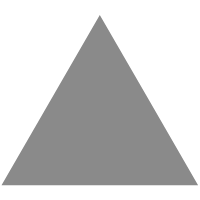
24
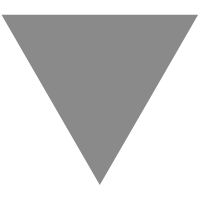
Unity联网游戏基础原理与字节数组-腾讯游戏学院
source link: http://gad.qq.com/article/detail/288006
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
如果你要制作一个能联网的游戏,无论是网络游戏还是局域网游戏都离不开网络通信,网络游戏一般是一个服务器对应多个客户端,如图



多个玩家访问一台服务器,每个玩家实际上是一个客户端,在这里我们列举两个小例子
玩家登录后需要显示玩家的角色相关数据比如装备,道具,
玩家的数据是存在服务器端数据库的,想要得到这些数据必须从服务器获得,
这个过程分为两步:
第一步:玩家向服务器发送请求数据(客户端=>服务器)
第二步:服务器收到玩家发送的请求数据信息,读取玩家信息返回数据给玩家(服务器=>客户端)
第三步:玩家收到服务器数据,显示到unity里
王者荣耀里有十个玩家,为什么一个玩家走路,其他玩家都能看到这个玩家往哪里走呢?这个过程,其实现思路就是分这几步实现的:
第一步:玩家1向服务器发送自己当前的位置数据(客户端=>服务器)
第二步:服务器收到玩家1发送的自己的当前的位置数据
第三步:服务器把玩家1的位置发送给剩余的9个玩家(服务器=>客户端)
第四步:剩余的9个玩家收到了玩家1的当前的位置数据
第五步:根据收到的玩家1的位置数据显示到unity里
我们发现网络游戏数据走向一般分为两种情况

1(客户端=>服务器)数据从客户端发送到服务器 这种情况叫请求
2(服务器=>客户端)数据从服务器返回到客户端 这种情况叫响应
所以,网络游戏开发中,玩家玩游戏时,大部分情况都是一个请求对应一个响应来进行的,
比如玩家登录发送登录请求 数据包含玩家账号密码 服务器处理后下发登录响应
玩家购买道具发送购买请求 服务器处理后返回购买后获得的道具和消耗的钱币
玩家更换阵容发送更换阵容请求 服务器处理后返回更换阵容成功失败
玩家穿上装备发送穿装备请求 服务器处理后返回装备更换
等等等等
了解了这个客户端与服务器一个请求对应一个响应交互的过程后,下一步我们需要关心的是数据如何发送,发送什么样的数据。
网络通信中的数据是以字节数组的形式进行传输,我们需要把代码中的int,string等类型转换成字节数组发送出去,字节数组听起来很抽象,那么实际上字节数组是什么东西呢,以一个int为例,来看看字节数组和int之间到底有什么关系
新建一个c#控制台程序,写一段测试代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace LeaderVSServer
{
class Program
{
static void Main(string[] args)
{
int num1 = 1;
byte[] numbyte1 = BitConverter.GetBytes(num1);//将int类型的变量转换成byte数组
Console.Write("int:"+ num1 + "= numbyte:");
PrintByteAry(numbyte1);//打印byte数组
int num2 = 10;
byte[] numbyte2 = BitConverter.GetBytes(num2);
Console.Write("int:" + num2 + "= numbyte:");
PrintByteAry(numbyte2);
int num3 = 255;
byte[] numbyte3 = BitConverter.GetBytes(num3);
Console.Write("int:" + num3 + "= numbyte:");
PrintByteAry(numbyte3);
int num4 = 258;
byte[] numbyte4 = BitConverter.GetBytes(num4);
Console.Write("int:" + num4 + "= numbyte:");
PrintByteAry(numbyte4);
int num5 = 2560;
byte[] numbyte5 = BitConverter.GetBytes(num5);
Console.Write("int:" + num5 + "= numbyte:");
PrintByteAry(numbyte5);
int num6 = 256*256;
byte[] numbyte6 = BitConverter.GetBytes(num6);
Console.Write("int:256*256" + "= numbyte:");
PrintByteAry(numbyte6);
int num7 = 256 * 256 * 256;
byte[] numbyte7 = BitConverter.GetBytes(num7);
Console.Write("int:256 * 256 * 256" + "= numbyte:");
PrintByteAry(numbyte7);
int num8 = 256 * 256 * 256 + 3;
byte[] numbyte8 = BitConverter.GetBytes(num8);
Console.Write("int:256 * 256 * 256 + 3" + "= numbyte:");
PrintByteAry(numbyte8);
Console.ReadKey();
}
public static void PrintByteAry(byte[] numbyte)
{
foreach (byte ubyte in numbyte)
{
Console.Write(ubyte.ToString() + " ");
}
Console.WriteLine("");
}
}
}
运行起来后,控制台打印

接下来我们来找一下规律
1对应的字节数组是 1,0,0,0
10对应的字节数组是10,0,0,0
255对应的字节数组是255,0,0,0
258对应的字节数组是2,1,0,0
2560对应的字节数组是0,10,0,0
256*256对应的字节数组是0,0,1,0
256*256*256对应的字节数组是0,0,0,1
256*256*256 + 3对应的字节数组是3,0,0,1
由此我们可以看到每个int类型的变量转换成byte数组里面共有4个元素
第一个位置代表2的0次方
第二个位置表示2的8次方
第三个位置表示2的16次方
第四个位置表示2的24次方
如果我们发送两个int类型数据,1和10,它转换的byte数组是这样的1,0,0,0,10,0,0,0
这就是一个int类型转换成byte数组占4个字节的原因啦,同理我们可以研究下其他类型的转换,我在这里就不一一细说了,大家自行编写测试代码进行打印查看即可。
好了,到了这里应该已经明白了在网络传输的数据是什么样的了,知道了网络传输数据结构将会为你以后实现网络通信编码打下一个基础,以后进行网络编程时就不会慌了。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK