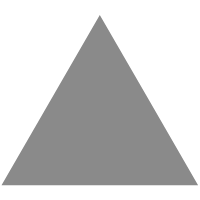
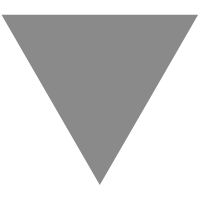
PHP Exceptions: Try Catch for Error Handling
source link: https://www.tuicool.com/articles/hit/je6zeuZ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
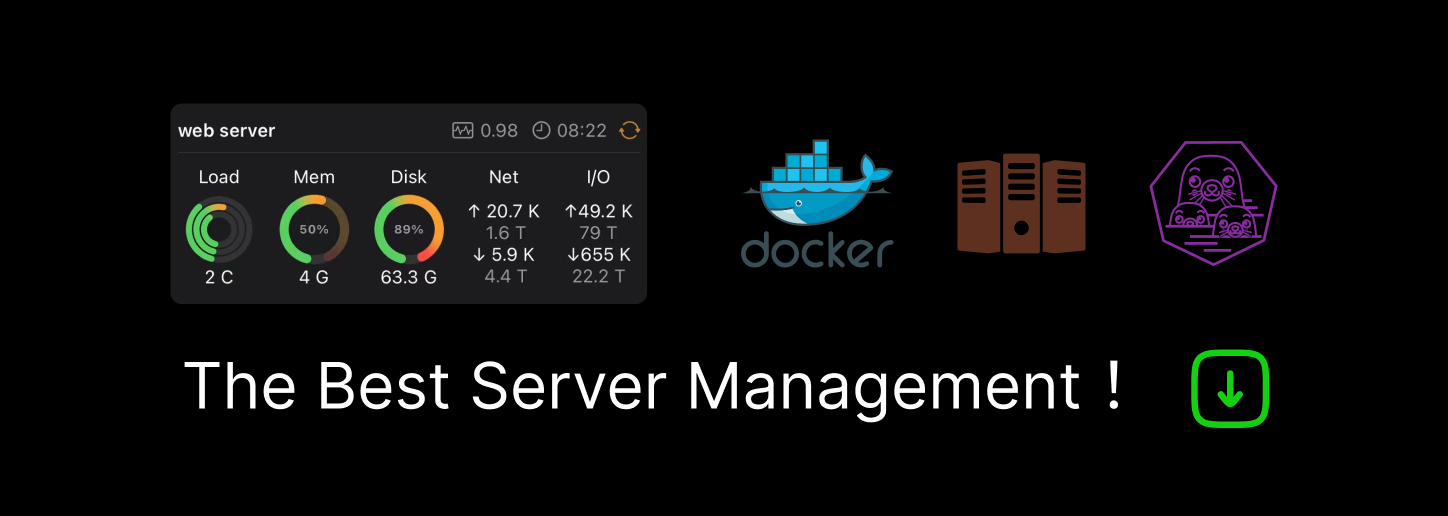
In this post, you'll learn how to use exception handling in PHP. As of PHP 5, we can use try catch blocks for error handling—this is a better way to handle exceptions and control the flow of your application. In this article, we'll go through the basics of exception handling along with a couple of real-world examples.
What is an Exception?
PHP 5 introduced a new error model which allows you to throw and catch exceptions in your application—this is a better way of handling errors than what we had in older versions of PHP. All exceptions are instances of the base class Exception
, which we can extend to introduce our own custom exceptions.
It's important to note here that exception handling is different than error handling. In error handling, we can use the set_error_handler
function to set our custom error handling function so that whenever an error is triggered, it calls our custom error handling function. In that way, you can control errors. Generally however, certain kinds of errors are unrecoverable and stop program execution.
On the other hand, exceptions are something that are thrown deliberately by the code, and it's expected that it'll be caught at some point in your application. So we can say that exceptions are recoverable as opposed to certain errors which are unrecoverable. If an exception which is thrown is caught somewhere in your application, program execution continues from the point where the exception was caught. And an exception which is not caught anywhere in your application results in an error, thus halting program execution.
Exception Handling Control Flow
Let's refer to the following diagram that shows the generic exception handling control flow.

Exceptions can be thrown and caught by using the try
and catch
blocks. You are responsible for throwing exceptions when something occurs which is not expected. Let's quickly go through the basic exception handling flow as shown in the following pseudo code.
// code before the try-catch block try { // code // if something is not as expected // throw exception using the "throw" keyword // code, it won't be executed if the above exception is thrown } catch (Exception $e) { // exception is raised and it'll be handled here // $e->getMessage() contains the error message } // code after the try-catch block, will always be executed
Most of the time, when you're dealing with exceptions, you'll end up using a pattern as shown in the above snippet. You can also use the finally
block along with the try
and catch
blocks, but we'll get back to that later in this article.
The try
block is the one to use where you suspect that your code may generate an exception. You should always wrap such code using try
and catch
.
Throwing an Exception
An exception might be thrown by a function that you call, or you can use the throw
keyword to throw an exception manually. For example, you might validate some input before performing any operation, and throw an exception if the data is not valid.
It's important to note here that if you throw an exception but you haven't defined the catch
block which is supposed to handle that exception, it'll result in the fatal error. So you need to make sure that you always define the catch
block if you're throwing exceptions in your application.
Once an exception is caught in the catch
block, the Exception
object contains the error message which was thrown using the throw
keyword. The $e
variable in the above example is an instance of the Exception
class, so it has access to all methods of that class. In this block, you should define your own exception handling logic — what exactly you want to do with the error you catch.
In the next section, we'll go through a real-world example to understand how exception handling works.
A Real-World Example
In this section, we'll build a real-world example to demonstrate exception handling in PHP.
Let's assume that you've built an application which loads application configuration from the config.php file. Now, it's essential that the config.php file must be present when your application is bootstrapped. Thus, your application can't run if the config.php file is not present. So this is the perfect use-case to throw an exception and let the user know they need to fix the issue.
<?php try { // init bootstrapping phase $config_file_path = "config.php"; if (!file_exists($config_file_path)) { throw new Exception("Configuration file not found."); } // continue execution of the bootstrapping phase } catch (Exception $e) { echo $e->getMessage(); die(); } ?>
As you can see in the above example, we're checking for the existence of the config.php file in the beginning of the bootstrapping phase. If the config.php file is found, the execution is continued normally. On the other hand, we'll throw an exception if the config.php file doesn't exist. Also, we would like to stop execution as well in case there's an exception!
So that's how you can use exceptions in your applications. You should throw exceptions for use-cases that are exceptional—you shouldn't unnecessarily throw exceptions for generic errors like invalid user credentials, improper directory permissions etc., that you expect to happen frequently. These are better handled by generic error messages in the regular application execution flow.
So that was an example of handling exceptions using the default Exception
class. In the next section, we'll see how you can extend the core Exception
class and create your own custom exceptions in your application.
How to Create Custom Exceptions
In this section, we'll discuss how you can create custom exceptions in your applications. In fact, we'll extend the example which we've just discussed in the previous section to demonstrate custom exceptions.
In the previous example, we threw the configuration exception using the default Exception
class. That's perfectly fine as long as you just want to deal with the exception error message. However, sometimes you want to do a bit more based on the type of exception which is being thrown. That's where custom exceptions are useful.
Let's revisit the previous example as shown in the following snippet.
<?php class ConfigFileNotFoundException extends Exception {} try { // init bootstrapping phase $config_file_path = "config.php"; if (!file_exists($config_file_path)) { throw new ConfigFileNotFoundException("Configuration file not found."); } // continue execution of the bootstrapping phase } catch (ConfigFileNotFoundException $e) { echo "ConfigFileNotFoundException: ".$e->getMessage(); // other additional actions that you want to carry out for this exception die(); } catch (Exception $e) { echo $e->getMessage(); die(); } ?>
Firstly, we've defined the ConfigFileNotFoundException
class which extends the default Exception
class. Now, it becomes our custom exception class and we can use it when we want to throw the ConfigFileNotFoundException
exception in our application.
Next, we've used the throw
keyword to throw the ConfigFileNotFoundException
exception in case the config.php file doesn't exists. The important difference lies in the catch
block though. As you can see, we've defined two catch
blocks, and each block is used to catch the different type of exception.
The first one catches exceptions of the ConfigFileNotFoundException
type. So, if an exception which is being thrown is of the ConfigFileNotFoundException
type, this block will be executed. If the exception type doesn't match any of the specific catch
blocks, it will match the last one which is there to catch all generic exception messages.
The Finally Block
In this section, we'll how you can use the finally
keyword along with the try
and catch
blocks. Sometimes, you want to execute a piece of code irrespective of whether an exception was thrown. That's where you can use the finally
block, since the code you place in the finally block will always be executed after execution of the try and catch blocks, irrespective of whether or not an exception has been thrown.
Let's try to understand it using the following example.
try { // code // if something is not as expected // throw exception using the "throw" keyword // code, it won't be executed if the above exception is thrown } catch (Exception $e) { // exception is raised and it'll be handled here // $e->getMessage() contains the error message } finally { // code, it'll always be executed }
The code in the above example is pretty much the same with the only exception that we've added the finally
block after the catch
block. And as we discussed, the code in this block will always be executed.
The typical use-cases that we could think of using the finally block are generally related to resource cleanup. For example, if you've opened a database connection or a file on the disk in the try
block, you can perform cleanup tasks like closing the connection in the finally
block as it's guaranteed to run.
Exception handling is a key coding skill and you should consider how exceptions will be handled while developing your applications. This will help you detect and recover from unexpected errors in your application. I hope that this post will inspire you to write better error handling code!
Conclusion
Today, we discussed the topic of exception handling in PHP. In the first half of the article, we discussed the basics of exceptions in PHP and built a real-world example to demonstrate how they work. At the end, we explored how you can create custom exceptions by extending the core Exception
class.
Recommend
-
114
Try catch ftw. Add to FavoritesShareTry catch ftw.
-
40
今天在掘金看到了一篇文章,慎用 try catch,发布者的昵称是“前端妹子”。根据我的经验,这种昵称一般都不是妹子,大概率是营销号(PS:如果能换个美女头像就更走心了)。(这个还真是个妹子,www.facebook.com/raoenhui 之前言论欠妥,在
-
40
前言 ECMA-262第3版引入了try catch语句,作为JavaScript中处理异常的一种标准方式。基本的语法如下所示。 但是在前端js代码中很少看到try catch语句,并不是所以代码都需要加try catch来作得不偿失的“保险”,下面来分析作为
-
48
Insights Gotta Catch ’Em All ByDean Hiller andSean Smith Friday, 29 March 2019 Link copied successfully ...
-
11
Why use exceptions? ¶ Δ What good can using exceptions do for me? The basic answer is: Using exceptions for er...
-
9
Error Handling with JavaScript Try CatchJavaScript Try Catch: What You Need to KnowImage from unsplash.comHave you ever made a mistake in your code that could not be...
-
11
Blog / JavaScript / Error Handling in JavaScript: A Guide to Try/Catch, Throw, and Custom Error Classes Erro...
-
4
BlogExceptions in Kotlin: Beyond the Try/Catch Raphael De Lio 15 May, 2023
-
6
This post will cover how to alter NGINX’s error page handling when using PHP 8.x “Blessed Images” on App Service Linux. Overview With PHP 8.x Blessed Images using NGINX (the current default), if the application retur...
-
8
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK