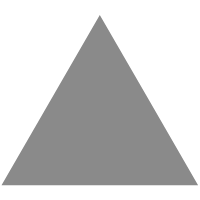
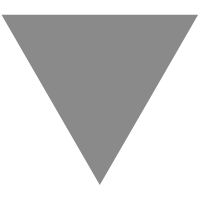
GitHub - enquirer/enquirer: Stylish, intuitive and user-friendly prompt system.
source link: https://github.com/enquirer/enquirer
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Enquirer
Stylish CLI prompts that are user-friendly, intuitive and easy to create.
>_ Prompts should be more like conversations than inquisitions▌
Enquirer is fast, easy to use, and lightweight enough for small projects, while also being powerful and customizable enough for the most advanced use cases.
- Fast - Loads in ~4ms (that's about 3-4 times faster than a single frame of a HD movie at 60fps)
- Lightweight - Only one dependency.
- Easy to use - Uses promises and async/await to make prompts easy to create and use.
- Intuitive - Navigating around input and choices is a breeze. Advanced keypress combos are available to simplify usage. You can even record and playback keypresses to aid with tutorials and videos.
- Multilingual - Easily add support for multiple languages.
- Extensible - Prompts are easy to create and extend.
- Flexible - All prompts can be used standalone or chained together.
- Pluggable - Add advanced features to Enquirer with plugins.
- Stylish - Easily override styles and symbols for any part of the prompt.
- Validation - Optionally validate user input with any prompt.
- Well tested - All prompts are well-tested, and tests are easy to create without having to use brittle, hacky solutions to spy on prompts or "inject" values.
❯ Table of Contents
- Install
- Getting started
- Usage
- Enquirer API
- Prompts
- Types
- Keypresses
- Options
- Release History
- Performance
- About
❯ Install
Install with npm:
$ npm install --save enquirer
(Requires Node.js 8.6 or higher. Please let us know if you need support for an earlier version by creating an issue.)
❯ Getting started
Get started with Enquirer, the most powerful and easy-to-use Node.js library for creating interactive CLI prompts.
❯ Usage
Single prompt
The easiest way to get started with enquirer is to pass a question object to the prompt
method.
const { prompt } = require('enquirer'); const response = await prompt({ type: 'input', name: 'username', message: 'What is your username?' }); console.log(response); //=> { username: 'jonschlinkert' }
(Examples with await
need to be run inside an async
function)
Multiple prompts
Pass an array of "question" objects to run a series of prompts.
const response = await prompt([ { type: 'input', name: 'name', message: 'What is your name?' }, { type: 'input', name: 'username', message: 'What is your username?' } ]); console.log(response); //=> { name: 'Edward Chan', username: 'edwardmchan' }
❯ Enquirer API
Enquirer
Create an instance of Enquirer
.
Params
options
{Object}: (optional) Options to use with all prompts.answers
{Object}: (optional) Answers object to initialize with.
Example
const Enquirer = require('enquirer'); const enquirer = new Enquirer();
register
Register a custom prompt type.
Params
type
{String}fn
{Function|Prompt}:Prompt
class, or a function that returns aPrompt
class.returns
{Object}: Returns the Enquirer instance
Example
const Enquirer = require('enquirer'); const enquirer = new Enquirer(); enquirer.register('customType', require('./custom-prompt'));
prompt
Prompt function that takes a "question" object or array of question objects, and returns an object with responses from the user.
Params
questions
{Array|Object}: Options objects for one or more prompts to run.returns
{Promise}: Promise that returns an "answers" object with the user's responses.
Example
const Enquirer = require('enquirer'); const enquirer = new Enquirer(); const response = await enquirer.prompt({ type: 'input', name: 'username', message: 'What is your username?' }); console.log(response);
use
Use an enquirer plugin.
Params
plugin
{Function}: Plugin function that takes an instance of Enquirer.returns
{Object}: Returns the Enquirer instance.
Example
const Enquirer = require('enquirer'); const enquirer = new Enquirer(); const plugin = enquirer => { // do stuff to enquire instance }; enquirer.use(plugin);
Enquirer#prompt
Prompt function that takes a "question" object or array of question objects, and returns an object with responses from the user.
Params
questions
{Array|Object}: Options objects for one or more prompts to run.returns
{Promise}: Promise that returns an "answers" object with the user's responses.
Example
const { prompt } = require('enquirer'); const response = await prompt({ type: 'input', name: 'username', message: 'What is your username?' }); console.log(response);
❯ Prompts
- AutoComplete
- Confirm
- Input
- Invisible
- List
- Multiselect
- Number
- Password
- Select
- Snippet
- Sort
- Survey
Text
(alias for Input)
AutoComplete Prompt
Prompt that auto-completes as the user types, and returns the selected value as a string.
Related prompts
Confirm Prompt
Prompt that returns true
or false
.
Related prompts
Input Prompt
Prompt that takes user input and returns a string.
Related prompts
Invisible Prompt
Prompt that takes user input, hides it from the terminal, and returns a string.
Related prompts
List Prompt
Prompt that returns a list of values, created by splitting the user input. The default split character is ,
with optional trailing whitespace.
Related prompts
Multiselect Prompt
Prompt that allows the user to select multiple items from a list of options.
Related prompts
Number Prompt
Prompt that takes a number as input.
Related prompts
Password Prompt
Prompt that takes user input and masks it in the terminal. Also see the invisible prompt
Related prompts
Select Prompt
Prompt that allows the user to select from a list of options.
Related prompts
Snippet Prompt
Prompt that allows the user to replace placeholders in a snippet of code or text.
Related prompts
Sort Prompt
Prompt that allows the user to sort items in a list.
Example
In this example, custom styling is applied to the returned values to make it easier to see what's happening.
Related prompts
Survey Prompt
Prompt that allows the user to provide feedback for a list of questions.
Related prompts
❯ Types
- ArrayPrompt
- BooleanPrompt
- DatePrompt (Coming Soon!)
- NumberPrompt
- StringPrompt
ArrayPrompt
todo
BooleanPrompt
todo
NumberPrompt
todo
StringPrompt
todo
❯ Keypresses
All prompts
Key combinations that may be used with all prompts.
command description ctrl+a Move the cursor to the first character in user input. ctrl+c Cancel the prompt. ctrl+g Reset the prompt to its initial state.Move cursor
Key combinations that may be used on prompts that support user input, such as the input prompt, password prompt, and invisible prompt).
command description left Move the cursor forward one character. right Move the cursor back one character. ctrl+a Move cursor to the start of the line ctrl+e Move cursor to the end of the line ctrl+b Move cursor back one character ctrl+f Move cursor forward one character ctrl+x Toggle between first and cursor positionSelect choices
These key combinations may be used on prompts that support multiple choices, such as the multiselect prompt, or the select prompt when the multiple
options is true.
options.multiple
is true.
number
Move the pointer to the choice at the given index. Also toggles the selected choice when options.multiple
is true.
a
Toggle all choices to be enabled or disabled.
i
Invert the current selection of choices.
g
Toggle the current choice group.
Hide/show choices
command description fn+up Decrease the number of visible choices by one. fn+down Increase the number of visible choices by one.Move/lock Pointer
command description number Move the pointer to the choice at the given index. Also toggles the selected choice whenoptions.multiple
is true.
up
Move the pointer up.
down
Move the pointer down.
ctrl+a
Move the pointer to the first visible choice.
ctrl+e
Move the pointer to the last visible choice.
(mac) fn+left / (win) home
Move the pointer to the first choice in the choices array.
(mac) fn+right / (win) end
Move the pointer to the last choice in the choices array.
shift+up
Scroll up one choice without changing pointer position (locks the pointer while scrolling).
shift+down
Scroll down one choice without changing pointer position (locks the pointer while scrolling).
❯ Options
Prompt options
Each prompt takes an options object (aka "question" object), that implements the following interface:
{ type: string | function, name: string | function, message: string | function | async function, initial: string | function | async function format: function | async function, result: function | async function, validate: function | async function }
type
string|function
Enquirer uses this value to determine the type of prompt to run, but it's optional when prompts are run directly.
name
string|function
Used as the key for the answer on the returned values (answers) object.
message
string|function
The message to display when the prompt is rendered in the terminal.
initial
string|function
The default value to return if the user does not supply a value.
format
function
Function to format user input in the terminal.
result
function
Function to format the final submitted value before it's returned.
validate
function
Function to validate the submitted value before it's returned. This function may return a boolean or a string. If a string is returned it will be used as the validation error message.
(type
, name
and message
are required).
Example
const question = { type: 'select', name: 'color', message: 'Favorite color?', initial: 1, choices: [ { name: 'red', message: 'Red', value: '#ff0000' }, { name: 'green', message: 'Green', value: '#00ff00' }, { name: 'blue', message: 'Blue', value: '#0000ff' } ] };
Choice objects
Choice { name: string; message: string | undefined; value: string | undefined; hint: string | undefined; disabled: boolean | string | undefined; }
name
string
The unique key to identify a choice
message
string
The message to display in the terminal
value
string
An optional value to associate with the choice. This is useful for creating key-value pairs from user choices.
hint
string
Value to display to provide user help next to a choice.
disabled
boolean|string
Disable a choice so that it cannot be selected. This value may either be true
, false
, or a message to display.
❯ Release History
Please see CHANGELOG.md.
❯ Performance
MacBook Pro, Intel Core i7, 2.5 GHz, 16 GB.
Load time
Time it takes for the module to load the first time (average of 3 runs):
enquirer: 4.013ms
inquirer: 286.717ms
prompts: 17.010ms
❯ About
ContributingPull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Running TestsRunning and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb#dev verb-generate-readme && verb
Contributors
Commits Contributor 72 jonschlinkert 12 doowb 1 mischah 1 skellockAuthor
Jon Schlinkert
Credit
Thanks to derhuerst, creator of prompt libraries such as prompt-skeleton, which influenced some of the concepts we used in our prompts.
License
Copyright © 2018, Jon Schlinkert. Released under the MIT License.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK