterknife.OnClick; import butterknife.Unbinder; import timber.log.Timber; public class MainActivity extends AppCompatActivity { private Unbinder unbinder; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); unbinder = ButterKnife.bind(this); Toolbar toolbar = findViewById(R.id.toolbar); setSupportActionBar(toolbar); // boolean boolean isWeekend = false; Timber.d("This prints the boolean value. Is weekend: %b", isWeekend); // integer int a = 100; Timber.d("Integer a value is: %d", a); // float float pi = 3.14159f; Timber.d("Pi value is: %f", pi); } @OnClick(R.id.btn_log_string) void logMessage() { Timber.d("Hello from Timber!"); showToast(); } @OnClick(R.id.btn_log_exception) void logException() { try { int a = 10 / 0; Timber.d("Value of a: %d", a); } catch (Exception e) { Timber.e(e); // or // Timber.e("Exception in math operation: %s", e.getMessage()); } showToast(); } private void showToast() { Toast.makeText(getApplicationContext(), "Check LogCat for message or error!", Toast.LENGTH_SHORT).show(); } @Override protected void onDestroy() { super.onDestroy(); unbinder.unbind(); } }
Hi there! I am Founder at androidhive and programming enthusiast. My skills includes Android, iOS, PHP, Ruby on Rails and lot more. If you have any idea that you would want me to develop? Let’s talk: [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK